We define an m-section to be a sequence of code that can be run concurrently by maximum m threads. There are n threads in a process. Each thread executes a thread function doWork() that calls doCriticalWork() in an infinite loop. Function doCriticalWork() requires that at most m threads run it concurrently. The enter) and leave() functions are used to limit the number of threads within the m- section to a maximum of m and are the only functions that deal with synchronization. The pseudo-code algorithm for the thread function is this: void doWork(.) { while (true) { enter.): I/ limit access to m threads Il execute m-section doCriticalWork(.); Il run by max. m threads leave(.): I/ leave m-section Il do more work Function enter() returns immediately only if there are less than m threads in the m-section. Otherwise, the calling thread will be blocked. A thread calls leave() to indicate it has finished the m-section. If there was another thread blocked (in enter(0) waiting to enter the m-section, that thread will now be resumed and allowed to continue, since there are now less than m threads remaining in the m-section. a) Write a C program called msection-sem.c using the pthread library that implements the algorithm above. For the enter) and leave() functions use only one shared semaphore for synchronization. This is actually very easy if you know how a counting semaphore works. Write the enter() and leave() functions so that they are reusable, i.e. not depending on global variables. Declare any necessary shared and global variables as needed and also specify the parameters for the three functions. Declare M as a global integer variable and hard-code its value to 3. The doCriticalWork) function within the m-section must print the current thread id and the number of threads currently in the m- section to the terminal using printf(). The main() function should create and start N=10 threads that call doWork().
We define an m-section to be a sequence of code that can be run concurrently by maximum m threads. There are n threads in a process. Each thread executes a thread function doWork() that calls doCriticalWork() in an infinite loop. Function doCriticalWork() requires that at most m threads run it concurrently. The enter) and leave() functions are used to limit the number of threads within the m- section to a maximum of m and are the only functions that deal with synchronization. The pseudo-code algorithm for the thread function is this: void doWork(.) { while (true) { enter.): I/ limit access to m threads Il execute m-section doCriticalWork(.); Il run by max. m threads leave(.): I/ leave m-section Il do more work Function enter() returns immediately only if there are less than m threads in the m-section. Otherwise, the calling thread will be blocked. A thread calls leave() to indicate it has finished the m-section. If there was another thread blocked (in enter(0) waiting to enter the m-section, that thread will now be resumed and allowed to continue, since there are now less than m threads remaining in the m-section. a) Write a C program called msection-sem.c using the pthread library that implements the algorithm above. For the enter) and leave() functions use only one shared semaphore for synchronization. This is actually very easy if you know how a counting semaphore works. Write the enter() and leave() functions so that they are reusable, i.e. not depending on global variables. Declare any necessary shared and global variables as needed and also specify the parameters for the three functions. Declare M as a global integer variable and hard-code its value to 3. The doCriticalWork) function within the m-section must print the current thread id and the number of threads currently in the m- section to the terminal using printf(). The main() function should create and start N=10 threads that call doWork().
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:We define an m-section to be a sequence of code that can be run concurrently by maximum m threads.
There are n threads in a process. Each thread executes a thread function doWork() that calls
doCriticalWork() in an infinite loop. Function doCriticalWork() requires that at most m threads run it
concurrently. The enter() and leave() functions are used to limit the number of threads within the m-
section to a maximum of m and are the only functions that deal with synchronization. The pseudo-code
algorithm for the thread function is this:
void doWork(...) {
while (true) {
enter(...);
I/ limit access to m threads
Il execute m-section
doCriticalWork(.); Il run by max. m threads
leave(.);
I/ leave m-section
Il do more work
Function enter() returns immediately only if there are less than m threads in the m-section. Otherwise,
the calling thread will be blocked. A thread calls leave() to indicate it has finished the m-section. If
there was another thread blocked (in enter() waiting to enter the m-section, that thread will now be
resumed and allowed to continue, since there are now less than m threads remaining in the m-section,
a) Write a C program called msection-sem.c using the pthread library that implements the algorithm
above. For the enter() and leave() functions use only one shared semaphore for synchronization. This is
actually very easy if you know how a counting semaphore works. Write the enter() and leave()
functions so that they are reusable, i.e. not depending on global variables. Declare any necessary shared
and global variables as needed and also specify the parameters for the three functions.
Declare M as a global integer variable and hard-code its value to 3. The doCriticalWork) function
within the m-section must print the current thread id and the number of threads currently in the m-
section to the terminal using printf(). The main() function should create and start N=10 threads that call
doWork().
Use the pthread library and the POSIX semaphore API from the semaphore.h header file.
Include a screenshot with the program running.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
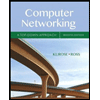
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
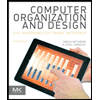
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
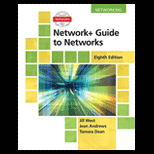
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
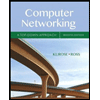
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
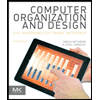
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
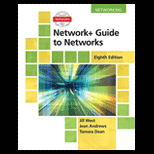
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
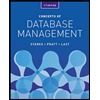
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
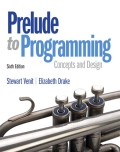
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
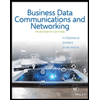
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY