What am i doing wrong. In c /* ---------------------------------------------------------------------- Rock, Paper, Scissors Game v.1 CSC101 v.1 declares variables, prompts user for input, displays results. ------------------------------------------------------------------------ */ #include #include #include int main() { // declare variables int computer; int player; int game; int results; int theGame(int thePlayer, int theComputer); void theResults(int theGame); //the game theGame(player, computer); theResults; return 0; } /*------------------- FUNCTION: game PURPOSE: deterime win, loss, draw PARAMETER: char player, char computer RETURNS: int -------------------*/ int theGame(int thePlayer, int theComputer) { srand(time(NULL)); int randomNumber = rand()%3+1; theComputer = randomNumber; // 0 = player wins, 1 = computer wins, 2 = tie if (theComputer == 1) theComputer = 1; else if (theComputer == 2) theComputer = 2; else theComputer = 3; //intro to game printf("\n\tThe game is Rock, Paper, Scissor."); printf("\n\tRock = 1, Paper = 2, Scissor = 3."); // prompt for input printf("\n\n\tPlease enter your selection: "); scanf("%c", &thePlayer); // Player and computers choices printf("\n\t The players choice: %c", thePlayer); printf("\n\t The computers choice: %i", randomNumber); if(thePlayer == theComputer) return 2; if(thePlayer == 1 && theComputer == 2) return 1; if(thePlayer == 1 && theComputer == 3) return 0; if(thePlayer == 2 && theComputer == 3) return 1; if(thePlayer == 2 && theComputer == 1) return 0; if(thePlayer == 3 && theComputer == 1) return 1; if(thePlayer == 3 && theComputer == 2) return 0; } /*------------------- FUNCTION: shows results PURPOSE: print win, loss, draw PARAMETER: game RETURNS: none -------------------*/ void theResults(int theGame) { if(theResults == '2') { printf("The games a draw!"); } else if(theResults == '1') { printf("The Computer won!!"); } else { printf("Hooray you won!"); } return; }
What am i doing wrong.
In c
/* ----------------------------------------------------------------------
Rock, Paper, Scissors Game v.1
CSC101
v.1 declares variables, prompts user for input, displays results.
------------------------------------------------------------------------ */
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
int main()
{
// declare variables
int computer;
int player;
int game;
int results;
int theGame(int thePlayer, int theComputer);
void theResults(int theGame);
//the game
theGame(player, computer);
theResults;
return 0;
}
/*-------------------
FUNCTION: game
PURPOSE: deterime win, loss, draw
PARAMETER: char player, char computer
RETURNS: int
-------------------*/
int theGame(int thePlayer, int theComputer)
{
srand(time(NULL));
int randomNumber = rand()%3+1;
theComputer = randomNumber;
// 0 = player wins, 1 = computer wins, 2 = tie
if (theComputer == 1)
theComputer = 1;
else if (theComputer == 2)
theComputer = 2;
else
theComputer = 3;
//intro to game
printf("\n\tThe game is Rock, Paper, Scissor.");
printf("\n\tRock = 1, Paper = 2, Scissor = 3.");
// prompt for input
printf("\n\n\tPlease enter your selection: ");
scanf("%c", &thePlayer);
// Player and computers choices
printf("\n\t The players choice: %c", thePlayer);
printf("\n\t The computers choice: %i", randomNumber);
if(thePlayer == theComputer)
return 2;
if(thePlayer == 1 && theComputer == 2)
return 1;
if(thePlayer == 1 && theComputer == 3)
return 0;
if(thePlayer == 2 && theComputer == 3)
return 1;
if(thePlayer == 2 && theComputer == 1)
return 0;
if(thePlayer == 3 && theComputer == 1)
return 1;
if(thePlayer == 3 && theComputer == 2)
return 0;
}
/*-------------------
FUNCTION: shows results
PURPOSE: print win, loss, draw
PARAMETER: game
RETURNS: none
-------------------*/
void theResults(int theGame)
{
if(theResults == '2')
{
printf("The games a draw!");
}
else if(theResults == '1')
{
printf("The Computer won!!");
}
else
{
printf("Hooray you won!");
}
return;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

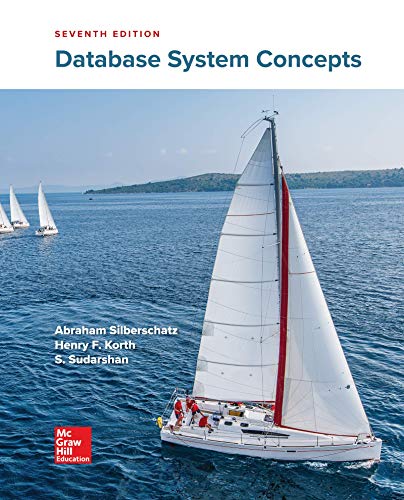
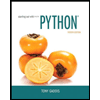
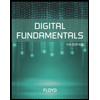
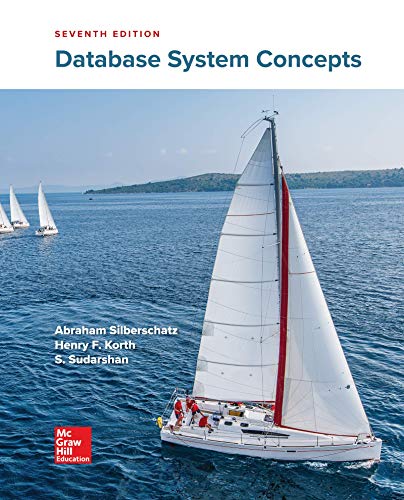
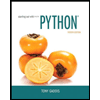
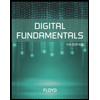
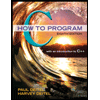
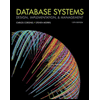
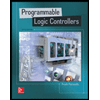