Write a C++ program following the instructions below. Please follow the order of the tasks given below, and write one function a time, test it, and then move on to the next function. 1. Implement Euclidean Algorithm for calculating greatest common divisor First write and test a function that calculates the greatest common divisor of two non- negative integers, as follows: /* precondition: a>=b>=0 */ /* postcondition: return d=gcd(a,b) */ int EuclidAlgGCD (int a, int b); Then implement the extended Euclidean Algorithm to find not only the greatest common divisor of two integers, but also find the integer coefficients that expresses the gcd in terms of the integers, as illustrated below: /* precondition: a>=b>=0 */ /* postcondition: return d=gcd(a,b), s and t are set so that d=sa+tb */ int ExtendedEuclidAlgGCD (int a, int b, int & s, int & t); 2. Modular arithmetic Write a function that returns the result of any integer modulo of n (i.e., reduce the integer modulo n). Note that the C++ operator % can be used, but it returns a negative value, for example, -1 % 3 yields -1, so we need to do something adjustment (recall that -1 mod 3 = 2 because -1= (-1)×3+2). Therefore, we will use the mod() function from this step to implement the later encode() and decode() functions. /* precondition: n is greater than 1,a can be negative postcondition: return a mod n (as defined in class) a mod n = r if and only if a = nk+r, 0 =< r < n (note that r needs to be non-negative). */ int mod (int a, int n); 3. Find Relatively Prime Write a function to return a number that is relatively prime with the given number. /* return an integer that is relatively prime with n, and greater than 1 i.e., the gcd of the returned int and n is 1 Note: Although gcd(n,n-1)=1, we don't want to return n-1*/ int RelativelyPrime (int n) 4. Find Inverse Then implement the following function, which returns the inverse modulo, and test it. Note that you need to check whether a and n are relative prime before calling this function. Recall that a and n are relatively prime means that gcd(a,n)=1, i.e., their greatest common divisor is 1. Also recall that the extended Euclidean Algorithm can find the integer s and t for a and n such that as+nt=gcd(a,n), where s is the inverse of a modulo n. /* n>1, a is nonnegative */ /* a<=n */ /* a and n are relative prime to each other */ /* return s such that a*s mod n is 1 */ int inverse (int a, int n) { int s, t; int d = ExtednedEuclidAlgGCD (n, a, s, t); if (d==1) { return (mod (t, n)); // t might be negative, use mod() to reduce to // an integer between 0 and n-1 } else { cout <<"a and n are not relatively prime!\n"; } } 5. Practice with RSA algorithm 1. Picktwoprimenumbersp,q,forexample, const int P=23; const int Q=17; int PQ=P*Q; Find a e that is relatively prime with (p-1)(q-1) Call RelativelyPrime () Calculatetheinversemodulo(p-1)(q-1)ofetobeyourd Use inverse () WriteafunctionEncodeasfollows: // Return M^e mod PQ int Encode (int M, int e, int PQ); 5. WriteafunctionDecodeasfollows: //Return C^d mod PQ int Decode (int C, int d, int PQ); 6. Verify that RSA algorithm works, i.e., intM; /* M is an integer that is smaller than PQ */ cout <<"Enter an integer that is smaller than"<< PQ; cin <
Write a C++ program following the instructions below. Please follow the order of the tasks given below, and write one function a time, test it, and then move on to the next function.
1. Implement Euclidean
First write and test a function that calculates the greatest common divisor of two non- negative integers, as follows:
/* precondition: a>=b>=0 */
/* postcondition: return d=gcd(a,b) */ int EuclidAlgGCD (int a, int b);
Then implement the extended Euclidean Algorithm to find not only the greatest common divisor of two integers, but also find the integer coefficients that expresses the gcd in terms of the integers, as illustrated below:
/* precondition: a>=b>=0 */
/* postcondition: return d=gcd(a,b), s and t are set so that d=sa+tb */ int ExtendedEuclidAlgGCD (int a, int b, int & s, int & t);
2. Modular arithmetic
Write a function that returns the result of any integer modulo of n (i.e., reduce the integer modulo n). Note that the C++ operator % can be used, but it returns a negative value, for example, -1 % 3 yields -1, so we need to do something adjustment (recall that -1 mod 3 = 2 because -1= (-1)×3+2). Therefore, we will use the mod() function from this step to implement the later encode() and decode() functions.
/* precondition: n is greater than 1,a can be negative postcondition: return a mod n (as defined in class)
a mod n = r if and only if a = nk+r, 0 =< r < n (note that r needs to be non-negative).
*/ int mod (int a, int n);3. Find Relatively Prime
Write a function to return a number that is relatively prime with the given number.
/* return an integer that is relatively prime with n, and greater than 1 i.e., the gcd of the returned int and n is 1
Note: Although gcd(n,n-1)=1, we don't want to return n-1*/
4. Find Inverse
Then implement the following function, which returns the inverse modulo, and test it. Note that you need to check whether a and n are relative prime before calling this function. Recall that a and n are relatively prime means that gcd(a,n)=1, i.e., their greatest common divisor is 1. Also recall that the extended Euclidean Algorithm can find the integer s and t for a and n such that as+nt=gcd(a,n), where s is the inverse of a modulo n.
/* n>1, a is nonnegative */
/* a<=n */
/* a and n are relative prime to each other */ /* return s such that a*s mod n is 1 */
int inverse (int a, int n)
{
int s, t;
int d = ExtednedEuclidAlgGCD (n, a, s, t);
if (d==1) {
return (mod (t, n)); // t might be negative, use mod() to reduce to // an integer between 0 and n-1
} else {
cout <<"a and n are not relatively prime!\n"; }}
5. Practice with RSA algorithm
1. Picktwoprimenumbersp,q,forexample,
-
const int P=23;
-
const int Q=17;
-
int PQ=P*Q;
-
Find a e that is relatively prime with (p-1)(q-1) Call RelativelyPrime ()
-
Calculatetheinversemodulo(p-1)(q-1)ofetobeyourd Use inverse ()
-
WriteafunctionEncodeasfollows:
-
// Return M^e mod PQ
-
int Encode (int M, int e, int PQ);
5. WriteafunctionDecodeasfollows:
-
//Return C^d mod PQ
-
int Decode (int C, int d, int PQ);
6. Verify that RSA algorithm works, i.e.,
-
intM;
-
/* M is an integer that is smaller than PQ */
-
cout <<"Enter an integer that is smaller than"<< PQ;
-
cin <<M; •
-
C=Encode (M, e, PQ);
-
M1=Decode (C, d, PQ);
-
assert (M==M1); //Note: include assert.h header file to use this //macro/function.

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 8 images

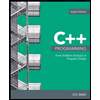
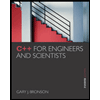
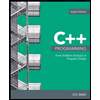
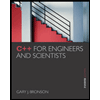