Postfix interpreter def postfix_evaluate(items): When arithmetic expressions are given in the familiar infix notation 2 + 3 * 4, we need to use parentheses to force a different evaluation order than the usual PEMDAS order determined by precedence and associativity. Writing arithmetic expressions in postfix notation (also known as Reverse Polish Notation) may look strange to us humans accustomed to the conventional infix notation, but is computationally far easier to handle, since postfix notation allows any evaluation order to be expressed unambiguously without using any parentheses at all! A postfix expression is given as a list of items that can be either individual integers or one of the strings '+', '-', '*' and '/' for the four possible arithmetic operators. To evaluate a postfix expression using a simple linear loop, use a list as a stack that is initially empty. Loop through the items one by one, in order from left to right. Whenever the current item is an integer, just append it to the end of the list. Whenever the current item is one of the four arithmetic operations, pop two items from the end of the list, perform that operation on those items, and append the result to the list. Assuming that items is a legal postfix expression, which is guaranteed in this problem so that you don't need to do any error handling, once all items have been processed this way, the one number that remains in the stack is returned as the final answer. To avoid the intricacies of floating point arithmetic, you should perform the division operation using the Python integer division operator // that truncates the result to the integer part. Furthermore, to avoid the crash caused by dividing by zero, in this problem we shall artificially make up a rule that dividing anything by zero will simply evaluate to zero instead of crashing. (By adding more operators and another auxiliary stack, an entire Turing-complete programming language can be built around postfix evaluation. See the Wikipedia page "Forth", if interested.)
Postfix interpreter
def postfix_evaluate(items):
When arithmetic expressions are given in the familiar infix notation 2 + 3 * 4, we need to use parentheses to force a different evaluation order than the usual PEMDAS order determined by precedence and associativity. Writing arithmetic expressions in postfix notation (also known as
Reverse Polish Notation) may look strange to us humans accustomed to the conventional infix notation, but is computationally far easier to handle, since postfix notation allows any evaluation order to be expressed unambiguously without using any parentheses at all! A postfix expression is
given as a list of items that can be either individual integers or one of the strings '+', '-', '*' and '/' for the four possible arithmetic operators.
To evaluate a postfix expression using a simple linear loop, use a list as a stack that is initially empty. Loop through the items one by one, in order from left to right. Whenever the current item is an integer, just append it to the end of the list. Whenever the current item is one of the four
arithmetic operations, pop two items from the end of the list, perform that operation on those items, and append the result to the list. Assuming that items is a legal postfix expression, which is guaranteed in this problem so that you don't need to do any error handling, once all items have been
processed this way, the one number that remains in the stack is returned as the final answer.
To avoid the intricacies of floating point arithmetic, you should perform the division operation using the Python integer division operator // that truncates the result to the integer part. Furthermore, to avoid the crash caused by dividing by zero, in this problem we shall artificially make up a rule that dividing anything by zero will simply evaluate to zero instead of crashing.
(By adding more operators and another auxiliary stack, an entire Turing-complete
![items
(Equivalent infix) Expected result
[2, 3, '+', 4,
'*' ]
(2+3) * 4
20
[2, 3, 4, '*', '+']
2 + (3*4)
14
[3, 3, 3, '-', '/']
3/ (3 - 3)
[7, 3, '/']
7 / 3
2
'*',
1 * 2 * 3 * 4 * 5 * 6 | 720
[1, 2, 3, 4, 5, 6,
'* ' ]
'*',
*](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F316af9c1-5440-42b3-bd44-a1bdda94a38d%2F9c16cb8a-6278-4046-8554-87fdb8808c85%2Flvrp1fs_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

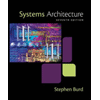
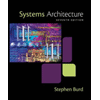