The Inheritance hierarchy is as follows: (Be sure to create this hierarchy correctly and efficiently; taking advantage of inheritance, polymorphism and exception handling concepts discussed in previous modules) A company pays its personnel on a weekly basis. The personnel are of 4 types: FixedWeekly personnel are paid a fixed amount regardless of the number of hours worked ByTheHour personnel are paid by the hour and receive overtime pay for all hours worked in excess of 40 PercentOfSales personnel are paid a percentage of their sales FixedWeeklyPercentOfSales personnel receive a fixed amount plus a percentage of their sales. All variables are private, thus all of them will have public getters and setters Create a class called Personnel. This class will represent the general concept of all personnel. All 4 types of personnel are considered Personnel. FixedWeeklyPercentOfSales personnel are considered to be PercentOfSales Personnel. The Personnel class will also be Payable. You will need to create the Payable interface. It will contain just one method called earnings(). Personnel have a first name, last name, and social security number. FixedWeekly personnel have a first name, last name, social security number and fixed weekly salary. ByTheHour personnel have a first name, last name, social security number, an hourly wage, and number of hours worked. PercentOfSales personnel have a first name, last name, social security number, gross sales amount, and commission rate. FixedWeeklyPercentOfSales personnel have a first name, last name, social security number, gross sales amount, commission rate and a fixed weekly salary
Code in JAVA and please see attached images on specifications
The Inheritance hierarchy is as follows: (Be sure to create this hierarchy correctly and efficiently; taking advantage of inheritance, polymorphism and exception handling concepts discussed in previous modules)
A company pays its personnel on a weekly basis. The personnel are of 4 types:
FixedWeekly personnel are paid a fixed amount regardless of the number of hours worked
ByTheHour personnel are paid by the hour and receive overtime pay for all hours worked in excess of 40
PercentOfSales personnel are paid a percentage of their sales
FixedWeeklyPercentOfSales personnel receive a fixed amount plus a percentage of their sales.
All variables are private, thus all of them will have public getters and setters
Create a class called Personnel. This class will represent the general concept of all personnel. All 4 types of personnel are considered Personnel. FixedWeeklyPercentOfSales personnel are considered to be PercentOfSales Personnel.
The Personnel class will also be Payable. You will need to create the Payable interface. It will contain just one method called earnings().
Personnel have a first name, last name, and social security number. FixedWeekly personnel have a first name, last name, social security number and fixed weekly salary. ByTheHour personnel have a first name, last name, social security number, an hourly wage, and number of hours worked. PercentOfSales personnel have a first name, last name, social security number, gross sales amount, and commission rate. FixedWeeklyPercentOfSales personnel have a first name, last name, social security number, gross sales amount, commission rate and a fixed weekly salary.



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 18 images

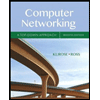
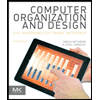
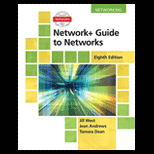
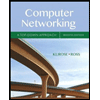
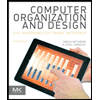
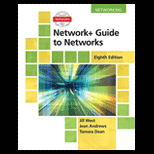
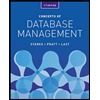
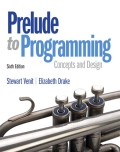
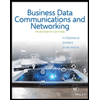