What is #1 called, overloading or overriding?
Download the following two java files. Create a class "Rectangle" and an interface "RegularPolygon" in your eclipse environment. Copy the code from the Java files to your class/interface.
/** Rectangle class, phase 5 */ public class Rectangle { private double length; private double width; /** Default Constructor */ public Rectangle() { // length = 1; // width = 1; } /** Constructor @param len The length of the rectangle. @param w The width of the rectangle. */ public Rectangle(double len, double w) { length = len; width = w; } /** The setLength method stores a value in the length field. @param len The value to store in length. */ public void setLength(double len) { length = len; } /** The setWidth method stores a value in the width field. @param w The value to store in width. */ public void setWidth(double w) { width = w; } /** The getLength method returns a Rectangle object's length. @return The value in the length field. */ public double getLength() { return length; } /** The getWidth method returns a Rectangle object's width. @return The value in the width field. */ public double getWidth() { return width; } /** The getArea method returns a Rectangle object's area. @return The product of length times width. */ public double getArea() { return length * width; } }
ublic interface RegularPolygon { /** * * @return the perimeter of the regular polygon (n * length) */ double getPerimeter(); }
Create a class Square, which is a special case of Rectangle, where the length equals to width. The class Square should inherit from class Rectangle and:
1. Have a constructor that only takes one double parameter. Apply this parameter to both length and width. Note: You can either use a super class constructor or use setLength() and setWidth() methods.
2. Both setLength() and setWidth() method should change both length and width to the same value.
Additional question:
1. What is #1 called, overloading or overriding?
2. What is #2 called, overloading or overriding?
3. Do you need to override getArea() method?

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

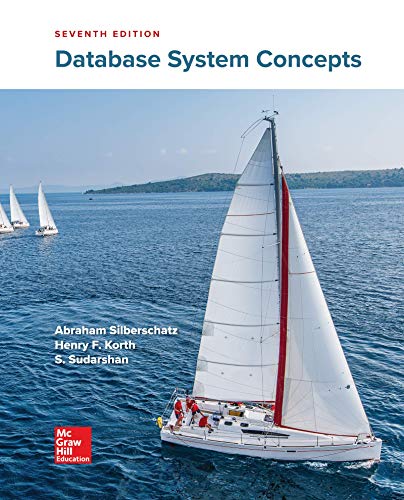
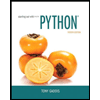
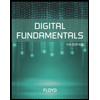
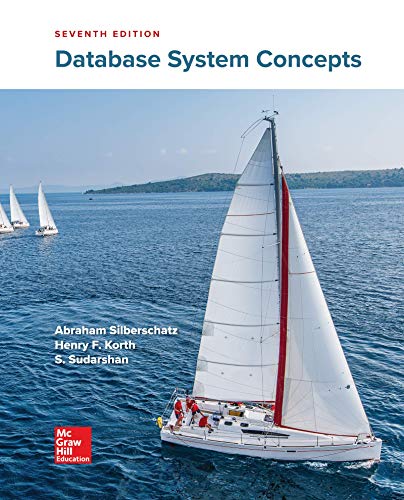
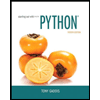
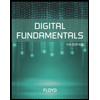
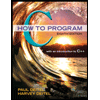
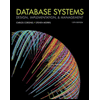
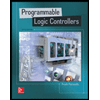