Sample Console Output Baseball Team Manager CURRENT DATE: 2020-07-12 GAME DATE: DAYS UNTIL GAME: 7 2020-07-19 MENU OPTIONS 1 - Display lineup 2 - Add player 3 - Remove player 4 - Move player 5 - Edit player position 6 - Edit player stats 7 - Exit program POSITIONS с, 1в, 2в, зв, ss, LP, CP, RP, P Menu option: 1 Player POS AB AVG 1 Dominick Gilbert 2 Craig Mitchell 3 Jack Quinn 4 Simon Harris 5 Darryl Moss 6 Grady Guzman 7 Wallace Cruz 8 Cedric Cooper 9 Alberto Gomez 1B СР 545 396 170 125 0.317 0.316 RF 345 450 99 135 0.287 0.300 3B 501 120 0.240 SS LF 463 443 114 131 0.246 0.296 2B 165 72 54 19 0.327 0.264 Menu option: General Specifications Use a Player class that provides attributes that store the playerID, batOrder, first name, last name, position, at bats, and hits for a player. The class constructor should use these seven attributes as parameters. This class should also provide a method that returns the full name of a player and a method that returns the batting average for a player. • The playerlID is not used when adding a player to the lineup. A PlayerID, the primary key for the Player table, is automatically created by the database. • Use a Lineup class to store the lineup for the team as a list of player objects. This class should include methods with appropriate parameters that allow you to add, remove, move, and edit a player. In addition, it should include an iterator so you can easily loop through each player in the lineup. A Lineup object replaces the list that holds all players. Use the same console Input/output system that was used in project 3. The file for this system will be named UI. • Use a file named Objects to store the code for the Player and Lineup classes. Use a file named DB to store the functions that work with the file that stores the data. A db_comments.py file is included as a template for the DB file that must be turned in.
SQL
SQL stands for Structured Query Language, is a form of communication that uses queries structured in a specific format to store, manage & retrieve data from a relational database.
Queries
A query is a type of computer programming language that is used to retrieve data from a database. Databases are useful in a variety of ways. They enable the retrieval of records or parts of records, as well as the performance of various calculations prior to displaying the results. A search query is one type of query that many people perform several times per day. A search query is executed every time you use a search engine to find something. When you press the Enter key, the keywords are sent to the search engine, where they are processed by an algorithm that retrieves related results from the search index. Your query's results are displayed on a search engine results page, or SER.
#Fill in comments to spec.
import sqlite3
from contextlib import closing
from Objects import Player, Lineup
conn = None
def connect():
global conn
if not conn:
DB_FILE = "player_db.sqlite"
conn = sqlite3.connect(DB_FILE)
conn.row_factory = sqlite3.Row
def close():
if conn:
conn.close()
def get_players():
return None #remove this line when code is added.
# SQL statement to select all 7 fields for all players
# Use a with statement to execute the query
# Create a lineup object
# use a loop to populate the lineup object with player objects
# return the lineup object
def get_player(playerID):
return None #remove this line when code is added.
# SQL statement to select all 7 fields for a player
# Use a with statement to execute the query & return a player object if the player exists
def add_player(player):
return None #remove this line when code is added.
# SQL statement to insert 6 fields for a player added to the table
# Use a with statement to execute the query
def delete_player(player):
return None #remove this line when code is added.
# SQL statement to delete a single player
# Use a with statement to execute the query
def update_bat_order(lineup):
return None #remove this line when code is added.
# Use a loop to call a SQL statement that updates
# the batOrder for each player based on their playerID
# Use a with statement to execute the query
def update_player(player):
return None #remove this line when code is added.
# SQL statement to update 6 fields of a player based on the playerID
# Use a with statement to execute the query
def main():
# code to test the get_players function
connect()
players = get_players()
if players != None:
for player in players:
print(player.batOrder, player.firstName, player.lastName,
player.position, player.atBats, player.hits, player.getBattingAvg())
else:
print("Code is needed for the get_players function.")
if __name__ == "__main__":
main()


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

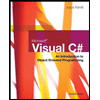
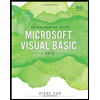
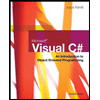
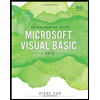