Problem Description MUST BE WRITTEN IN C++ A retail company must file a monthly sales tax report listing the total sales for the month and the amount of state and county sales tax collected. The state sales tax rate is 4 percent, and the county sales tax rate is 2 percent. Write a program that asks the user to enter the total sales for the month. The application should calculate and display the following: The amount of county sales tax The amount of state sales tax The total sales tax (county plus state) Problem Algorithm Get the total sales for the month. Multiply the total sales by .04 to calculate the state sales tax. Multiply the total sales by .02 to calculate the county sales tax. Add the state tax and county tax to calculate the total sales tax. Display the calculated county tax, state tax, and total sales tax. Solutions This program is most easily solved using just four variables. Declare the variables that you will need in the program, using the proper data type and documenting the purpose. Variable Name Purpose Declare Real totalSales Stores total sales the user inputs Given the problem for this program, what modules might you consider including? Provide additional module names and describe the purpose of each module. Module Name Purpose Module inputData() Allows the user to enter required user input Write the pseudocode. Also, when writing your modules and making calls, be sure to pass necessary variables as arguments and accept them as reference parameters if they need to be modified in the module. //Pseudocode goes here Translate your pseudocode from the previous section to actual code using C++. Read the following program prior to completing the lab. Call your C++ file, “monthly_sales_report.cpp”. Consider the following C++ functions for your program: main that calls your other functions inputData that will ask for the monthly sales calcCounty that will calculate the county tax calcState that will calculate the state tax calcTotal that will calculate the total tax printData that will display the county tax, the state tax, and the total tax If your program is correct, its output should look as follows (you might get fewer than 2, or more than 2 digits after the decimal point; this is okay): Welcome to the total tax calculator program Enter the total sales for the month: 12567 The county tax is 251.34 The state tax is 502.68 The total tax is 754.02
Problem Description
MUST BE WRITTEN IN C++
A retail company must file a monthly sales tax report listing the total sales for the month and the amount of state and county sales tax collected. The state sales tax rate is 4 percent, and the county sales tax rate is 2 percent. Write a
- The amount of county sales tax
- The amount of state sales tax
- The total sales tax (county plus state)
Problem Algorithm
- Get the total sales for the month.
- Multiply the total sales by .04 to calculate the state sales tax.
- Multiply the total sales by .02 to calculate the county sales tax.
- Add the state tax and county tax to calculate the total sales tax.
- Display the calculated county tax, state tax, and total sales tax.
Solutions
- This program is most easily solved using just four variables. Declare the variables that you will need in the program, using the proper data type and documenting the purpose.
Variable Name |
Purpose |
Declare Real totalSales |
Stores total sales the user inputs |
|
|
|
|
|
|
- Given the problem for this program, what modules might you consider including? Provide additional module names and describe the purpose of each module.
Module Name |
Purpose |
Module inputData() |
Allows the user to enter required user input |
|
|
|
|
|
|
|
|
Write the pseudocode. Also, when writing your modules and making calls, be sure to pass necessary variables as arguments and accept them as reference parameters if they need to be modified in the module.
//Pseudocode goes here
|
- Translate your pseudocode from the previous section to actual code using C++. Read the following program prior to completing the lab. Call your C++ file, “monthly_sales_report.cpp”.
Consider the following C++ functions for your program:
- main that calls your other functions
- inputData that will ask for the monthly sales
- calcCounty that will calculate the county tax
- calcState that will calculate the state tax
- calcTotal that will calculate the total tax
- printData that will display the county tax, the state tax, and the total tax
If your program is correct, its output should look as follows (you might get fewer than 2, or more than 2 digits after the decimal point; this is okay):
Welcome to the total tax calculator program
Enter the total sales for the month: 12567<Enter>
The county tax is 251.34
The state tax is 502.68
The total tax is 754.02

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

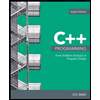
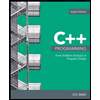