public class Date { privateintyear; privateintmonth; privateintday; public Date() { } public Date(intyear, intmonth, intday) { this.year = year; this.month = month; this.day = day; } publicint getYear() { returnyear; } publicint getMonth() { returnmonth; } publicint getDay() { returnday; } @Override public String toString() { return"Date [year=" + year + ", month=" + month + ", day=" + day + "]"; } } public class Date { privateintyear; privateintmonth; privateintday; public Date() { } public Date(intyear, intmonth, intday) { this.year = year; this.month = month; this.day = day; } publicint getYear() { returnyear; } publicint getMonth() { returnmonth; } publicint getDay() { returnday; } @Override public String toString() { return"Date [year=" + year + ", month=" + month + ", day=" + day + "]"; } } public class Date { privateintyear; privateintmonth; privateintday; public Date() { } public Date(intyear, intmonth, intday) { this.year = year; this.month = month; this.day = day; } publicint getYear() { returnyear; } publicint getMonth() { returnmonth; } publicint getDay() { returnday; } @Override public String toString() { return"Date [year=" + year + ", month=" + month + ", day=" + day + "]"; } } public class Mailbox { private String client; private Email[] emails; privateintactualSize; /** * Default Constructor creates new Email array with maximum of 15 email's sets * the client to Brad's inbox. */ public Mailbox() { this.emails = new Email[15]; actualSize = 0; this.client = "Brad's inbox"; } /** * * @param client calls the default consturctor sets client to client set in * Default Constructor */ public Mailbox(String client) { this(); this.client = client; } /** * * @return the email client */ public String getClient() { returnclient; } /** * * @return emails from emails Array */ public Email[] getEmails() { returnemails; } /** * * @return how many emails are in inbox */ publicint getActualSize() { returnactualSize; } /** * * @param email If there is no capacity there is a message to upgrade account If * not it adds to how many emails till maximum capacity is reached */ publicvoid addEmail(Email email) { if (this.actualSize >= 15) { System.out.println("You have reached maximum capacity. Upgrade your account. "); } else { emails[actualSize] = email; actualSize++; } } publicvoid sortEmailsByDate() { } /** * * @param year email was received * @return email from year and returns emails that match */ public Email findEmail(intyear) { for (inti = 0; i < this.actualSize; i++) { if (this.emails[i].getDate().getYear() == year) { returnthis.emails[i]; } } returnnull; } /** * * @return number of urgent emails */ publicint countUrgent() { intnumberOfEmails = 0; for (inti = 0; i < this.actualSize; i++) { if (this.emails[i].isUrgent()) { numberOfEmails++; } } returnnumberOfEmails; } /** * toString method that returns the client, the urgent emails, and the number of * emails */ @Override public String toString() { String mailboxInformation = "Mailbox for " + client + " - Urgent emails: " + countUrgent() + " - Emails:\n"; for (inti = 0; i < actualSize; i++) { mailboxInformation += emails[i].toString() + "\n"; } returnmailboxInformation; } } In the driver class, define three static methods in addition to the main method. a. displayMenu(): which will print the following: Hello! What would you like to do? 1- List Emails in a mailbox 2- Add an email to a mailbox 3- Search for an email in a mailbox by year 4- Sort emails in a mailbox by year 5- Quit b. findMailbox(String client): which loops through the mailboxes array (see main method description below) and return the mailbox object who’s client variable matches the client string parameter. c. addEmailToMailbox(String client): which will take a client name (e.g., gmail) and do the following: i. It will get the mailbox object corresponding to that client by calling findMailbox method ii. It will then prompt the user for the email data then allow the user to enter all of the email data on one line separated by commas: iii. It will then read the data from the line corresponding to each variable inside the Email class iv. Then, it will create a Date object from the month, day, year, and use that in addition to the subject and urgent flag to create an Email object v. Finally it will add the email object to the emails array of the desired mailbox object at the next available location. (Note: the addEmail method of the mailbox object should take care of verifying that we haven’t reached the maximum limit by displaying: You have reached maximum capacity. Upgrade your account In Java
public class Date
{
privateintyear;
privateintmonth;
privateintday;
public Date()
{
}
public Date(intyear, intmonth, intday)
{
this.year = year;
this.month = month;
this.day = day;
}
publicint getYear()
{
returnyear;
}
publicint getMonth()
{
returnmonth;
}
publicint getDay()
{
returnday;
}
@Override
public String toString()
{
return"Date [year=" + year + ", month=" + month + ", day=" + day + "]";
}
}
public class Date
{
privateintyear;
privateintmonth;
privateintday;
public Date()
{
}
public Date(intyear, intmonth, intday)
{
this.year = year;
this.month = month;
this.day = day;
}
publicint getYear()
{
returnyear;
}
publicint getMonth()
{
returnmonth;
}
publicint getDay()
{
returnday;
}
@Override
public String toString()
{
return"Date [year=" + year + ", month=" + month + ", day=" + day + "]";
}
}
public class Date
{
privateintyear;
privateintmonth;
privateintday;
public Date()
{
}
public Date(intyear, intmonth, intday)
{
this.year = year;
this.month = month;
this.day = day;
}
publicint getYear()
{
returnyear;
}
publicint getMonth()
{
returnmonth;
}
publicint getDay()
{
returnday;
}
@Override
public String toString()
{
return"Date [year=" + year + ", month=" + month + ", day=" + day + "]";
}
}
public class Mailbox
{
private String client;
private Email[] emails;
privateintactualSize;
/**
* Default Constructor creates new Email array with maximum of 15 email's sets
* the client to Brad's inbox.
*/
public Mailbox()
{
this.emails = new Email[15];
actualSize = 0;
this.client = "Brad's inbox";
}
/**
*
* @param client calls the default consturctor sets client to client set in
* Default Constructor
*/
public Mailbox(String client)
{
this();
this.client = client;
}
/**
*
* @return the email client
*/
public String getClient()
{
returnclient;
}
/**
*
* @return emails from emails Array
*/
public Email[] getEmails()
{
returnemails;
}
/**
*
* @return how many emails are in inbox
*/
publicint getActualSize()
{
returnactualSize;
}
/**
*
* @param email If there is no capacity there is a message to upgrade account If
* not it adds to how many emails till maximum capacity is reached
*/
publicvoid addEmail(Email email)
{
if (this.actualSize >= 15)
{
System.out.println("You have reached maximum capacity. Upgrade your account. ");
} else
{
emails[actualSize] = email;
actualSize++;
}
}
publicvoid sortEmailsByDate()
{
}
/**
*
* @param year email was received
* @return email from year and returns emails that match
*/
public Email findEmail(intyear)
{
for (inti = 0; i < this.actualSize; i++)
{
if (this.emails[i].getDate().getYear() == year)
{
returnthis.emails[i];
}
}
returnnull;
}
/**
*
* @return number of urgent emails
*/
publicint countUrgent()
{
intnumberOfEmails = 0;
for (inti = 0; i < this.actualSize; i++)
{
if (this.emails[i].isUrgent())
{
numberOfEmails++;
}
}
returnnumberOfEmails;
}
/**
* toString method that returns the client, the urgent emails, and the number of
* emails
*/
@Override
public String toString()
{
String mailboxInformation = "Mailbox for " + client + " - Urgent emails: " + countUrgent() + " - Emails:\n";
for (inti = 0; i < actualSize; i++)
{
mailboxInformation += emails[i].toString() + "\n";
}
returnmailboxInformation;
}
}
In the driver class, define three static methods in addition to the main method.
a. displayMenu(): which will print the following:
Hello! What would you like to do?
1- List Emails in a mailbox
2- Add an email to a mailbox
3- Search for an email in a mailbox by year
4- Sort emails in a mailbox by year
5- Quit
b. findMailbox(String client): which loops through the mailboxes array
(see main method description below) and return the mailbox object
who’s client variable matches the client string parameter.
c. addEmailToMailbox(String client): which will take a client name (e.g.,
gmail) and do the following:
i. It will get the mailbox object corresponding to that client by
calling findMailbox method
ii. It will then prompt the user for the email data then allow the
user to enter all of the email data on one line separated by
commas:
iii. It will then read the data from the line corresponding to each
variable inside the Email class
iv. Then, it will create a Date object from the month, day, year, and
use that in addition to the subject and urgent flag to create an
Email object
v. Finally it will add the email object to the emails array of the
desired mailbox object at the next available location. (Note: the
addEmail method of the mailbox object should take care of
verifying that we haven’t reached the maximum limit by
displaying:
You have reached maximum capacity. Upgrade your account
In Java

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

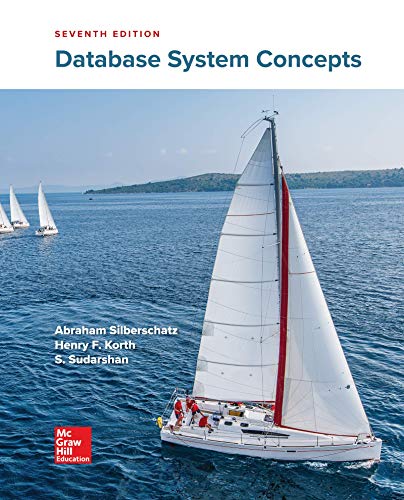
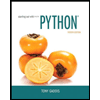
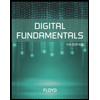
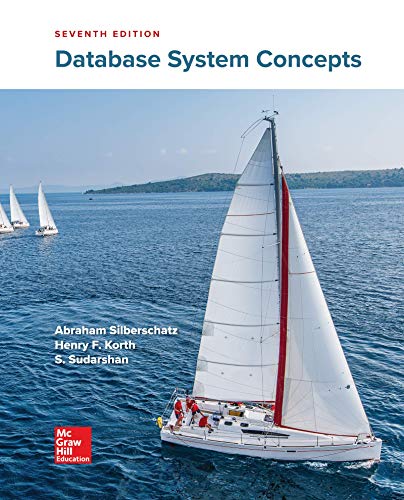
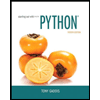
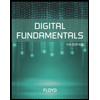
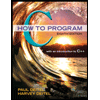
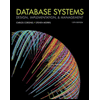
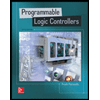