Using Python, write an application that will calculate a loan payment. To complete this, you are required to write 2 functions, LoanPayment and InterestOnlyLoanPayment, as well as a test application that calls each of the functions to get the payment amount based on parameters supplied to the functions. The test application should output both the loan payment and the interest-only loan payment based on input values from the user. The LoanPayment function is defined as follows: Payment = Loan amount / Discount factor The discount factor is {[(1 + i)^n] - 1} / [i(1 + i)^n], where you have the following: n = Payments per year × Number of years i = Annual interest rate / Payments per year Take for example the following: D = {[(1 + 0.005)^360] – 1} / [0.005(1 + 0.005)^360] = 166.7916, where n = 12 × 30 and i = 0.06 / 12. Loan payment = $250,000 / 166.7916 = $1,498.88 The InterestOnlyLoanPayment is defined as follows: Interest-only payment = Loan amount × (Annual interest rate / 12) Take for example the following: Interest-only payment = $250,000 × (0.06 / 12) = $1,250 The test application interaction should be something like the following: Enter loan amount: $250,000 Enter duration of loan: 30 Enter interest rate: 6 Interest only payment: $1,250 Principle or interest payment: $1,498.88
Using Python, write an application that will calculate a loan payment. To complete this, you are required to write 2 functions, LoanPayment and InterestOnlyLoanPayment, as well as a test application that calls each of the functions to get the payment amount based on parameters supplied to the functions. The test application should output both the loan payment and the interest-only loan payment based on input values from the user.
The LoanPayment function is defined as follows:
Payment = Loan amount / Discount factor
The discount factor is {[(1 + i)^n] - 1} / [i(1 + i)^n], where you have the following:
- n = Payments per year × Number of years
- i = Annual interest rate / Payments per year
Take for example the following:
D = {[(1 + 0.005)^360] – 1} / [0.005(1 + 0.005)^360] = 166.7916, where n = 12 × 30 and i = 0.06 / 12.
Loan payment = $250,000 / 166.7916 = $1,498.88
The InterestOnlyLoanPayment is defined as follows:
Interest-only payment = Loan amount × (Annual interest rate / 12)
Take for example the following:
Interest-only payment = $250,000 × (0.06 / 12) = $1,250
The test application interaction should be something like the following:
- Enter loan amount: $250,000
- Enter duration of loan: 30
- Enter interest rate: 6
- Interest only payment: $1,250
- Principle or interest payment: $1,498.88

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

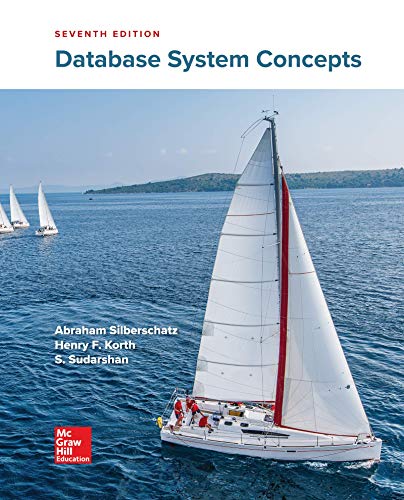
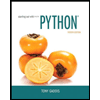
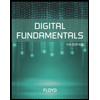
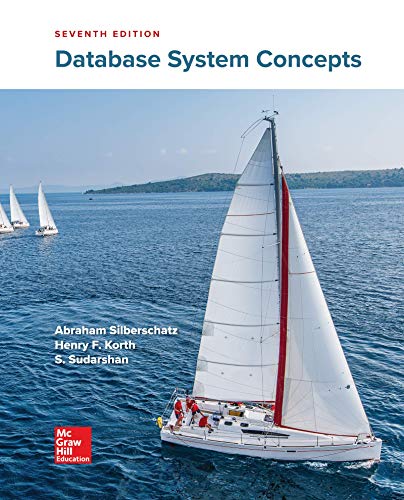
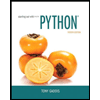
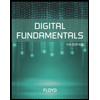
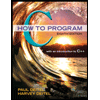
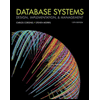
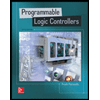