Program Specifications Write a program to play an automated dice game that uses two dice (GVDie class provided). The player rolls both dice and either wins one credit, loses one credit, or sets a goal for future rolls. Current round ends when player wins or loses a credit. Game ends when credits are zero. Note: this program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. Step 0. Read starter template and do not change the provided code. Two GVDie objects are created. A random seed is read from input and passed to a die. This supports automated testing and creates predictable results that would otherwise be random. Starting credits is read from input. Step 1 . Roll both dice. Player wins one credit by rolling 7 or 11. Player losses one credit by rolling 2, 3, or 12. Otherwise, credits do not change and the player's goal is set to the dice total. The player's goal must be repeated in Step #2 to win a credit. GVDie class includes roll() and getValue() methods. Output dice total and credits. Submit for grading to confirm 3 tests pass. Ex: If input is: 24 10 Sample output is: Dice total: 7 Credits: 11 Step 2 . If player did not win or lose in step 1 (i.e. a goal was set), continue rolling both dice until one of two outcomes: 1) player rolls a 7 and loses one credit or 2) player rolls the goal value and wins one credit. Current round ends. Set goal to -1 and output credits. Submit for grading to confirm 5 tests pass. Ex: If input is: 33 7 Sample output is: Dice total: 8 Dice total: 12 Dice total: 7 Credits: 6 Step 3 Add a loop to repeat steps 1 and 2 until credits are zero. Count and output the number of rounds when game is over. Submit for grading to confirm all tests pass. Ex: If input is: 43 4 Sample output ends with: Credits: 2 Dice total: 8 Dice total: 7 Credits: 1 Dice total: 5 Dice total: 6 Dice total: 6 Dice total: 4 Dice total: 7 Credits: 0 Rounds: 162 import java.util.*; public class GVDie implements Comparable { // Static members are shared across all instances of class GVDie private static Random rand = new Random(); private int myValue; // Set initial die value public GVDie() { myValue = rand.nextInt(6) + 1; } // Roll the die to get 1 - 6 public void roll () { myValue = rand.nextInt(6) + 1; } // Return current die value public int getValue() { return myValue; } // Set the random number generator seed to support testing public void setSeed(int seed) { rand.setSeed(seed); } // Allows dice to be compared if necessary public int compareTo(GVDie d) { return getValue() - d.getValue(); } }
Program Specifications Write a program to play an automated dice game that uses two dice (GVDie class provided). The player rolls both dice and either wins one credit, loses one credit, or sets a goal for future rolls. Current round ends when player wins or loses a credit. Game ends when credits are zero.
Note: this program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress.
Step 0. Read starter template and do not change the provided code. Two GVDie objects are created. A random seed is read from input and passed to a die. This supports automated testing and creates predictable results that would otherwise be random. Starting credits is read from input.
Step 1 . Roll both dice. Player wins one credit by rolling 7 or 11. Player losses one credit by rolling 2, 3, or 12. Otherwise, credits do not change and the player's goal is set to the dice total. The player's goal must be repeated in Step #2 to win a credit. GVDie class includes roll() and getValue() methods. Output dice total and credits. Submit for grading to confirm 3 tests pass.
Ex: If input is:
24 10
Sample output is:
Dice total: 7 Credits: 11
Step 2 . If player did not win or lose in step 1 (i.e. a goal was set), continue rolling both dice until one of two outcomes: 1) player rolls a 7 and loses one credit or 2) player rolls the goal value and wins one credit. Current round ends. Set goal to -1 and output credits. Submit for grading to confirm 5 tests pass.
Ex: If input is:
33 7
Sample output is:
Dice total: 8 Dice total: 12 Dice total: 7 Credits: 6
Step 3 Add a loop to repeat steps 1 and 2 until credits are zero. Count and output the number of rounds when game is over. Submit for grading to confirm all tests pass.
Ex: If input is:
43 4
Sample output ends with:
Credits: 2 Dice total: 8 Dice total: 7 Credits: 1 Dice total: 5 Dice total: 6 Dice total: 6 Dice total: 4 Dice total: 7 Credits: 0 Rounds: 162
import java.util.*;
public class GVDie implements Comparable <GVDie> {
// Static members are shared across all instances of class GVDie
private static Random rand = new Random();
private int myValue;
// Set initial die value
public GVDie() {
myValue = rand.nextInt(6) + 1;
}
// Roll the die to get 1 - 6
public void roll () {
myValue = rand.nextInt(6) + 1;
}
// Return current die value
public int getValue() {
return myValue;
}
// Set the random number generator seed to support testing
public void setSeed(int seed) {
rand.setSeed(seed);
}
// Allows dice to be compared if necessary
public int compareTo(GVDie d) {
return getValue() - d.getValue();
}
}
![1 import java.util.Scanner;
2 public class LabProgram {
3 public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
+567 ∞ N34567
LLLLLL
4
8
9
10
11
12
13
14
15
16
17 }
}
Current file: LabProgram.java
int credits;
int seed;
GVDie diel, die2;
diel new GVDie();
die2 = new GVDie();
// Read random seed to support testing (do not alter)
seed scnr.nextInt();
=
diel.setSeed (seed);
// Read starting credits
credits = scnr.nextInt ();
/* Insert your code here */](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2Fa7810c71-94a2-4fcd-850d-4bdb577daa08%2Fttym4j1_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

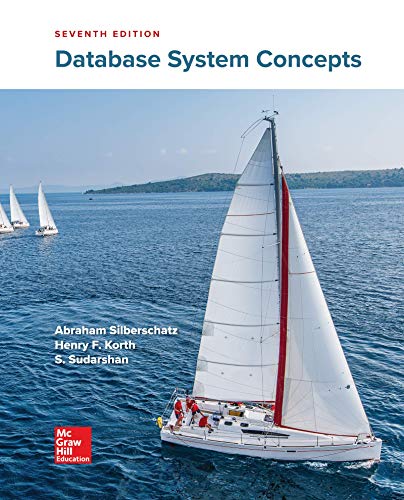
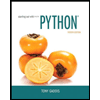
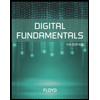
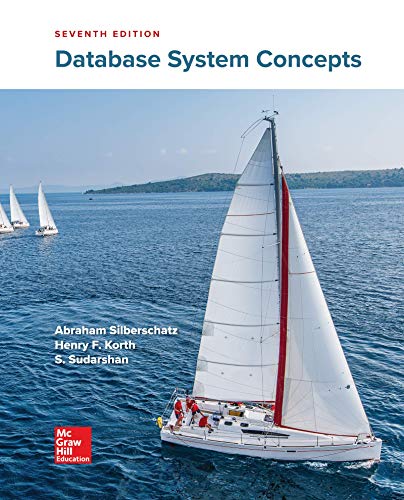
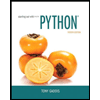
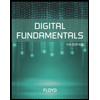
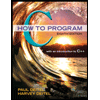
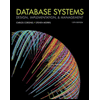
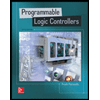