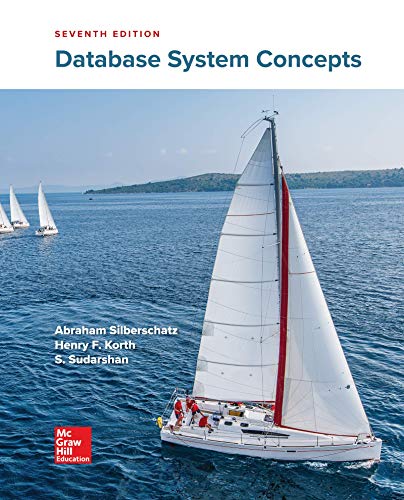
Lab 8.1 - Using Value and Reference Parameters
-
In Visual Studio Code under labactivity8_1 folder, create a new file main.cpp. Open the C++ source file main.cpp in the text editor and copy the source code below.
/** * @file WRITE FILE NAME * @author WRITE STUDENT NAME(S) * @brief Using value and reference parameters. Thisprogram * uses a function to swap the values in two variables. * @date WRITE DATE TODAY * */ #include <iostream> using namespace std; // Function prototype void swapNums(int number1, int number2); int main() { int num1 = 5, num2 = 7; // Print the two variable values cout << "In main the two numbers are " << num1 << " and " << num2 << endl; // Call a function to swap the values stored // in the two variables swapNums(num1, num2); // Print the same two variable values again cout << "Back in main again the two numbers are " << num1 << " and " << num2 << endl; return 0; } /** * @brief WRITE DESCRIPTION OF THE FUNCTION * * @param number1 DESCRIPTION * @param number2 DESCRIPTION */ void swapNums(int number1, int number2) { // Parameter a receives num1 and parameter b receives num2 // Swap the values that came into parameters a and b int temp = number1; number1 = number2; number2 = temp; // Print the swapped values cout << "In swapNums, after swapping, the two numbers are " << number1 << " and " << number2 << endl; } -
Update the header comment with the correct information.
-
Read the source code, paying special attention to the swapNums parameters. When the program is run do you think it will correctly swap the two numbers? Compile and run the program to find out.
Explain what happened. It swapped the numbers in the function but did not return any values so the numbers won’t get swapped in main.
-
Change the two swapNums parameters to be reference variables. Section 6.13 of your text shows how to do this. You will need to make the change on both the function header and the function prototype. Nothing will need to change in the function call. After making this change, recompile and rerun the program. If you have done this correctly, you should get the following output.
In main the two numbers are 5 and 7 In swapNums, after swapping, the two numbers are 7 and 5 Back in main again the two numbers are 7 and 5Explain what happened this time. The function was passed references to the variables in main. This allows the function to change the contents of the variables in main as it is working on the same variable address in memory.
-
Update the function header comments of swapNums.
-
Commit your code with a message "lab 8.1 completed". Recall the terminal commands:
-
- git add -A
- git commit -m "COMMIT MESSAGE"
-
- Push your code back to the GitHub repo then continue with Lab 8.2.
Lab 8.2 - Three File Structure 1
-
We will keep working with Lab 8.1 program. Create a new file called functions.h. Inside this file, you will have to write the guard code, to ensure the code inside this file is only included once. The guard code is demonstrated below. Copy the code below and paste it inside functions.h.
/** * @file functions.h * @author WRITE STUDENT NAME(S) * @brief Declaration file * @date WRITE DATE TODAY * */ #ifndef FUNCTIONS_H // GUARD CODE #define FUNCTIONS_H // GUARD CODE // INCLUDE THE HEADER FILES NEEDED FOR THE FUNCTIONS TO WORK using namespace std; // PUT FUNCTION PROTOTYPES HERE #endif // GUARD CODE -
Update the header comment with the correct information.
-
From main.cpp, copy and remove the function prototypes, then paste the function prototypes in functions.h.
-
Back in main.cpp, just above using namespace std, you now need to include functions.h. Observe the code below.
#include <iostream> #include "functions.h" using namespace std; -
Create another new file called functions.cpp. This file is where the function definitions are going to be placed. Note, that the first line of code in this file is to include functions.h.
/** * @file functions.cpp * @author WRITE STUDENT NAME(S) * @brief Implementation/Definition file * @date WRITE DATE TODAY * */ #include "functions.h" // PUT FUNCTION DEFINTIONS HERE -
Update the header comment with the correct information.
-
From main.cpp, copy and remove the function definitions, then paste the function definitions in functions.cpp. Observe that you are using cout inside the function, which means you need to include iostream. The right place to include iostream will be inside functions.h.
-
Compile the program. For the executable named Lab8_2 see command below
- Use the compile command g++ -Wall lab8_1.cpp functions.cpp -o Lab8_2
-
Run the program. The program should give you the same result as in Lab 8.1. Understand how this code organization could help in the maintainability of your code. You will see the importance of this type of code organization when you have lots of functions in your C++ project.
-
Commit your code with the message "lab 8.2 completed".

Step by stepSolved in 3 steps

- for c++ need 3 files please all three files (character.h, castle.h, 81.cpp) you have been asked by a computer gaming company to create a role-playing game, commonly known as an rpg. the theme of this game will be of the user trying to get a treasure that is being guarded the user will choose a character from a list you create create a character class, in a file named character.h, which will: have the following variables: name race (chosen from a list that you create, like knight, wizard, elf, etc.) weapon (chosen from a list that you create) spells (true meaning has the power to cast spells on others) anything else you want to add the treasure will be hidden in one of the rooms in a castle create a castle class, in a file named castle.h, that will: have these variables: at least four rooms named room1, room2, etc. moat (which is a lagoon surrounding a castle) which boolean (not all castles have them) anything else you want to add create a default constructor for each class that…arrow_forwardIn C++ PLEASE Use the text file from Chapter 12, forChap12.txt. SEE BELOW Write a program that opens a specified text file then displays a list of all the unique words found in the file. Addition to the text book specifications, print the total of unique words in the file. Text File = forChap12.txt No one is unaware of the name of that famous English shipowner, Cunard. In 1840 this shrewd industrialist founded a postal service between Liverpool and Halifax, featuring three wooden ships with 400-horsepower paddle wheels and a burden of 1,162 metric tons. Eight years later, the company's assets were increased by four 650-horsepower ships at 1,820 metric tons, and in two more years, by two other vessels of still greater power and tonnage. In 1853 the Cunard Co., whose mail-carrying charter had just been renewed, successively added to its assets the Arabia, the Persia, the China, the Scotia, the Java, and the Russia, all ships of top speed and, after the Great Eastern, the biggest ever…arrow_forwardPlease help with my C++Specifications • For the view and delete commands, display an error message if the user enters an invalid contact number. • Define a structure to store the data for each contact. • When you start the program, it should read the contacts from the tab-delimited text file and store them in a vector of contact objects. •When reading data from the text file, you can read all text up to the next tab by adding a tab character ('\t') as the third argument of the getline() function. •When you add or delete a contact, the change should be saved to the text file immediately. That way, no changes are lost, even if the program crashes laterarrow_forward
- Design a program that reads a file called sales.txt containing the following data: Jane, 215.5,445.5,910.0 John, 825.0,250.5,675.0 Bill,0.0,999.15 Each line has a employee's name and their weekly sales totals all separated by commas. Some employees have taken vacation some weeks so no numbers are captured for those weeks. Create a function that takes a list of floats and returns the average value for those numbers. After reading the information from the file, use a for loop to calculate the sales average using your new average function for each employee and display the following output to the screen. Jane, average sales: 523.67 John, average sales: 583.50 Bill, average sales: 499.56arrow_forwardAdd a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forwardJava : Create a .txt file with 3 rows of various movies data of your choice in the following table format: Movie ID number of viewers rating release year Movie name 0000012211 174 8.4 2017 "Star Wars: The Last Jedi" 0000122110 369 7.9 2017 "Thor: Ragnarok" Create a class Movie which instantiates variables corresponds to the table columns. Implement getters, setters, constructors and toString. Implement 2 readData() methods : the first one will return an array of Movies and the second one will return a List of movies . Test your methods.arrow_forward
- I need this done in C++ please! This program implements the top-level menu option 'R' of the Airport Application and constitutes Part 2 of the Airport Application Project requirement. When the user selects 'R' in the airport application, she will be taken to the "page" that is displayed by this program. This program is meant to replace the flight status report program (Programming Assignment 3) that did not use arrays to store the flight status information. It also reads the information from the file token wise.arrow_forwardNEED HELP DEBUGGING THE FOLLOW JS CODE JS CODE /* JavaScript 7th Edition Chapter 3 Project 03-05 Application to generate a horizontal bar chart Author: Date: Filename: project03-05.js*/ // Array of phone models sold by the companylet phones = ("Photon 6E", "Photon 6X", "Photon 7E", "Photon 7X", "Photon 8P"); // Units sold in the previous quarterlet sales = (10281, 12255, 25718, 21403, 16142); // Variable to calculate total saleslet totalSales = 0; // Use the forEach() method to sum the sales for each phone model and add it to the totalSales variablesales.forEach(addtoTotal); // For loop to generate bar chart of phone salesfor (let i = 1; i <= phones.length; i++) { let barChart = ""; // Variable to store HTML code for table cells used to create bar chart // Calculate the percent of total sales for a particular phone model let barPercent = 100*sales/totalSales; let cellTag; // Variable that will define the class of td…arrow_forwardPlease help me with these question. I am having trouble understanding what to do. Please use HTML, CSS, and JavaScript Thank youarrow_forward
- C++ I cannot run the code. Please help me fix it. Below is the requirement of the code. Design and write a C++ class that reads text, binary and csv files. The class functions: Size: Returns the file sizeName: Returns the file nameRaw: Returns the unparsed raw data (use vector here to get the unparsed raw data)Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse. With binary files, need to use RegEx to do the parse(). [My code doesn't have this part] Please refer to the Callback.cpp example on how to setup a Call-Back. CallBack.cpp: #include <string>#include <functional>#include <iostream>#include <vector>using namespace std; string ToLower(string s){ string temp; for (char c : s)…arrow_forwardAdd comments for assignment p05Thanks! Run it with C, Linuxarrow_forwardPlease answer in C++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
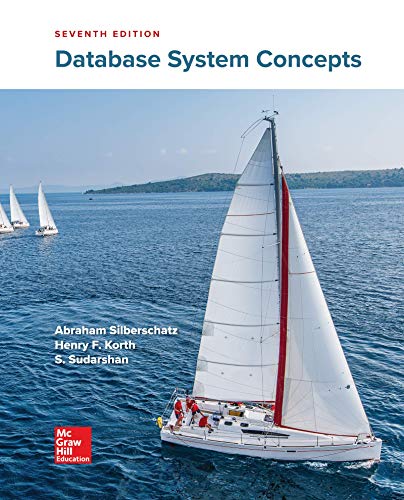
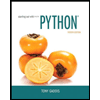
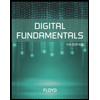
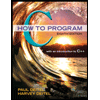
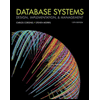
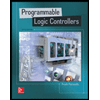