Please write in your own original code. Example output is attached Objective Create a class hierarchy by defining an abstract Shape class and concrete classes pertaining to various shapes: Circle, Sphere, Cylinder, Square, Cube, Triangle and Tetrahedron. Related SLO Develop properly structured multifile programs with automatic compilation. Use classes, polymorphism and inheritance in C++. Instructions Download the following starter file: https://laulima.hawaii.edu/x/6rw8eB Right-click on the link above > Save link as Rename LastnameFirstname22.cpp so that Lastname is your own last name and Firstname is your own first name. Create a makefile named makefile that will automatically compile, link, and create an executable for this assignment. IMPORTANT: Be sure to use g++ as the compiler NOT gcc as we are now programming in C++. Example makefile using g++ here There are no extra .h or .cpp needed for this assignment. Do NOT submit a makefile with any other name. The starter file contains code for an abstract class definition named Shape, a derived class definition named Circle, main() driver program, and example output. Here is what you need to do: From the abstract Shape class, derive concrete classes named Square and Triangle. From class Circle, derive concrete classes Sphere and Cylinder. From class Square, derive concrete class Cube. From class Triangle, derive concrete class Tetrahedron. Here is a visual representation of the class hierarchy: Shape / | \ / Square \ / | \ / Cube Triangle Circle \ / \ Tetrahedron / \ Sphere Cylinder The abstract Shape class defines the following virtual functions: virtual char* name() const - returns the name of the class virtual void printDetails() const - display the area, or surface area & volume of the object virtual void inputData() - ask the user for the radius, side, and/or height of the object and set the appropriate data member(s) virtual double area() const - returns the area or surface area of the object virtual double volume() const - returns the volume of the object For each derived class, implement all applicable virtual functions. Applicable meaning not all virtual functions will appear in all derived classes. For instance, Circle, Square, and Triangle will not have volume. Any data members that will be used in a child class should be declared as protected, for all other data members, use private. Error checking and correction is not required for the data members. After defining a class, do two things: Uncomment the line in main() for the corresponding class Increase the value of the NUM symbolic constant by 1 The shape will be added to the menu array and the corresponding menu option will be shown when the program runs. Work in steps and define one class at a time and it ensure it works before moving on to the next. The calculations associated with each derived shape are as follows: These also give some indication of which virtual functions are implemented in each class. π = 3.14 r = radius s = side Circle circle's area = πr2 Sphere sphere's surface area = 4πr2 sphere's volume = (4πr3) / 3 Cylinder cylinder's surface area = 2πrh + 2πr2 cylinder's volume = πr2h Square square's area = s2 Cube cube's surface area = 6s2 cube's volume = s3 Triangle equilateral triangle's area = s2 * sqrt(3)/4 Tetrahedron regular tetrahedron's surface area = s2 * sqrt(3) regular tetrahedron's volume = s3 * sqrt(2)/12 For square root, use the sqrt() function, the starter file already has the necessary include. Be sure to have the usual program header at the top that contains the program description, author information, etc. Comments are not required for this assignment other than the description at the top. NOTE: The main error students make with this assignment is to repeat data unnecessarily. The point of inheritance is to be a "lazy" programmer and not re-invent the wheel. For example, if the base class Circle has a radius protected data member, the derived class Sphere does NOT need a radius because it already inherited radius from Circle. Keep this concept in mind when writing your code.
Please write in your own original code. Example output is attached Objective Create a class hierarchy by defining an abstract Shape class and concrete classes pertaining to various shapes: Circle, Sphere, Cylinder, Square, Cube, Triangle and Tetrahedron. Related SLO Develop properly structured multifile programs with automatic compilation. Use classes, polymorphism and inheritance in C++. Instructions Download the following starter file: https://laulima.hawaii.edu/x/6rw8eB Right-click on the link above > Save link as Rename LastnameFirstname22.cpp so that Lastname is your own last name and Firstname is your own first name. Create a makefile named makefile that will automatically compile, link, and create an executable for this assignment. IMPORTANT: Be sure to use g++ as the compiler NOT gcc as we are now programming in C++. Example makefile using g++ here There are no extra .h or .cpp needed for this assignment. Do NOT submit a makefile with any other name. The starter file contains code for an abstract class definition named Shape, a derived class definition named Circle, main() driver program, and example output. Here is what you need to do: From the abstract Shape class, derive concrete classes named Square and Triangle. From class Circle, derive concrete classes Sphere and Cylinder. From class Square, derive concrete class Cube. From class Triangle, derive concrete class Tetrahedron. Here is a visual representation of the class hierarchy: Shape / | \ / Square \ / | \ / Cube Triangle Circle \ / \ Tetrahedron / \ Sphere Cylinder The abstract Shape class defines the following virtual functions: virtual char* name() const - returns the name of the class virtual void printDetails() const - display the area, or surface area & volume of the object virtual void inputData() - ask the user for the radius, side, and/or height of the object and set the appropriate data member(s) virtual double area() const - returns the area or surface area of the object virtual double volume() const - returns the volume of the object For each derived class, implement all applicable virtual functions. Applicable meaning not all virtual functions will appear in all derived classes. For instance, Circle, Square, and Triangle will not have volume. Any data members that will be used in a child class should be declared as protected, for all other data members, use private. Error checking and correction is not required for the data members. After defining a class, do two things: Uncomment the line in main() for the corresponding class Increase the value of the NUM symbolic constant by 1 The shape will be added to the menu array and the corresponding menu option will be shown when the program runs. Work in steps and define one class at a time and it ensure it works before moving on to the next. The calculations associated with each derived shape are as follows: These also give some indication of which virtual functions are implemented in each class. π = 3.14 r = radius s = side Circle circle's area = πr2 Sphere sphere's surface area = 4πr2 sphere's volume = (4πr3) / 3 Cylinder cylinder's surface area = 2πrh + 2πr2 cylinder's volume = πr2h Square square's area = s2 Cube cube's surface area = 6s2 cube's volume = s3 Triangle equilateral triangle's area = s2 * sqrt(3)/4 Tetrahedron regular tetrahedron's surface area = s2 * sqrt(3) regular tetrahedron's volume = s3 * sqrt(2)/12 For square root, use the sqrt() function, the starter file already has the necessary include. Be sure to have the usual program header at the top that contains the program description, author information, etc. Comments are not required for this assignment other than the description at the top. NOTE: The main error students make with this assignment is to repeat data unnecessarily. The point of inheritance is to be a "lazy" programmer and not re-invent the wheel. For example, if the base class Circle has a radius protected data member, the derived class Sphere does NOT need a radius because it already inherited radius from Circle. Keep this concept in mind when writing your code.
Chapter4: Making Decisions
Section: Chapter Questions
Problem 8E: In the game Rock paper Scissors, two players simultaneously choose one of three options: rock,...
Related questions
Concept explainers
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Question
- Please write in your own original code. Example output is attached
- Objective
- Create a class hierarchy by defining an abstract Shape class and concrete classes pertaining to various shapes: Circle, Sphere, Cylinder, Square, Cube, Triangle and Tetrahedron.
- Related SLO
- Develop properly structured multifile programs with automatic compilation.
- Use classes, polymorphism and inheritance in C++.
- Instructions
- Download the following starter file: https://laulima.hawaii.edu/x/6rw8eB
- Right-click on the link above > Save link as
- Rename LastnameFirstname22.cpp so that Lastname is your own last name and Firstname is your own first name.
- Create a makefile named makefile that will automatically compile, link, and create an executable for this assignment.
- IMPORTANT: Be sure to use g++ as the compiler NOT gcc as we are now programming in C++.
- Example makefile using g++ here
- There are no extra .h or .cpp needed for this assignment.
- Do NOT submit a makefile with any other name.
- The starter file contains code for an abstract class definition named Shape, a derived class definition named Circle, main() driver program, and example output.
- Here is what you need to do:
- From the abstract Shape class, derive concrete classes named Square and Triangle.
- From class Circle, derive concrete classes Sphere and Cylinder.
- From class Square, derive concrete class Cube.
- From class Triangle, derive concrete class Tetrahedron.
- Here is a visual representation of the class hierarchy: Shape / | \ / Square \ / | \ / Cube Triangle Circle \ / \ Tetrahedron / \ Sphere Cylinder
- The abstract Shape class defines the following virtual functions:
- virtual char* name() const - returns the name of the class
- virtual void printDetails() const - display the area, or surface area & volume of the object
- virtual void inputData() - ask the user for the radius, side, and/or height of the object and set the appropriate data member(s)
- virtual double area() const - returns the area or surface area of the object
- virtual double volume() const - returns the volume of the object
- For each derived class, implement all applicable virtual functions. Applicable meaning not all virtual functions will appear in all derived classes. For instance, Circle, Square, and Triangle will not have volume.
- Any data members that will be used in a child class should be declared as protected, for all other data members, use private.
- Error checking and correction is not required for the data members.
- After defining a class, do two things:
- Uncomment the line in main() for the corresponding class
- Increase the value of the NUM symbolic constant by 1
- Work in steps and define one class at a time and it ensure it works before moving on to the next.
- The calculations associated with each derived shape are as follows:
These also give some indication of which virtual functions are implemented in each class. - π = 3.14
- r = radius
- s = side
- Circle
- circle's area = πr2
- Sphere
- sphere's surface area = 4πr2
- sphere's volume = (4πr3) / 3
- Cylinder
- cylinder's surface area = 2πrh + 2πr2
- cylinder's volume = πr2h
- Square
- square's area = s2
- Cube
- cube's surface area = 6s2
- cube's volume = s3
- Triangle
- equilateral triangle's area = s2 * sqrt(3)/4
- Tetrahedron
- regular tetrahedron's surface area = s2 * sqrt(3)
- regular tetrahedron's volume = s3 * sqrt(2)/12
- For square root, use the sqrt() function, the starter file already has the necessary include.
- Be sure to have the usual program header at the top that contains the program description, author information, etc.
Comments are not required for this assignment other than the description at the top. - NOTE: The main error students make with this assignment is to repeat data unnecessarily. The point of inheritance is to be a "lazy" programmer and not re-invent the wheel. For example, if the base class Circle has a radius protected data member, the derived class Sphere does NOT need a radius because it already inherited radius from Circle. Keep this concept in mind when writing your code.

Transcribed Image Text:Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
0
Enter the Circle's radius: 10
The Circle's area = 314
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
1
Enter the Sphere's radius: 20
The Sphere's surface area = 5024
The Sphere's volume = 33493.3
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
2
Enter the Cylinder's radius: 30
Enter the Cylinder's height: 40
The Cylinder's surface area = 13188
The Cylinder's volume = 113040
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
3
Enter the Square's side: 50
The Square's area = 2500
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron

Transcribed Image Text:4
Enter the Cube's side: 60
The Cube's surface area = 21600
The Cube's volume = 216000
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
5
Enter the Triangle's side: 70
The Triangle's area = 2121.76
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
6
Enter the Tetrahedron's side: 80
The Tetrahedron's surface area = 11085.1
The Tetrahedron's volume = 60339.8
Select an object from the menu (enter 7 to quit).
0. Circle
1. Sphere
2. Cylinder
3. Square
4. Cube
5. Triangle
6. Tetrahedron
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
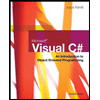
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
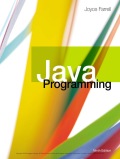
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
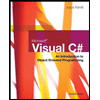
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
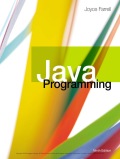
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT