Uising the code below. Write a test class called CircleTest that implement below specification: a). The class has an ArrayList that holds objects of type Circle. b). It displays a menu that allows the user to: Enter a Circle (the user only needs to enter the radius). Print all Circles (print the toString for each Circle in the ArrayList). Quit. c). If the user selects option 1, allow the user to input the radius of a circle. Place the circle in the ArrayList in order. You can do this by using loops and the CompareTo method. Do not use methods of ArrayList for this part. Use the compareTo method to insert elements into the array. For example addAt. d) To add a circle Cases: The ArrayList is empty The new circle is less than the first circle, add it at the beginning. The circle is greater than the last circle, add it at the end The new circle belongs somewhere in the middle. e). If the user selects option 2, loop through the ArrayList and print the toString for each member of the list. import java.lang.Math;public class Circle implements Comparable<Circle> {private double radius;public Circle(double radius) {this.radius = radius;}public double findArea() {return Math.PI * Math.pow(this.radius, 2);}public double findCircumference() {return 2 * Math.PI * this.radius;}@Overridepublic boolean equals(Object obj) {if (this == obj) return true;if (obj == null || getClass() != obj.getClass()) return false;Circle circle = (Circle) obj;return Double.compare(circle.radius, radius) == 0;}@Overridepublic String toString() {return "Radius: " + this.radius + " Area: " + this.findArea() + " Circumference: " + this.findCircumference();}@Overridepublic int compareTo(Circle c) {return Double.compare(this.radius, c.radius);}}
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Uising the code below. Write a test class called CircleTest that implement below specification:
a). The class has an ArrayList that holds objects of type Circle.
b). It displays a menu that allows the user to:
- Enter a Circle (the user only needs to enter the radius).
- Print all Circles (print the toString for each Circle in the ArrayList).
- Quit.
c). If the user selects option 1, allow the user to input the radius of a circle. Place the circle in the ArrayList in order. You can do this by using loops and the CompareTo method. Do not use methods of ArrayList for this part. Use the compareTo method to insert elements into the array. For example addAt.
d) To add a circle
Cases:
- The ArrayList is empty
- The new circle is less than the first circle, add it at the beginning.
- The circle is greater than the last circle, add it at the end
- The new circle belongs somewhere in the middle.
e). If the user selects option 2, loop through the ArrayList and print the toString for each member of the list.
public class Circle implements Comparable<Circle> {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double findArea() {
return Math.PI * Math.pow(this.radius, 2);
}
public double findCircumference() {
return 2 * Math.PI * this.radius;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Circle circle = (Circle) obj;
return Double.compare(circle.radius, radius) == 0;
}
@Override
public String toString() {
return "Radius: " + this.radius + " Area: " + this.findArea() + " Circumference: " + this.findCircumference();
}
@Override
public int compareTo(Circle c) {
return Double.compare(this.radius, c.radius);
}
}
Unlock instant AI solutions
Tap the button
to generate a solution
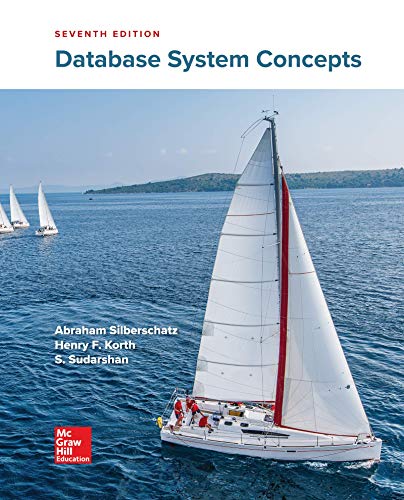
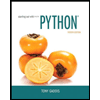
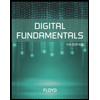
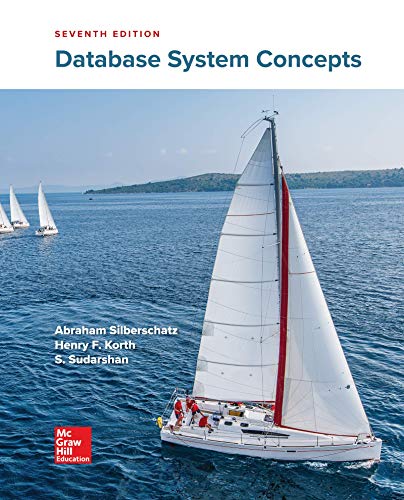
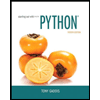
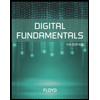
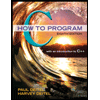
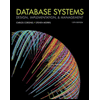
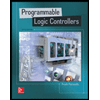