Use C++ Modify the code to have operator overloading functions with the following operators. ==, <, <=, >, >=, +, -, *, / Use the Rational interface and main code in the next page. I need three files one main.cpp, one rational.cpp file and one rational.h file. Main file and h file are shown on the picture they don't need to be changed I just need rational.cpp file. Here is the rational.cpp file I have: #include "rational.h" Rational::Rational() { numerator = 0; denominator = 1; } Rational::Rational(int num, int den) { numerator = num; denominator = den; } istream& operator >>(istream& take, Rational& r1) { string userInput = " "; string num = " "; string den = " "; bool findSlash = false; take >> userInput; for (int i = 0; i < userInput.length(); i++) { if (userInput[i] != '/' && !findSlash) { num = num + userInput[i]; } else if (userInput[i] == '/') { findSlash = true; } else if (userInput[i] != '/' && !findSlash) { den = den + userInput[i]; } } r1.numerator = stoi(num); r1.denominator = stoi(den); return take; } ostream& operator<<(ostream& out, const Rational& r1) { Rational temp(r1.numerator, r1.denominator); temp.simplify(); out << temp.numerator << "/" << temp.denominator; return out; } Rational operator+(const Rational&f, const Rational&r) { Rational temp((f.numerator * r.denominator + r.numerator * f.denominator), (f.denominator * r.denominator)); return temp; } Rational operator-(const Rational&f, const Rational&r) { Rational temp((f.numerator * r.denominator - f.denominator * r.numerator), (f.denominator * r.denominator)); return temp; } Rational operator*(const Rational&f, const Rational&r) { Rational temp((f.numerator * r.numerator), (f.denominator * r.denominator)); return temp; } Rational operator/(const Rational&f, const Rational&r) { Rational temp((f.numerator * r.denominator), (f.denominator * r.numerator)); return temp; } bool operator==(const Rational& f, const Rational& r) { } bool operator>(const Rational&, const Rational&) { } bool operator<(const Rational&, const Rational&) { } bool operator>=(const Rational&, const Rational&) { } bool operator<=(const Rational&, const Rational&) { } void Rational::simplify() { }
Use C++
Modify the code to have operator overloading functions with the following operators.
==, <, <=, >, >=, +, -, *, /
Use the Rational interface and main code in the next page.
I need three files one main.cpp, one rational.cpp file and one rational.h file. Main file and h file are shown on the picture they don't need to be changed I just need rational.cpp file.
Here is the rational.cpp file I have:
#include "rational.h"
Rational::Rational()
{
numerator = 0;
denominator = 1;
}
Rational::Rational(int num, int den)
{
numerator = num;
denominator = den;
}
istream& operator >>(istream& take, Rational& r1)
{
string userInput = " ";
string num = " ";
string den = " ";
bool findSlash = false;
take >> userInput;
for (int i = 0; i < userInput.length(); i++)
{
if (userInput[i] != '/' && !findSlash)
{
num = num + userInput[i];
}
else if (userInput[i] == '/')
{
findSlash = true;
}
else if (userInput[i] != '/' && !findSlash)
{
den = den + userInput[i];
}
}
r1.numerator = stoi(num);
r1.denominator = stoi(den);
return take;
}
ostream& operator<<(ostream& out, const Rational& r1)
{
Rational temp(r1.numerator, r1.denominator);
temp.simplify();
out << temp.numerator << "/" << temp.denominator;
return out;
}
Rational operator+(const Rational&f, const Rational&r)
{
Rational temp((f.numerator * r.denominator + r.numerator * f.denominator), (f.denominator * r.denominator));
return temp;
}
Rational operator-(const Rational&f, const Rational&r)
{
Rational temp((f.numerator * r.denominator - f.denominator * r.numerator), (f.denominator * r.denominator));
return temp;
}
Rational operator*(const Rational&f, const Rational&r)
{
Rational temp((f.numerator * r.numerator), (f.denominator * r.denominator));
return temp;
}
Rational operator/(const Rational&f, const Rational&r)
{
Rational temp((f.numerator * r.denominator), (f.denominator * r.numerator));
return temp;
}
bool operator==(const Rational& f, const Rational& r)
{
}
bool operator>(const Rational&, const Rational&)
{
}
bool operator<(const Rational&, const Rational&)
{
}
bool operator>=(const Rational&, const Rational&)
{
}
bool operator<=(const Rational&, const Rational&)
{
}
void Rational::simplify()
{
}



Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

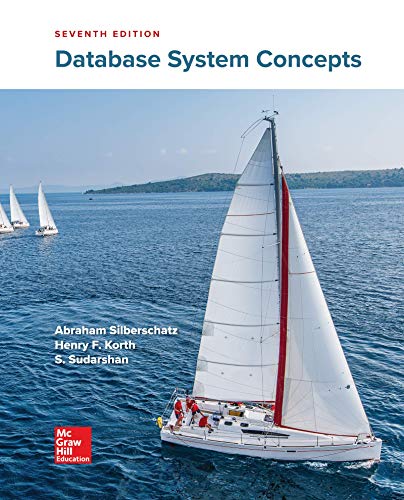
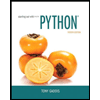
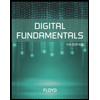
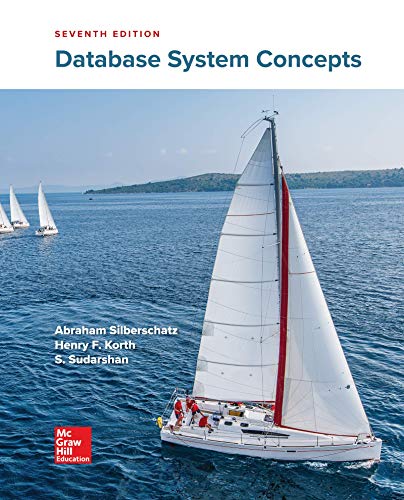
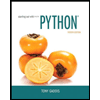
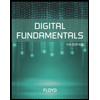
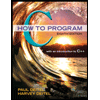
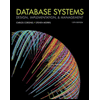
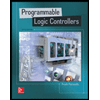