plz redo this code in C using system functionsinstead of the standard functions i have: #include #include int main(int argc, char const *argv[]) { // if entered file list is not sufficient if (argc < 3) { printf("Enter required file names\n"); exit(0); } // last filename entered would be the resultant file FILE *resultFile = fopen(argv[argc - 1], "w"), *f; // checking if file opening is successful or not if (resultFile == NULL) { printf("Error opening file %s\n", argv[argc - 1]); exit(1); } // buffer to read the files line by line char buff[1000]; // loop to read the entered files for (int i = 1; i < argc - 1; ++i) { // opening the file f = fopen(argv[i], "r"); // checking if it opened or not if (f == NULL) { printf("Error opening file %s\n", argv[i]); exit(1); } // reading the file line by line and appending them to the resultFile while (fgets(buff, 1000, f)) { fprintf(resultFile, "%s", buff); } // closing current file fclose(f); } // closing resultFile fclose(resultFile); return 0; }
plz redo this code in C using system functionsinstead of the standard functions i have:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char const *argv[]) {
// if entered file list is not sufficient
if (argc < 3) {
printf("Enter required file names\n");
exit(0);
}
// last filename entered would be the resultant file
FILE *resultFile = fopen(argv[argc - 1], "w"), *f;
// checking if file opening is successful or not
if (resultFile == NULL) {
printf("Error opening file %s\n", argv[argc - 1]);
exit(1);
}
// buffer to read the files line by line
char buff[1000];
// loop to read the entered files
for (int i = 1; i < argc - 1; ++i) {
// opening the file
f = fopen(argv[i], "r");
// checking if it opened or not
if (f == NULL) {
printf("Error opening file %s\n", argv[i]);
exit(1);
}
// reading the file line by line and appending them to the resultFile
while (fgets(buff, 1000, f)) {
fprintf(resultFile, "%s", buff);
}
// closing current file
fclose(f);
}
// closing resultFile
fclose(resultFile);
return 0;
}

Step by step
Solved in 2 steps with 1 images

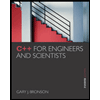
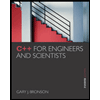