please do this in java! 3. RetailItem Exceptions Programming Challenge 4 of Chapter 6 required you to write a RetailItem class that holds data pertaining to a retail item. Write an exception class that can be instantiated and thrown when a negative number is given for the price. Write another exception class that can be instantiated and thrown when a negative number is given for the units on hand. Demonstrate the exception classes in a program. /** *Description: This program will displays a string without any user interaction *Class: Fall - COSC 1437.81002 *Assignment1: Hello World *Date: 08/15/2011 *@author Zoltan Szabo *@version 0.0.0 */ For each method, you will also be required to create docstring as follows: /** * @param String as args * @return Termination code as int, 0 for normal, anything else is error condition * @throws Nothing is implemented */ Flowcharts/UML and Pseudo code All assignment questions must show design flowchart/UML and/or pseudo code unless otherwise stated for the assignment by your instructor. Flowcharts/UMLs can be created via an application (DIA) or drawn by hand and photographed for submission. Here is the information about chapter 6 Chapter 6 - Programming Challenge 4,8 4. RetailItem Class Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields: • description. The description field references a String object that holds a brief description of the item. • unitsOnHand. The unitsOnHand field is an int variable that holds the number of units currently in inventory. • price. The price field is a double that holds the item’s retail price. Write a constructor that accepts arguments for each field, appropriate mutator methods that store values in these fields, and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them: Description Units on Hand Price Item #1 Jacket 12 59.95 Item #2 Designer Jeans 40 34.95 Item #3 Shirt 20 24.95 8. Temperature Class Write a Temperature class that will hold a temperature in Fahrenheit, and provide methods to get the temperature in Fahrenheit, Celsius, and Kelvin. The class should have the following field: • ftemp – A double that holds a Fahrenheit temperature. The class should have the following methods: • Constructor – The constructor accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field. • setFahrenheit – The setFahrenheit method accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field. • getFahrenheit – Returns the value of the ftemp field, as a Fahrenheit temperature (no conversion required). • getCelsius – Returns the value of the ftemp field converted to Celsius. • getKelvin – Returns the value of the ftemp field converted to Kelvin. Use the following formula to convert the Fahrenheit temperature to Celsius: Celsius = (5/9) * (Fahrenheit - 32) Use the following formula to convert the Fahrenheit temperature to Kelvin: Kelvin = ((5/9) * (Fahrenheit - 32)) + 273 Demonstrate the Temperature class by writing a separate program that asks the user for a Fahrenheit temperature. The program should create an instance of the Temperature class, with the value entered by the user passed to the constructor. The program should then call the object’s methods to display the temperature in Celsius and Kelvin.
please do this in java! 3. RetailItem Exceptions Programming Challenge 4 of Chapter 6 required you to write a RetailItem class that holds data pertaining to a retail item. Write an exception class that can be instantiated and thrown when a negative number is given for the price. Write another exception class that can be instantiated and thrown when a negative number is given for the units on hand. Demonstrate the exception classes in a program. /** *Description: This program will displays a string without any user interaction *Class: Fall - COSC 1437.81002 *Assignment1: Hello World *Date: 08/15/2011 *@author Zoltan Szabo *@version 0.0.0 */ For each method, you will also be required to create docstring as follows: /** * @param String as args * @return Termination code as int, 0 for normal, anything else is error condition * @throws Nothing is implemented */ Flowcharts/UML and Pseudo code All assignment questions must show design flowchart/UML and/or pseudo code unless otherwise stated for the assignment by your instructor. Flowcharts/UMLs can be created via an application (DIA) or drawn by hand and photographed for submission. Here is the information about chapter 6 Chapter 6 - Programming Challenge 4,8 4. RetailItem Class Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields: • description. The description field references a String object that holds a brief description of the item. • unitsOnHand. The unitsOnHand field is an int variable that holds the number of units currently in inventory. • price. The price field is a double that holds the item’s retail price. Write a constructor that accepts arguments for each field, appropriate mutator methods that store values in these fields, and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them: Description Units on Hand Price Item #1 Jacket 12 59.95 Item #2 Designer Jeans 40 34.95 Item #3 Shirt 20 24.95 8. Temperature Class Write a Temperature class that will hold a temperature in Fahrenheit, and provide methods to get the temperature in Fahrenheit, Celsius, and Kelvin. The class should have the following field: • ftemp – A double that holds a Fahrenheit temperature. The class should have the following methods: • Constructor – The constructor accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field. • setFahrenheit – The setFahrenheit method accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field. • getFahrenheit – Returns the value of the ftemp field, as a Fahrenheit temperature (no conversion required). • getCelsius – Returns the value of the ftemp field converted to Celsius. • getKelvin – Returns the value of the ftemp field converted to Kelvin. Use the following formula to convert the Fahrenheit temperature to Celsius: Celsius = (5/9) * (Fahrenheit - 32) Use the following formula to convert the Fahrenheit temperature to Kelvin: Kelvin = ((5/9) * (Fahrenheit - 32)) + 273 Demonstrate the Temperature class by writing a separate program that asks the user for a Fahrenheit temperature. The program should create an instance of the Temperature class, with the value entered by the user passed to the constructor. The program should then call the object’s methods to display the temperature in Celsius and Kelvin.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please do this in java!
3. RetailItem Exceptions Programming Challenge 4 of Chapter 6 required you to write a RetailItem class that holds data pertaining to a retail item. Write an exception class that can be instantiated and thrown when a negative number is given for the price. Write another exception class that can be instantiated and thrown when a negative number is given for the units on hand.
Demonstrate the exception classes in a program.
/**
*Description: This program will displays a string without any user interaction
*Class: Fall - COSC 1437.81002
*Assignment1: Hello World
*Date: 08/15/2011
*@author Zoltan Szabo
*@version 0.0.0
*/
For each method, you will also be required to create docstring as follows:
/**
* @param String as args
* @return Termination code as int, 0 for normal, anything else is error condition
* @throws Nothing is implemented
*/
Flowcharts/UML and Pseudo code
All assignment questions must show design flowchart/UML and/or pseudo code unless otherwise stated for the assignment by your instructor. Flowcharts/UMLs can be created via an application (DIA) or drawn by hand and photographed for submission.
Here is the information about chapter 6
Chapter 6 - Programming Challenge 4,8
4. RetailItem Class
Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields:
• description. The description field references a String object that holds a brief description of the item.
• unitsOnHand. The unitsOnHand field is an int variable that holds the number of units currently in inventory.
• price. The price field is a double that holds the item’s retail price.
Write a constructor that accepts arguments for each field, appropriate mutator methods that store values in these fields, and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them:
Description Units on Hand Price
Item #1 Jacket 12 59.95
Item #2 Designer Jeans 40 34.95
Item #3 Shirt 20 24.95
8. Temperature Class
Write a Temperature class that will hold a temperature in Fahrenheit, and provide methods to get the temperature in Fahrenheit, Celsius, and Kelvin. The class should have the following field: •
ftemp – A double that holds a Fahrenheit temperature.
The class should have the following methods:
• Constructor – The constructor accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field.
• setFahrenheit – The setFahrenheit method accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field.
• getFahrenheit – Returns the value of the ftemp field, as a Fahrenheit temperature (no conversion required).
• getCelsius – Returns the value of the ftemp field converted to Celsius.
• getKelvin – Returns the value of the ftemp field converted to Kelvin.
Use the following formula to convert the Fahrenheit temperature to Celsius: Celsius = (5/9) * (Fahrenheit - 32)
Use the following formula to convert the Fahrenheit temperature to Kelvin: Kelvin = ((5/9) * (Fahrenheit - 32)) + 273
Demonstrate the Temperature class by writing a separate program that asks the user for a Fahrenheit temperature. The program should create an instance of the Temperature class, with the value entered by the user passed to the constructor. The program should then call the object’s methods to display the temperature in Celsius and Kelvin.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
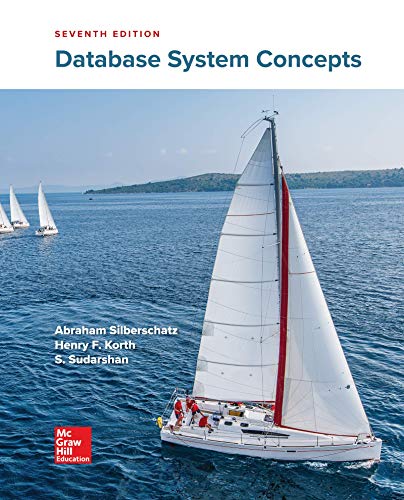
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
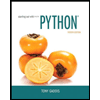
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
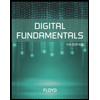
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
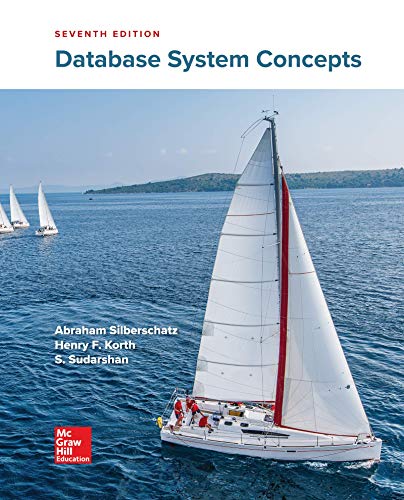
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
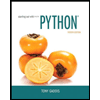
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
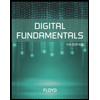
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
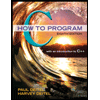
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
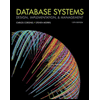
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
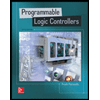
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education