The binary search algorithm that follows may be used to search an array when the elements are in order. This algorithm is analogous to the following approach to finding a name in a telephone book. a. Open the book in the middle and look at the middle name on the page. b. b. If the middle name isn't the one, you're looking for, decide whether it comes before or after the name you want. c. Take the appropriate half of the section of the book you were looking in and repeat these steps until you land on the name. 1. Let the bottom be the subscript of the initial array element. 2. Let the top be the subscript of the last array element. 3. Let found be false. 4. Repeat as long as the bottom isn't greater than the top and the target has not been found. 5. Let middle be the subscript of the element halfway between bottom and top. 6. If the element in the middle is the target 7. Set found to true and index to middle. else if the element in the middle is larger than the target 8. Let the top be middle - 1. else 9. Let bottom be middle +1 Develop a recursive binary search algorithm and write and test a function binary_srch that implements the algorithm for an array of integers Use the following example to write your code. #include #include // Function to perform binary search on an array of names int binary_srch(const char* arr[], const char* target, int bottom, int top) { //Your code Here } int main() { const char* names[] = {"Alice", "Bob", "Charlie", "David", "Eve", "Frank", "Grace", "Hannah", "Isabel", "Jack"}; char target[100]; printf("Enter the name to search: "); scanf("%s", target); //Your code here return 0; }
The binary search
elements are in order. This algorithm is analogous to the following approach to finding a
name in a telephone book.
a. Open the book in the middle and look at the middle name on the page.
b. b. If the middle name isn't the one, you're looking for, decide whether it comes
before or after the name you want.
c. Take the appropriate half of the section of the book you were looking in and repeat
these steps until you land on the name.
1. Let the bottom be the subscript of the initial array element.
2. Let the top be the subscript of the last array element.
3. Let found be false.
4. Repeat as long as the bottom isn't greater than the top and the target has not
been found.
5. Let middle be the subscript of the element halfway between bottom and top.
6. If the element in the middle is the target
7. Set found to true and index to middle. else if the element in the middle is larger
than the target
8. Let the top be middle - 1. else 9. Let bottom be middle +1
Develop a recursive binary search algorithm and write and test a function
binary_srch that implements the algorithm for an array of integers
Use the following example to write your code.
#include <stdio.h>
#include <string.h>
// Function to perform binary search on an array of names
int binary_srch(const char* arr[], const char* target, int
bottom, int top) {
//Your code Here
}
int main() {
const char* names[] = {"Alice", "Bob", "Charlie", "David",
"Eve", "Frank", "Grace", "Hannah", "Isabel", "Jack"};
char target[100];
printf("Enter the name to search: ");
scanf("%s", target);
//Your code here
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

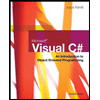
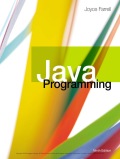
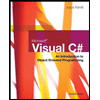
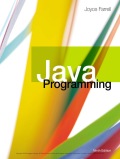