Timer Class Concepts tested: Classes 1) Write a class called Timer that models a stopwatch with start, stop, and reset buttons. It has the following members: a) -int startTime; // variable tracking when the timer was started (unused when timer is not running). In number of seconds since 1970 b) -int timeOnClock; // variable tracking the time on the clock since last reset in seconds c) -bool isRunning; //variable tracking wether the clock is running or stopped d) +Timer(); //constructor, initializes all to zero/false e) +Timer(bool startNow); // constructur that starts clock automatically if startNow is true f) +int getTime(); // returns time on clock in seconds. If clock is running, this must also include the time since it was last started g) +void addTimeToClock(int seconds); // allows manually adding time to the clock h) +void reset(); // resets time on clock to zero i) +void start(); // starts clock j) +void stop(); // stops clock k) +void printTime(); // prints the time (via cout) EXACTLY in the format 0h:0m:0s 2) Although you would normally create separate cpp and header files for your code, you must define and fully implement the class in the main file due to the constraints of the lab environment 3) Class name and class member names MUST BE EXACTLY THE SAME as above Example: --If the timer is started and stopped after 2 seconds, a call getTime should return int integer 2. A call to printTime should print "0h:0m:2s". --If the same timer is then started and stopped after an hour, getTime() should return 3602 and printTime() should print "1h:0m:2s"; --If addTimeToClock(120) is then run, printTime() should print "1h:2m:2s"; --If timer is reset, getTime() should return 0, and printTime() should print "0h:0m:0s". code given: #include // OTHER INCLUDES??? using namespace std; // YOUR CODE HERE #include "testcode.h" // For automatic grading purposes DO NOT REMOVE (but you can comment out temporarily for testing) int main() { testTimerClass(); // Test code for automatic grading. DO NOT REMOVE (but you can temporarily comment out for your own testing). Remove any test code you may have added prior to final submission. } Additional Files: These files will automatically be compiled with your code. You cannot modify them. testcode.h using namespace std; void testGetTime(){ Timer timer(true); system("sleep 2"); cout << timer.getTime() << std::endl; } void testStartAndStop(){ Timer timer(true); system("sleep 1"); timer.printTime(); timer.stop(); system("sleep 1"); timer.printTime(); timer.start(); system("sleep 1"); timer.printTime(); timer.stop(); system("sleep 1"); timer.printTime(); } void testAddTimeToClock(){ Timer timer; timer.addTimeToClock(60); cout << timer.getTime() << std::endl; } void testReset(){ Timer timer; timer.addTimeToClock(60); timer.printTime(); timer.reset(); timer.printTime(); } void testHoursMinutes(){ Timer timer; timer.addTimeToClock(90); timer.printTime(); timer.addTimeToClock(3600 * 2); timer.printTime(); } void testTimerClass(){ int testNumber; cin >> testNumber; switch (testNumber){ case 1: testStartAndStop(); break; case 2: testAddTimeToClock(); break; case 3: testReset(); break; case 4: testHoursMinutes(); break; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Timer Class
Concepts tested: Classes
1) Write a class called Timer that models a stopwatch with start, stop, and reset buttons. It has the following members:
a) -int startTime; // variable tracking when the timer was started (unused when timer is not running). In number of seconds since 1970
b) -int timeOnClock; // variable tracking the time on the clock since last reset in seconds
c) -bool isRunning; //variable tracking wether the clock is running or stopped
d) +Timer(); //constructor, initializes all to zero/false
e) +Timer(bool startNow); // constructur that starts clock automatically if startNow is true
f) +int getTime(); // returns time on clock in seconds. If clock is running, this must also include the time since it was last started
g) +void addTimeToClock(int seconds); // allows manually adding time to the clock
h) +void reset(); // resets time on clock to zero
i) +void start(); // starts clock
j) +void stop(); // stops clock
k) +void printTime(); // prints the time (via cout) EXACTLY in the format 0h:0m:0s<newline>
2) Although you would normally create separate cpp and header files for your code, you must define and fully implement the class in the main file due to the constraints of the lab environment
3) Class name and class member names MUST BE EXACTLY THE SAME as above
Example:
--If the timer is started and stopped after 2 seconds, a call getTime should return int integer 2. A call to printTime should print "0h:0m:2s".
--If the same timer is then started and stopped after an hour, getTime() should return 3602 and printTime() should print "1h:0m:2s";
--If addTimeToClock(120) is then run, printTime() should print "1h:2m:2s";
--If timer is reset, getTime() should return 0, and printTime() should print "0h:0m:0s".
#include <iostream>
// OTHER INCLUDES???
using namespace std;
// YOUR CODE HERE
#include "testcode.h" // For automatic grading purposes DO NOT REMOVE (but you can comment out temporarily for testing)
int main() {
testTimerClass(); // Test code for automatic grading. DO NOT REMOVE (but you can temporarily comment out for your own testing). Remove any test code you may have added prior to final submission.
}
Additional Files:
These files will automatically be compiled with your code. You cannot modify them.
testcode.h
using namespace std;
void testGetTime(){
Timer timer(true);
system("sleep 2");
cout << timer.getTime() << std::endl;
}
void testStartAndStop(){
Timer timer(true);
system("sleep 1");
timer.printTime();
timer.stop();
system("sleep 1");
timer.printTime();
timer.start();
system("sleep 1");
timer.printTime();
timer.stop();
system("sleep 1");
timer.printTime();
}
void testAddTimeToClock(){
Timer timer;
timer.addTimeToClock(60);
cout << timer.getTime() << std::endl;
}
void testReset(){
Timer timer;
timer.addTimeToClock(60);
timer.printTime();
timer.reset();
timer.printTime();
}
void testHoursMinutes(){
Timer timer;
timer.addTimeToClock(90);
timer.printTime();
timer.addTimeToClock(3600 * 2);
timer.printTime();
}
void testTimerClass(){
int testNumber;
cin >> testNumber;
switch (testNumber){
case 1: testStartAndStop(); break;
case 2: testAddTimeToClock(); break;
case 3: testReset(); break;
case 4: testHoursMinutes(); break;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

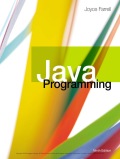
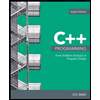
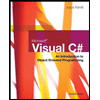
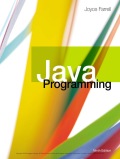
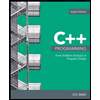
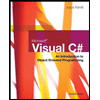
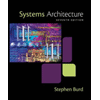