Please explain this Arduino code #include #include #include #include #include /* Set these to your desired credentials. */ const char *ssid = "admin"; //ENTER YOUR WIFI SETTINGS const char *password = "admin"; //Web/Server address to read/write from const char *host = "raw.githubusercontent.com"; const int httpsPort = 443; //HTTPS= 443 and HTTP = 80 //SHA1 finger print of certificate use web browser to view and copy const char fingerprint[] PROGMEM = " "; void setup() { pinMode(2, OUTPUT); pinMode(0, OUTPUT); pinMode(4, OUTPUT); pinMode(5, OUTPUT); delay(1000); Serial.begin(115200); WiFi.mode(WIFI_OFF); //Prevents reconnection issue (taking too long to connect) delay(1000); WiFi.mode(WIFI_STA); //Only Station No AP, This line hides the viewing of ESP as wifi hotspot WiFi.begin(ssid, password); //Connect to your WiFi router Serial.println(""); Serial.print("Connecting"); // Wait for connection while (WiFi.status() != WL_CONNECTED) { delay(0); Serial.print("."); } } void loop() { WiFiClientSecure httpsClient; //Declare object of class WiFiClient Serial.println(host); Serial.printf("Using fingerprint '%s'\n", fingerprint); httpsClient.setFingerprint(fingerprint); httpsClient.setTimeout(15000); // 15 Seconds delay(1000); Serial.print("HTTPS Connecting"); int r = 0; //retry counter while ((!httpsClient.connect(host, httpsPort)) && (r < 30)) { delay(100); Serial.print("."); r++; } if (r == 30) { Serial.println("Connection failed"); } else { Serial.println("Connected to web"); } String ADCData, getData, Link; //GET Data Link = ""; Serial.print("requesting URL: "); Serial.println(host + Link); httpsClient.print(String("GET ") + Link + " HTTP/1.1\r\n" + "Host: " + host + "\r\n" + "Connection: close\r\n\r\n"); Serial.println("request sent"); while (httpsClient.connected()) { String line = httpsClient.readStringUntil('\n'); if (line == "\r") { Serial.println("headers received"); break; } } Serial.println("reply was:"); Serial.println("=========="); String line; while (httpsClient.available()) { line = httpsClient.readStringUntil('\n'); //Read Line by Line Serial.print(line); String load = line; StaticJsonDocument<400> docum; deserializeJson(docum, load); JsonArray numbers = docum["numbers"]; for (int y = 0; y < 16; y++) { int x = numbers[y]; Serial.printf("Currently Showing: %u\n", x); if (x >= 8) { x = x - 8; digitalWrite(5, HIGH); } if (x >= 4) { x = x - 4; digitalWrite(4, HIGH); } if (x >= 2) { x = x - 2; digitalWrite(0, HIGH); } if (x >= 1) { x = x - 1; digitalWrite(2, HIGH); } delay(1000); // reset all LED state digitalWrite(2, LOW); digitalWrite(0, LOW); digitalWrite(4, LOW); digitalWrite(5, LOW); } } Serial.println("====================="); Serial.println("closing connection"); delay(5000); }
Please explain this Arduino code
#include <ESP8266WiFi.h>
#include <WiFiClientSecure.h>
#include <ESP8266WebServer.h>
#include <ESP8266HTTPClient.h>
#include <ArduinoJson.h>
/* Set these to your desired credentials. */
const char *ssid = "admin"; //ENTER YOUR WIFI SETTINGS
const char *password = "admin";
//Web/Server address to read/write from
const char *host = "raw.githubusercontent.com";
const int httpsPort = 443; //HTTPS= 443 and HTTP = 80
//SHA1 finger print of certificate use web browser to view and copy
const char fingerprint[] PROGMEM = " ";
void setup() {
pinMode(2, OUTPUT);
pinMode(0, OUTPUT);
pinMode(4, OUTPUT);
pinMode(5, OUTPUT);
delay(1000);
Serial.begin(115200);
WiFi.mode(WIFI_OFF); //Prevents reconnection issue (taking too long to connect)
delay(1000);
WiFi.mode(WIFI_STA); //Only Station No AP, This line hides the viewing of ESP as wifi hotspot
WiFi.begin(ssid, password); //Connect to your WiFi router
Serial.println("");
Serial.print("Connecting");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(0);
Serial.print(".");
}
}
void loop() {
WiFiClientSecure httpsClient; //Declare object of class WiFiClient
Serial.println(host);
Serial.printf("Using fingerprint '%s'\n", fingerprint);
httpsClient.setFingerprint(fingerprint);
httpsClient.setTimeout(15000); // 15 Seconds
delay(1000);
Serial.print("HTTPS Connecting");
int r = 0; //retry counter
while ((!httpsClient.connect(host, httpsPort)) && (r < 30)) {
delay(100);
Serial.print(".");
r++;
}
if (r == 30) {
Serial.println("Connection failed");
}
else {
Serial.println("Connected to web");
}
String ADCData, getData, Link;
//GET Data
Link = "";
Serial.print("requesting URL: ");
Serial.println(host + Link);
httpsClient.print(String("GET ") + Link + " HTTP/1.1\r\n" +
"Host: " + host + "\r\n" +
"Connection: close\r\n\r\n");
Serial.println("request sent");
while (httpsClient.connected()) {
String line = httpsClient.readStringUntil('\n');
if (line == "\r") {
Serial.println("headers received");
break;
}
}
Serial.println("reply was:");
Serial.println("==========");
String line;
while (httpsClient.available()) {
line = httpsClient.readStringUntil('\n'); //Read Line by Line
Serial.print(line);
String load = line;
StaticJsonDocument<400> docum;
deserializeJson(docum, load);
JsonArray numbers = docum["numbers"];
for (int y = 0; y < 16; y++) {
int x = numbers[y];
Serial.printf("Currently Showing: %u\n", x);
if (x >= 8) {
x = x - 8;
digitalWrite(5, HIGH);
}
if (x >= 4) {
x = x - 4;
digitalWrite(4, HIGH);
}
if (x >= 2) {
x = x - 2;
digitalWrite(0, HIGH);
}
if (x >= 1) {
x = x - 1;
digitalWrite(2, HIGH);
}
delay(1000);
// reset all LED state
digitalWrite(2, LOW);
digitalWrite(0, LOW);
digitalWrite(4, LOW);
digitalWrite(5, LOW);
}
}
Serial.println("=====================");
Serial.println("closing connection");
delay(5000);
}

Step by step
Solved in 3 steps

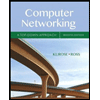
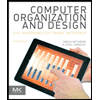
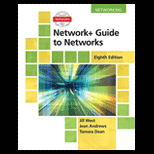
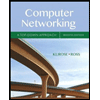
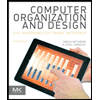
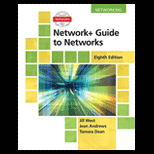
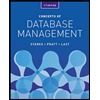
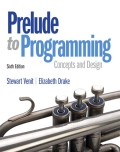
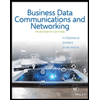