The 3n+ 1 problem is based on a famous sequence in mathematics that follows a very simple rule: • If the number is even, the next number in the sequence is its half, • If the number is odd, the next number in the sequence is three times the number plus one. In mathematical notation, the 3n+ 1 problem sequence is given as: an+1 = if an is even if an is odd 3an +1 It has been conjectured that for any positive integer number, the sequence will always end in 4, 2, 1. So, if the sequence starts with 7, the sequence is 7 22 11 34 17 52 26 13 40 20 10 5 168421 In this task, you are required to write a program that takes as input two positive inputs, one for the start of the sequence, followed by an index. Your code must: • Generate the above sequence inside a 1D array. Your code must stop when it reaches the last number (i.e., 1). • Your code must print the sequence number specified by the index. If the input index is larger than the sequence length, then the code outputs NA • Your code must also find and print the highest number the sequence reaches. • You must check if the inputs are positive numbers; otherwise, convert them to a positive number. • You must ensure if the start point is not a zero or one; otherwise, print out "Invalid"
The 3n+ 1 problem is based on a famous sequence in mathematics that follows a very simple rule: • If the number is even, the next number in the sequence is its half, • If the number is odd, the next number in the sequence is three times the number plus one. In mathematical notation, the 3n+ 1 problem sequence is given as: an+1 = if an is even if an is odd 3an +1 It has been conjectured that for any positive integer number, the sequence will always end in 4, 2, 1. So, if the sequence starts with 7, the sequence is 7 22 11 34 17 52 26 13 40 20 10 5 168421 In this task, you are required to write a program that takes as input two positive inputs, one for the start of the sequence, followed by an index. Your code must: • Generate the above sequence inside a 1D array. Your code must stop when it reaches the last number (i.e., 1). • Your code must print the sequence number specified by the index. If the input index is larger than the sequence length, then the code outputs NA • Your code must also find and print the highest number the sequence reaches. • You must check if the inputs are positive numbers; otherwise, convert them to a positive number. • You must ensure if the start point is not a zero or one; otherwise, print out "Invalid"
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
please can you do that in c++
Sample Testcase 0:
Input:
7
8
Output:
40
52
Sample Testcase 1:
Input:
7
19
Output:
NA
52
Sample Testcase 2:
Input:
-10
-5
Output:
2
16
#include <cmath>

Transcribed Image Text:The 3n+ 1 problem is based on a famous sequence in mathematics that follows a very simple rule:
• If the number is even, the next number in the sequence is its half,
• If the number is odd, the next number in the sequence is three times the number plus one.
In mathematical notation, the 3n+ 1 problem sequence is given as:
an
if an is even
2
an+1 =
3an
+1
if an is odd
It has been conjectured that for any positive integer number, the sequence will always end in 4, 2, 1.
So, if the sequence starts with 7, the sequence is 7 22 11 34 17 52 26 13 40 20 10 5 16 8 4 2 1
In this task, you are required to write a program that takes as input two positive inputs, one for the start of the sequence, followed by an index.
Your code must:
• Generate the above sequence inside a 1D array. Your code must stop when it reaches the last number (i.e., 1).
• Your code must print the sequence number specified by the index. If the input index is larger than the sequence length, then the code outputs NA
• Your code must also find and print the highest number the sequence reaches.
• You must check if the inputs are positive numbers; otherwise, convert them to a positive number.
• You must ensure if the start point is not a zero or one; otherwise, print out "Invalid"

Transcribed Image Text:IMPORTANT NOTE
• Do not add any cout statements except for the final answers as specified above.
• Do not add "Enter a number", "the number of digits is" or any similar prompts.
• Also note that the automatic grader is case-sensitive; so "na" is wrong but "NA" is correct.
• Do not add any unnecessary spaces inside the strings of cout statements " " unless we ask you to.
• You may add any libraries needed.
1/0
Program Input:
• One number that indicates the starting number of the sequence
• One number that specified the index of the number to be printed from the sequence
Program Output:
• One line that prints the sequence number at the specified index
• One line that outputs the highest number in the sequence.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
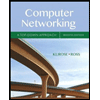
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
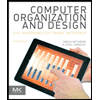
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
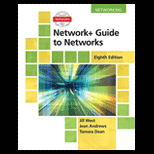
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
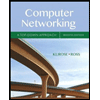
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
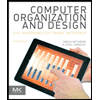
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
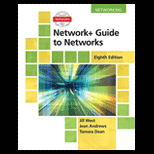
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
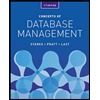
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
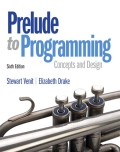
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
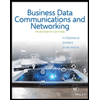
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY