Please read the instructions crefelly this is a java code programming please solve it. don plagarise or copy from other sources please. Please do what in the question says .There are previous lab codes needed. So, I am providing the codes- Apartment classes from Lab 17 code - public class Apartment extends Building { private int totalUnits; public Apartment (String name, CanadianAddress address, int squareFootage, int totalUnits) { super (name, address, squareFootage); this.totalUnits = totalUnits; } public int getTotalUnits () { return totalUnits; } public void setTotalUnits (int totalUnits) { this.totalUnits = totalUnits; } } CanadianAddress class from Lab 18 code - public class TestCanadianAddresslab18 { public static void main(String[] args) { CanadianAddress testAddress = new CanadianAddress(); CanadianAddress SMUAddress = new CanadianAddress("923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3"); System.out.println("The testAddress is created as:"); System.out.println(testAddress); System.out.println(); System.out.println("The SMUAddress is created as:"); System.out.println(SMUAddress); System.out.println(); System.out.println("The testAddress is equal to SMUAddress? : " + testAddress.equals(SMUAddress)); System.out.println(); testAddress.setStreetAddress("923 Robie St."); testAddress.setCity("Halifax"); testAddress.setProvince("Nova Scotia"); testAddress.setPostalCode("B3H 3C3"); System.out.println("The testAddress is updated as: "); System.out.println(testAddress); System.out.println(); System.out.println("The testAddress is equal to SMUAddress? : " + testAddress.equals(SMUAddress)); } } class CanadianAddress { private String streetAddress; private String city; private String province; private String postalCode; public CanadianAddress() {} public CanadianAddress(String streetAddress, String city, String province, String postalCode) { this.streetAddress = streetAddress; this.city = city; this.province = province; this.postalCode = postalCode; } public String getStreetAddress() { return streetAddress; } public void setStreetAddress(String streetAddress) { this.streetAddress = streetAddress; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } public String getProvince() { return province; } public void setProvince(String province) { this.province = province; } public String getPostalCode() { return postalCode; } public void setPostalCode(String postalCode) { this.postalCode = postalCode; } @Override public String toString() { return (streetAddress != null ? streetAddress : "null") + ", " + (city != null ? city : "null") + "\n" + (province != null ? province : "null") + " " + (postalCode != null ? postalCode : "null") + ", Canada"; } @Override public boolean equals(Object address) { if (this == address) return true; if (address == null || getClass() != address.getClass()) return false; CanadianAddress that = (CanadianAddress) address; return (this.streetAddress == null ? that.streetAddress == null : this.streetAddress.equals(that.streetAddress)) && (this.city == null ? that.city == null : this.city.equals(that.city)) && (this.province == null ? that.province == null : this.province.equals(that.province)) && (this.postalCode == null ? that.postalCode == null : this.postalCode.equals(that.postalCode)); } }
Please read the instructions crefelly this is a java code programming please solve it. don plagarise or copy from other sources please. Please do what in the question says .There are previous lab codes needed. So, I am providing the codes-
Apartment classes from Lab 17 code -
CanadianAddress class from Lab 18 code -
public class TestCanadianAddresslab18 {
public static void main(String[] args) {
CanadianAddress testAddress = new CanadianAddress();
CanadianAddress SMUAddress = new CanadianAddress("923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3");
System.out.println("The testAddress is created as:");
System.out.println(testAddress);
System.out.println();
System.out.println("The SMUAddress is created as:");
System.out.println(SMUAddress);
System.out.println();
System.out.println("The testAddress is equal to SMUAddress? : " + testAddress.equals(SMUAddress));
System.out.println();
testAddress.setStreetAddress("923 Robie St.");
testAddress.setCity("Halifax");
testAddress.setProvince("Nova Scotia");
testAddress.setPostalCode("B3H 3C3");
System.out.println("The testAddress is updated as: ");
System.out.println(testAddress);
System.out.println();
System.out.println("The testAddress is equal to SMUAddress? : " + testAddress.equals(SMUAddress));
}
}
class CanadianAddress {
private String streetAddress;
private String city;
private String province;
private String postalCode;
public CanadianAddress() {}
public CanadianAddress(String streetAddress, String city, String province, String postalCode) {
this.streetAddress = streetAddress;
this.city = city;
this.province = province;
this.postalCode = postalCode;
}
public String getStreetAddress() {
return streetAddress;
}
public void setStreetAddress(String streetAddress) {
this.streetAddress = streetAddress;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getProvince() {
return province;
}
public void setProvince(String province) {
this.province = province;
}
public String getPostalCode() {
return postalCode;
}
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
@Override
public String toString() {
return (streetAddress != null ? streetAddress : "null") + ", " +
(city != null ? city : "null") + "\n" +
(province != null ? province : "null") + " " +
(postalCode != null ? postalCode : "null") + ", Canada";
}
@Override
public boolean equals(Object address) {
if (this == address) return true;
if (address == null || getClass() != address.getClass()) return false;
CanadianAddress that = (CanadianAddress) address;
return (this.streetAddress == null ? that.streetAddress == null : this.streetAddress.equals(that.streetAddress))
&& (this.city == null ? that.city == null : this.city.equals(that.city))
&& (this.province == null ? that.province == null : this.province.equals(that.province))
&& (this.postalCode == null ? that.postalCode == null : this.postalCode.equals(that.postalCode));
}
}
![Instructions
Task: Polymorphism (The Building class and its subclasses)
This task practices polymorphism. You will extend and modify the Building and
Apartment classes from Lab 17. Save a copy of their previous implementation if you
need it. You will also use the new CanadianAddress class from Lab 18. Make sure it is
accessible with proper visibility modifiers and package settings.
Add to the Building class:
• Override the toString() method inherited from the Object class, returning a
string in the following format (you may copy the blue highlighted part): "A
Building object:\nName: " + name + "\nAddress:\n" + address + "\nSquare
footage: " + square Footage + "\n". Use the @Override annotation to make sure
you override the toString() method correctly.
Add to the Apartment class:
Override the toString() method inherited from the Object class, returning a
string in the following format (you may copy the blue highlighted part): "An
Apartment object:\nName: " + this.getName() + "\nAddress:\n" +
this.getAddress() + "\nSquare footage: " + this.getSquareFootage() + "\nTotal
units: " + totalUnits + "\n". Use the @Override annotation to make sure you
override the toString() method correctly.
Write a Mall class that extends the Building class and contains:
.
A private String[] data field named stores that contains a list of store names in
the mall. You do not need to create the array or initialize the array reference
variable when declaring this data field. These will be done in the constructor.
You can just declare the array reference variable. You must use the given name
for the data field.
• A constructor that accepts the following four parameters: a reference to a
String object named name, a reference to a CanadianAddress object named
address, an int named squareFootage, and a reference to a String[] array
named stores. You must use the given parameter names. The first three
parameters are to be passed to the superclass's constructor.
• Getter and setter methods for the data field stores.
• Override the toString() method inherited from the Object class. Firstly, create a
String named storeList that concatenating all the store names in the data field
stores, separating the store names by a comma and a space", ". Secondly,
return a string in the following format (you may copy the blue highlighted
part): "A Mall object: \nName: " + this.getName() + "\nAddress:\n" +
this.getAddress() + "\nSquare footage: " + this.getSquareFootage() + "\nStores:
+ storeList. Use the @Override annotation to make sure you override the
toString() method correctly.
So far, you have three classes Building, Apartment, and Mall. Each class has its own
version of the toString() method which returns a String representation of the object,
including the object type. The object type is used to help us distinguish which
toString() method is invoked and see how polymorphism works.
Write a test class named TestPolymorphismWithBuilding that performs the
followings in the given order:
• Create a Building object:
1. Create a CanadianAddress object named address1 with the empty
address, using the no-arg constructor.
2. Create a Building object with the building name "my empty building",
address1, and the square footage 0.
3. Assign the above object to a Building type reference variable named
building.
• Create an Apartment object and assign its reference to a Building reference
variable:
1. Create a CanadianAddress object named address2 for the following
address: 1030 South Park St., Halifax,
Nova Scotia B3H 2W3, Canada
2. Create an Apartment object with the building name "Somerset Place
Apartment", address2, the square footage 100000, and total units 100.
3. Assign the above object to a Building type reference variable
named apartment. Note the reference variable should be declared as
Building type.
• Create a Mall object and assign its reference to a Building reference variable:
1. Create a CanadianAddress object named address3 for the following
address: 7001 Mumford Rd., Halifax,
Nova Scotia B3L 4R3, Canada
2. Create a String[] array named stores that contains the following strings:
"Aldo", "American Eagle", and "Apple".
3. Create a Mall object with the building name "Halifax Shopping Centre",
address3, the square footage 200000, and stores.
4. Assign the above object to a Building type reference variable
named mall. Note the reference variable should be declared as Building
type.
• Print out the three objects building, apartment, and mall using:
System.out.println(building);
System.out.println(apartment);
System.out.println(mall);
• Observe that the toString() methods are invoked according to the actual types
of the referenced objects, not the declared type of the reference variables.
Additional requirement:
Put all the classes in separate files. You should have the following files (all files should
be included in an online submission):
•
CanadianAddress.java: from Lab 18, unchanged
•
Building.java: from Lab 17, modified
Apartment.java: from Lab 17, modified
• Mall.java: created in this lab
•
TestPolymorphismWithBuilding.java: created in this lab.
A sample run is as follows:
A Building object:
Name: my empty building
Address:
null, null,
null null, Canada
Square footage: 0
An Apartment object:
Name: Somerset Place Apartment
Address:
1030 South Park St., Halifax,
Nova Scotia B3H 2W3, Canada
Square footage: 100000
Total units: 100
A Mall object:
Name: Halifax Shopping Center
Address:
7001 Mumford Rd., Halifax,
Nova Scotia B3L 4R3, Canada
Square footage: 200000
Stores: Aldo, American Eagle, Apple](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F83cebb0c-db38-4f0e-a0d3-5fd209e24564%2F8f7e9f25-c8e2-4aac-b993-ff918a862c46%2Fujt14bv_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 6 images

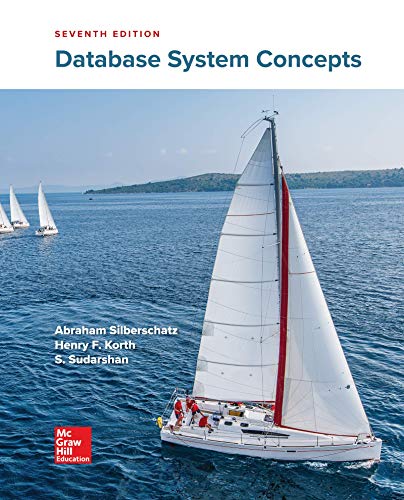
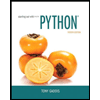
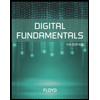
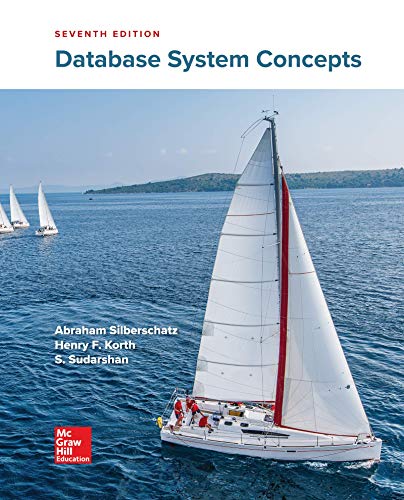
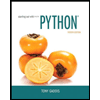
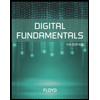
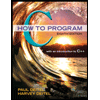
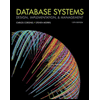
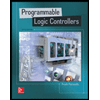