Please
Please use C++ to complete Artist.h, Artist.cpp, Artwork.h, and Artwork.cpp files. Main.cpp cannot be changed.
Artist.h Given:
#ifndef ARTISTH
#define ARTISTH
#include <string>
using namespace std;
class Artist{
public:
Artist();
Artist(string artistName, int birthYear, int deathYear);
string GetName() const;
int GetBirthYear() const;
int GetDeathYear() const;
void PrintInfo() const;
private:
// TODO: Declare private data members - artistName, birthYear, deathYear
};
#endif
Artist.cpp Given:
#include "Artist.h"
#include <iostream>
#include <string>
using namespace std;
// TODO: Define default constructor
// TODO: Define second constructor to initialize
// private fields (artistName, birthYear, deathYear)
// TODO: Define get functions: GetName(), GetBirthYear(), GetDeathYear()
// TODO: Define PrintInfo() function
// If deathYear is entered as -1, only print birthYear
Artwork.h Given:
#ifndef ARTWORKH
#define ARTWORKH
#include "Artist.h"
class Artwork{
public:
Artwork();
Artwork(string title, int yearCreated, Artist artist);
string GetTitle();
int GetYearCreated();
void PrintInfo();
private:
// TODO: Declare private data members - title, yearCreated
// TODO: Declare private data member artist of type Artist
};
#endif
Artwork.cpp Given:
#include "Artwork.h"
#include <iostream>
// TODO: Define default constructor
// TODO: Define second constructor to initialize
// private fields (title, yearCreated, artist)
// TODO: Define get functions: GetTitle(), GetYearCreated()
// TODO: Define PrintInfo() function
// Call the PrintInfo() function in the Artist class to print an artist's information



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

I typed your code in exactly as it was typed in your answer and I am getting a lot of errors.
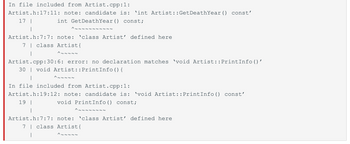
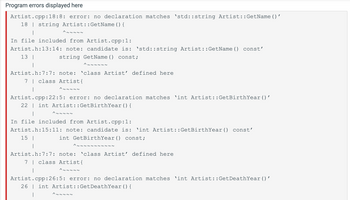
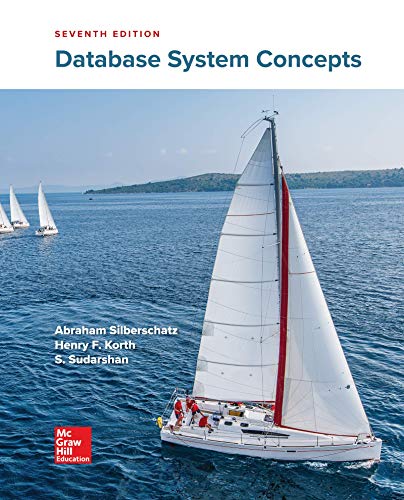
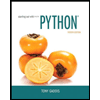
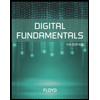
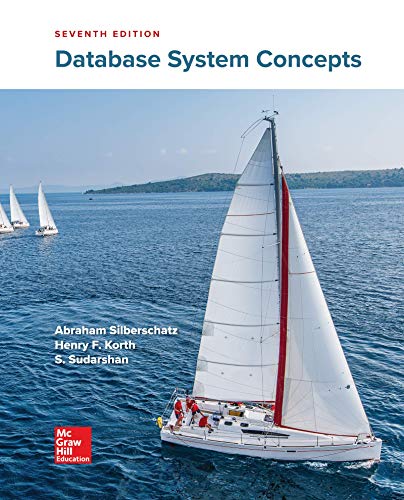
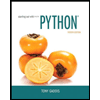
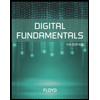
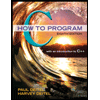
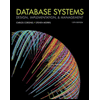
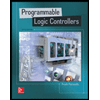