Problem Description Peter Pan needs a reliable translator to encode messages to evade his nosy pirate adversaries, and he needs your help! You will implement a translation system that can handle his diverse linguistic needs. To do that, you will write three classes (the name of each is bolded below). The Message class is at the core of the translation system. A Message stores a message and the dialect it's encoded into. A Dialect enum defines all supported dialects, where each dialect has its own set of rules governing the translation of messages. Additionally, the Message class maintains a running count of how many messages have been translated to each dialect at any point in time. Message exposes a public translate method that translates the original message into its specified dialect. It defines other private methods that handle the translation logic for each specific dialect. Note that a Message should not be considered translated until the first call to the translate method on that object. Message also exposes a method that returns the counts of messages translated into each dialect across all instances.
Problem Description Peter Pan needs a reliable translator to encode messages to evade his nosy pirate adversaries, and he needs your help! You will implement a translation system that can handle his diverse linguistic needs. To do that, you will write three classes (the name of each is bolded below). The Message class is at the core of the translation system. A Message stores a message and the dialect it's encoded into. A Dialect enum defines all supported dialects, where each dialect has its own set of rules governing the translation of messages. Additionally, the Message class maintains a running count of how many messages have been translated to each dialect at any point in time. Message exposes a public translate method that translates the original message into its specified dialect. It defines other private methods that handle the translation logic for each specific dialect. Note that a Message should not be considered translated until the first call to the translate method on that object. Message also exposes a method that returns the counts of messages translated into each dialect across all instances.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question

Transcribed Image Text:Problem Description
Peter Pan needs a reliable translator to encode messages to evade his nosy pirate adversaries, and he
needs your help! You will implement a translation system that can handle his diverse linguistic needs. To
do that, you will write three classes (the name of each is bolded below).
The Message class is at the core of the translation system. A Message stores a message and the
dialect it's encoded into. A Dialect enum defines all supported dialects, where each dialect has its
own set of rules governing the translation of messages. Additionally, the Message class maintains a
running count of how many messages have been translated to each dialect at any point in time.
Message exposes a public translate method that translates the original message into its specified
dialect. It defines other private methods that handle the translation logic for each specific dialect. Note
that a Message should not be considered translated until the first call to the translate method on that
object.
Message also exposes a method that returns the counts of messages translated into each dialect
across all instances.
The Peter Pan class is the driver program for the translation system. It's responsible for creating
messages with various dialects and displaying the translations along with other information. It enables
live input from the user for message content and dialect selection, offering a more engaging and
interactive experience.
Lastly, for this homework and all future ones, we will require you to Javadoc your code. The Checkstyle
section toward the end of the document discusses this some.

Transcribed Image Text:Solution Description
In the interest of encapsulation, data members for each of the following classes should only be
accessible from other classes using getters and/or setters where needed. The described methods and
constructors for this assignment should be public. It is OK to write private helper methods for your
classes, however.
Your solution will consist of three files:
Dialect.java
Message.java
Peter Pan.java
Dialect.java
Defines an enum that enumerates three dialects: PIRATE, ELMER_FUDD and COMPUTER.
Message.java
The Message class is the central component of the translation system, managing individual messages
by encapsulating their dialect and content. To instantiate a message, a client must provide the dialect
and original string message (in that order). Message should include methods to translate messages
into each of its three supported dialects respectively, while allowing the user only to interface with it
through the general translate method. (Think about what that means.)
A Message has the following attributes:
1. A dialect
2. The message (two versions)
a. Holds the original, unmodified message before translate has been called
b. Holds the translated, modified message once translate has been called
3. A true/false value specifying whether translate has been called yet for this message
Further, the Message class will track the values below across all instances:
1. The count of messages translated into Elmer Fudd dialect
2. The count of messages translated into Pirate dialect
3. The count of messages translated into Computer dialect
Each of the counts will start at 0.
You should initialize all instance variables in the constructor (consider hat value the true/false flag
should initially assume).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
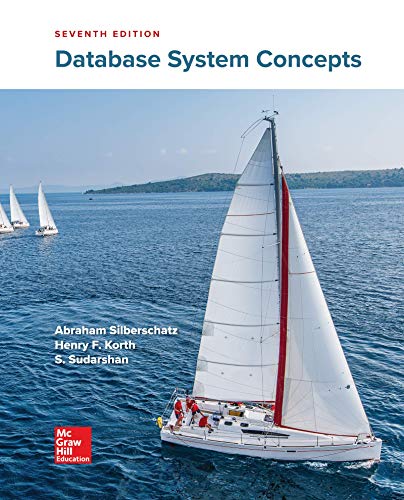
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
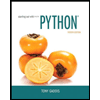
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
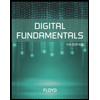
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
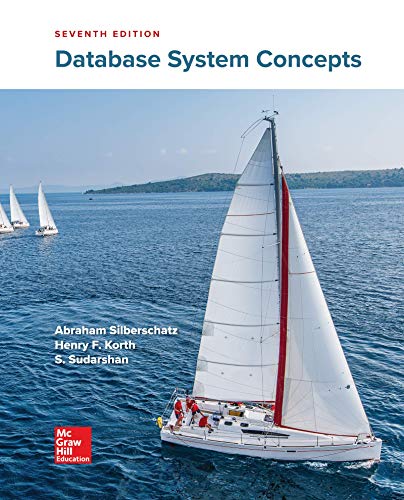
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
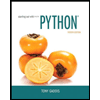
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
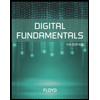
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
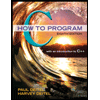
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
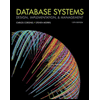
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
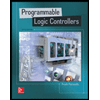
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education