Prompt the user (the shopkeeper) to enter the name, type, price and burn time for each of the three different types of candles, types 1, 2, and 3. Once you have this information, instantiate three Candle objects, one for each type using the information the user provided. This step can be considered the store setup. Assume a customer wants to make a purchase. Prompt the user to enter the number of candles of each type the customer wants to buy. Since you can’t buy a fraction of a candle, the number bought for each type of candle must be an integer. (You may assume that only integer values between 0 and 10 will be entered when this program is tested.) Calculate the total price of all the candles bought using the information provided in step 1 and by calling the appropriate getter to find out the price of each type of candle. The shop owner wants to offer a discount based on the total purchase price. Deduct this discount from the purchase price. If the price is more than $20 but $35 or less they receive a 5% discount. If the price is more than $35 but $55 or less they receive a 7% discount. If the price is more than $55 but $100 or less they receive a 10% discount If the price is more than $100 they receive a 20% discount. Calculate the total burn time of all the candles if they were burned consecutively (i.e., one after the other). Again, use the appropriate getter of the Candle class to determine the individual burn time. Calculate the total cost-per-minute for that purchase using the discounted purchase price and the total burn time. Output some kind of meaningful display that includes the number of candles of each type bought, their name and type, the total price (before and after discount if applied), the total burn time, and the cost-per-minute to burn all the candles all the way down. You can be as creative as you want with this! Once you have a working solution, you can begin to use loops to create a histogram of stars that show the breakdown of the different candle types and the total number of candles purchased. The following class file has to be used and unaltered if possible class Candle { private String name; private int type; private double cost; private int burnTime; public Candle(String nam, int typ, double cst, int brn) { this.name = nam; this.type = typ; this.cost = cst; this.burnTime = brn; } public String getName() { return this.name; } public void setName(String nam) { this.name = nam; } public int getType() { return this.type; } public void getType(int typ) { this.type = typ; } public double getCost() { return this.cost; } public void setCost(double amount) { this.cost = amount; } public int getTime() { return this.burnTime; } public void setTime(int minutes) { this.burnTime = minutes; } }
Prompt the user (the shopkeeper) to enter the name, type, price and burn time for each of the three different types of candles, types 1, 2, and 3. Once you have this information, instantiate three Candle objects, one for each type using the information the user provided. This step can be considered the store setup. Assume a customer wants to make a purchase. Prompt the user to enter the number of candles of each type the customer wants to buy. Since you can’t buy a fraction of a candle, the number bought for each type of candle must be an integer. (You may assume that only integer values between 0 and 10 will be entered when this program is tested.) Calculate the total price of all the candles bought using the information provided in step 1 and by calling the appropriate getter to find out the price of each type of candle. The shop owner wants to offer a discount based on the total purchase price. Deduct this discount from the purchase price. If the price is more than $20 but $35 or less they receive a 5% discount. If the price is more than $35 but $55 or less they receive a 7% discount. If the price is more than $55 but $100 or less they receive a 10% discount If the price is more than $100 they receive a 20% discount. Calculate the total burn time of all the candles if they were burned consecutively (i.e., one after the other). Again, use the appropriate getter of the Candle class to determine the individual burn time. Calculate the total cost-per-minute for that purchase using the discounted purchase price and the total burn time. Output some kind of meaningful display that includes the number of candles of each type bought, their name and type, the total price (before and after discount if applied), the total burn time, and the cost-per-minute to burn all the candles all the way down. You can be as creative as you want with this! Once you have a working solution, you can begin to use loops to create a histogram of stars that show the breakdown of the different candle types and the total number of candles purchased. The following class file has to be used and unaltered if possible class Candle { private String name; private int type; private double cost; private int burnTime; public Candle(String nam, int typ, double cst, int brn) { this.name = nam; this.type = typ; this.cost = cst; this.burnTime = brn; } public String getName() { return this.name; } public void setName(String nam) { this.name = nam; } public int getType() { return this.type; } public void getType(int typ) { this.type = typ; } public double getCost() { return this.cost; } public void setCost(double amount) { this.cost = amount; } public int getTime() { return this.burnTime; } public void setTime(int minutes) { this.burnTime = minutes; } }
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter10: Classes And Objects
Section: Chapter Questions
Problem 2MQ2
Related questions
Question
100%
- Prompt the user (the shopkeeper) to enter the name, type, price and burn time for each of the three different types of candles, types 1, 2, and 3. Once you have this information, instantiate three Candle objects, one for each type using the information the user provided. This step can be considered the store setup.
- Assume a customer wants to make a purchase. Prompt the user to enter the number of candles of each type the customer wants to buy. Since you can’t buy a fraction of a candle, the number bought for each type of candle must be an integer. (You may assume that only integer values between 0 and 10 will be entered when this program is tested.)
- Calculate the total price of all the candles bought using the information provided in step 1 and by calling the appropriate getter to find out the price of each type of candle.
- The shop owner wants to offer a discount based on the total purchase price. Deduct this discount from the purchase price.
- If the price is more than $20 but $35 or less they receive a 5% discount.
- If the price is more than $35 but $55 or less they receive a 7% discount.
- If the price is more than $55 but $100 or less they receive a 10% discount
- If the price is more than $100 they receive a 20% discount.
- Calculate the total burn time of all the candles if they were burned consecutively (i.e., one after the other). Again, use the appropriate getter of the Candle class to determine the individual burn time.
- Calculate the total cost-per-minute for that purchase using the discounted purchase price and the total burn time.
- Output some kind of meaningful display that includes the number of candles of each type bought, their name and type, the total price (before and after discount if applied), the total burn time, and the cost-per-minute to burn all the candles all the way down. You can be as creative as you want with this!
- Once you have a working solution, you can begin to use loops to create a histogram of stars that show the breakdown of the different candle types and the total number of candles purchased.
- The following class file has to be used and unaltered if possible
-
class Candle {private String name;private int type;private double cost;private int burnTime;public Candle(String nam, int typ, double cst, int brn) {this.name = nam;this.type = typ;this.cost = cst;this.burnTime = brn;}public String getName() {return this.name;}public void setName(String nam) {this.name = nam;}public int getType() {return this.type;}public void getType(int typ) {this.type = typ;}public double getCost() {return this.cost;}public void setCost(double amount) {this.cost = amount;}public int getTime() {return this.burnTime;}public void setTime(int minutes) {this.burnTime = minutes;}}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
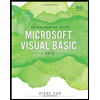
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
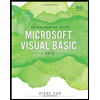
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning