Please help fix the following errors in red for the code below. (The .h file is not listed due to maximum character count) Bstree.cpp: using namespace std; #include "Bstree.h" /* Nested Node class definitions */ template template Bstree::Node::Node(T item) { data = item; left = nullptr; right = nullptr; } /* Outer Bstree class definitions */ template Bstree::Bstree() { root = nullptr; order = 0; } template Bstree::~Bstree() { recDestroy(root); } template bool Bstree::empty() const { return root == nullptr; } template void Bstree::insert(T item) { Node* tmp; Node* newnode = new Node(item); /* If it is the first node in the tree */ if (!root) { root = newnode; order++; return; } /*find where it should go */ tmp = root; while (true) { if (tmp->data == item) { /* Key already exists. */ tmp->data = item; delete newnode; /* dont need it */ return; } else if (tmp->data > item) { if (!(tmp->left)) {/* If the key is less than tmp */ tmp->left = newnode; order++; return; } else {/* continue searching for insertion pt. */ tmp = tmp->left; } } else { if (!(tmp->right)) {/* If the key is greater than tmp */ tmp->right = newnode; order++; return; } else {/* continue searching for insertion point*/ tmp = tmp->right; } } } } template bool Bstree::inTree(T item) const { Node* tmp; if (!root) return false; /*find where it is */ tmp = root; while (true) { if (tmp->data == item) return true; else if (tmp->data > item) { if (!(tmp->left)) return false; else {/* continue searching */ tmp = tmp->left; } } else { if (!(tmp->right)) return false; else /* continue searching for insertion pt. */ tmp = tmp->right; } } } template bool Bstree::remove(const T& item) { Node* nodeptr = search(item); if (nodeptr) { remove(nodeptr); order--; return true; } return false; } template const T& Bstree::retrieve(const T& key) const { Node* nodeptr; if (!root) throw BstreeException("Exception:tree empty on retrieve()."); nodeptr = search(key); if (!nodeptr) throw BstreeException("Exception: non-existent key on retrieve()."); return nodeptr->data; } template void Bstree::inorderTraverse(FuncType apply) const { inorderTraverse(root,apply); } template long Bstree::size() const { return order; } template bool Bstree::remove(Node* node) { T data; Node replacement; Node parent = findParent(node); if (!parent) return false; if (node->left && node->right) /two children case/ { Node* lchild = node->left; Node* lprnt = node; while(lchild->right) { lprnt = lchild; lchild = lchild->right; } data = node->data; node->data = lchild->data; node = lchild; parent = lprnt; } /* one or zero child case / replacement = node->left; if (!replacement) replacement = node->right; if (parent == nullptr) / root */ { delete node; root = replacement; return true; } if (parent->left == node) parent->left = replacement; else parent->right = replacement; delete node; return true;
Please help fix the following errors in red for the code below.
(The .h file is not listed due to maximum character count)
Bstree.cpp:
using namespace std;
#include "Bstree.h"
/* Nested Node class definitions */
template <typename U>
template <typename T>
Bstree<U>::Node<T>::Node(T item)
{
data = item;
left = nullptr;
right = nullptr;
}
/* Outer Bstree class definitions */
template <typename T>
Bstree<T>::Bstree()
{
root = nullptr;
order = 0;
}
template <typename T>
Bstree<T>::~Bstree()
{
recDestroy(root);
}
template <typename T>
bool Bstree<T>::empty() const
{
return root == nullptr;
}
template<typename T>
void Bstree<T>::insert(T item)
{
Node<T>* tmp;
Node<T>* newnode = new Node<T>(item);
/* If it is the first node in the tree */
if (!root)
{
root = newnode;
order++;
return;
}
/*find where it should go */
tmp = root;
while (true)
{
if (tmp->data == item)
{ /* Key already exists. */
tmp->data = item;
delete newnode; /* dont need it */
return;
}
else if (tmp->data > item)
{
if (!(tmp->left))
{/* If the key is less than tmp */
tmp->left = newnode;
order++;
return;
}
else
{/* continue searching for insertion pt. */
tmp = tmp->left;
}
}
else
{
if (!(tmp->right))
{/* If the key is greater than tmp */
tmp->right = newnode;
order++;
return;
}
else
{/* continue searching for insertion point*/
tmp = tmp->right;
}
}
}
}
template<typename T>
bool Bstree<T>::inTree(T item) const
{
Node<T>* tmp;
if (!root)
return false;
/*find where it is */
tmp = root;
while (true)
{
if (tmp->data == item)
return true;
else if (tmp->data > item)
{
if (!(tmp->left))
return false;
else
{/* continue searching */
tmp = tmp->left;
}
}
else
{
if (!(tmp->right))
return false;
else
/* continue searching for insertion pt. */
tmp = tmp->right;
}
}
}
template<typename T>
bool Bstree<T>::remove(const T& item)
{
Node<T>* nodeptr = search(item);
if (nodeptr)
{
remove(nodeptr);
order--;
return true;
}
return false;
}
template<typename T>
const T& Bstree<T>::retrieve(const T& key) const
{
Node<T>* nodeptr;
if (!root)
throw BstreeException("Exception:tree empty on retrieve().");
nodeptr = search(key);
if (!nodeptr)
throw BstreeException("Exception: non-existent key on retrieve().");
return nodeptr->data;
}
template<typename T>
void Bstree<T>::inorderTraverse(FuncType apply) const
{
inorderTraverse(root,apply);
}
template<typename T>
long Bstree<T>::size() const
{
return order;
}
template<typename T>
bool Bstree<T>::remove(Node<T>* node)
{
T data;
Node<T> replacement;
Node<T> parent = findParent(node);
if (!parent)
return false;
if (node->left && node->right) /two children case/
{
Node<T>* lchild = node->left;
Node<T>* lprnt = node;
while(lchild->right)
{
lprnt = lchild;
lchild = lchild->right;
}
data = node->data;
node->data = lchild->data;
node = lchild;
parent = lprnt;
}
/* one or zero child case /
replacement = node->left;
if (!replacement)
replacement = node->right;
if (parent == nullptr) / root */
{
delete node;
root = replacement;
return true;
}
if (parent->left == node)
parent->left = replacement;
else
parent->right = replacement;
delete node;
return true;
}

![Bstree.cpp: In member function 'bool Bstree<T>:: remove (Bstree<T>::Node<T>*)':
Bstree.cpp:162:32: error: expected primary-expression before '/' token
162 | if (node->left && node->right) /two children case/
Bstree.cpp:162:33: error: 'two' was not declared in this scope
162 | if (node->left && node->right) /two children case/
Bstree.cpp: In instantiation of 'bool Bstree<T>:: remove (Bstree<T>::Node<T>*) [with T = std::__cxx11:: basic_string<char>]':
Bstree.cpp:127:13: required from 'bool Bstree:: remove(const T&) [with T = std::__cxx11: :basic_string]'
BstreeParser.cpp:76:25: required from here
Bstree.cpp:158:9: error: no matching function for call to 'Bstree >::Node >::Node()'
158 | Node<T> replacement;
|](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9ea0e50e-bc61-40aa-a108-fd1d63d4d69b%2F44d5c743-525d-4727-9c25-e65b3c088325%2Fzjtkwx_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

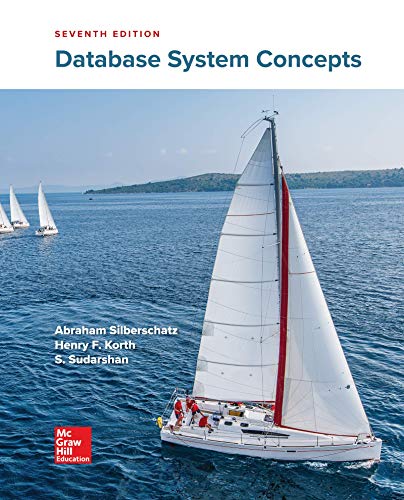
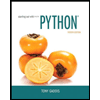
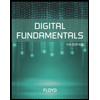
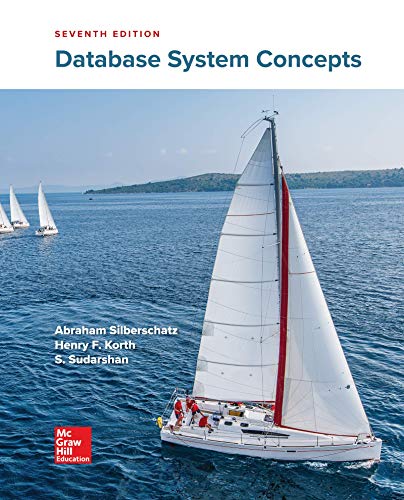
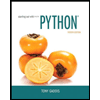
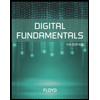
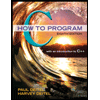
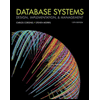
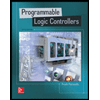