USE TEMPLATE PROVIDED AT END OF QUESTION WHILE MAKING SOLUTION ----------------------------------- Write a program to implement Hierarchical Inheritance A class called Bill has the following protected data member variables Datatype Variable name int billNumber string name Include the following member function in the Bill class Member function Description void generateBill() This function is used to display the bill number and customer name. A class called TelePhoneBill inherits the class Bill with the following private data member variables Datatype Variable name int numberOfCallsMade double costPerCall Include a 4 arguments constructor with the arguments in the order (int billNumber, string name, int numberOfCallsMade, double costPerCall). Include the following member function in the TelePhoneBill class Member function Description void display() This function is used to display the telephone bill details A class called CurrentBill inherits the class Bill with the following private data member variables Datatype Variable name int numberOfUnitsConsumed double costPerUnit Include a 4 arguments constructor with the arguments in the order (int billNumber, string name, int numberOfUnitsConsumed, double costPerUnit). Include the following member function in the CurrentBill class Member function Description void display() This function is used to display the current bill details In the main method get the user input in the console and create an instance of the above-mentioned classes to assign the inputs and display the details by calling the display method. Input and Output Format: Input consists of the Telephone bill and the Current bill details of the customer. The output consists of the Telephone bill number, customer name, and telephone bill amount. Then Current bill number, customer name, and the current bill amount. Refer sample input and output for formatting specifications. Note: The double values should be round off up to 2 decimal places. Sample Input and Output: [All text in bold corresponds to input and the rest corresponds to output.] Enter the Telephone Bill Number : 12345 Enter the Customer Name : Suresh Enter the Number of Calls made : 300 Enter the Cost per Call : 0.75 Enter the Current Bill Number : 5678 Enter the Customer Name : Raja Enter the Number of Units Consumed : 500 Enter the Cost per Unit : 1.50 Bill Number : 12345 Customer Name : Suresh Telephone Bill Amount : 225.00 Bill Number : 5678 Customer Name : Raja Current Bill Amount : 750.00 --------------------TEMPLATES TO USE WHLE MAKING SOLUTION--------------- MAIN.CPP #include #include "TelephoneBill.cpp" #include "CurrentBill.cpp" using namespace std; int main() { //fill your code here return 0; } telephonebill.cpp #include #include #include"Bill.cpp" using namespace std; class TelephoneBill : public Bill{ private: int numberOfCallsMade; double costPerCall; public: //fill your code here void display(){ //fill your code here } }; currentbill.cpp #include #include #include"Bill.cpp" using namespace std; class CurrentBill : public Bill{ private: int numberOfUnitsConsumed; double costPerUnit; public: //fill your code here void display(){ //fill your code here } }; bill.cpp #ifndef MYHEADER_H #define MYHEADER_H #include #include using namespace std; class Bill{ protected: int billNumber; string name; public: Bill(){} Bill(int billNumber, string name) { this->billNumber = billNumber; this->name = name; } void generateBill(){ //fill your code here } }; #endif
USE TEMPLATE PROVIDED AT END OF QUESTION WHILE MAKING SOLUTION
-----------------------------------
Write a program to implement Hierarchical Inheritance
A class called Bill has the following protected data member variables
Datatype | Variable name |
int | billNumber |
string | name |
Include the following member function in the Bill class
Member function | Description |
void generateBill() | This function is used to display the bill number and customer name. |
A class called TelePhoneBill inherits the class Bill with the following private data member variables
Datatype | Variable name |
int | numberOfCallsMade |
double | costPerCall |
Include a 4 arguments constructor with the arguments in the order (int billNumber, string name, int numberOfCallsMade, double costPerCall).
Include the following member function in the TelePhoneBill class
Member function | Description |
void display() | This function is used to display the telephone bill details |
A class called CurrentBill inherits the class Bill with the following private data member variables
Datatype | Variable name |
int | numberOfUnitsConsumed |
double | costPerUnit |
Include a 4 arguments constructor with the arguments in the order (int billNumber, string name, int numberOfUnitsConsumed, double costPerUnit).
Include the following member function in the CurrentBill class
Member function | Description |
void display() | This function is used to display the current bill details |
In the main method get the user input in the console and create an instance of the above-mentioned classes to assign the inputs and display the details by calling the display method.
Input and Output Format:
Input consists of the Telephone bill and the Current bill details of the customer.
The output consists of the Telephone bill number, customer name, and telephone bill amount.
Then Current bill number, customer name, and the current bill amount.
Refer sample input and output for formatting specifications.
Note: The double values should be round off up to 2 decimal places.
Sample Input and Output:
[All text in bold corresponds to input and the rest corresponds to output.]
Enter the Telephone Bill Number :
12345
Enter the Customer Name :
Suresh
Enter the Number of Calls made :
300
Enter the Cost per Call :
0.75
Enter the Current Bill Number :
5678
Enter the Customer Name :
Raja
Enter the Number of Units Consumed :
500
Enter the Cost per Unit :
1.50
Bill Number : 12345
Customer Name : Suresh
Telephone Bill Amount : 225.00
Bill Number : 5678
Customer Name : Raja
Current Bill Amount : 750.00
--------------------TEMPLATES TO USE WHLE MAKING SOLUTION---------------
MAIN.CPP
#include <iostream> int main() |
telephonebill.cpp
#include <iostream> #include<stdio.h> #include"Bill.cpp" using namespace std; class TelephoneBill : public Bill{ private: int numberOfCallsMade; double costPerCall; public: //fill your code here void display(){ //fill your code here } }; |
currentbill.cpp
#include <iostream> #include<stdio.h> #include"Bill.cpp" using namespace std; class CurrentBill : public Bill{ private: int numberOfUnitsConsumed; double costPerUnit; public: //fill your code here void display(){ //fill your code here } }; |
bill.cpp
#ifndef MYHEADER_H public:
|

Step by step
Solved in 2 steps with 1 images

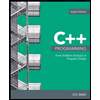
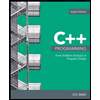