picture 1 shows the coding task to perform on a file named 'df_fuel_ckan.csv' while picture 2 shows my code the question asked for and the second block of code is me testing my function on the file. how do i get past the ' AttributeError: type object 'datetime.datetime' has no attribute 'datetime''. And also is my initial block of code correct
picture 1 shows the coding task to perform on a file named 'df_fuel_ckan.csv' while picture 2 shows my code the question asked for and the second block of code is me testing my function on the file. how do i get past the ' AttributeError: type object 'datetime.datetime' has no attribute 'datetime''. And also is my initial block of code correct
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
picture 1 shows the coding task to perform on a file named 'df_fuel_ckan.csv' while picture 2 shows my code the question asked for and the second block of code is me testing my function on the file. how do i get past the ' AttributeError: type object 'datetime.datetime' has no attribute 'datetime''. And also is my initial block of code correct

Transcribed Image Text:Implement the following function according to this specification:
Function name: readDataByDate
Function parameters:
f: the file handle to read data from
startDate: the date from which to start reading data
• endDate: the date at which to stop reading data
• col: the column number read data from
Function return values: a tuple of 2 lists: the first containing the list of a datetime objects for each processed line, and the second containing the column value.
Function description:
The function should loop over the data and return values for the dates and values for the specified column for all lines after or equal to the startDate and before the endDate (not including the endDate)
Additional information and hints:
You can take advantage of the fact that the input file is ordered when looping over the contents of the file. The loop should continue until the end date (or the end of the file) is reached. There is more than one way to achieve this the particular solution is left up to you!
Before the loop you will want to create two lists to store the outputs - one for the dates and one for the column values.
Inside the loop you should read a line from the file - then extract the date from the first column. If it is before the start date - just use a continue statement to move to the next iteration. If the date is equal to or after the end date then you can use a break statement to exit the loop. If the
line is in the valid date period then you should extract the requested column - as specified in the col parameter and then append the datetime object to your dates list, and the value your column list.
Outside the loop after it has terminated you should return the dates and the column values in two lists.
To get full marks on the question you must implement a function meeting the specification above. You are recommended to follow the hints well - but are free to implement the algorithm as you see fit.
![In [3]: # write your code here (creating the function)
from datetime import datetime
def readDataByDate(f, startDate, endDate, col):
#Initialize empty lists for the output
dates
[]
values [1
#Loop through the file
for line in f:
# Split the line into columns
columns
line.strip().split(','))
# Extract the date from the first column
date
te datetime.strptime(columns [0], '%Y-%m-%d %H:M:S+00')
# Check if the date is within the specified range
if date
startDate:
continue
elif date > endDate:
break
# Extract the value from the specified column
value float(columns [col])
# Append the date and value to the output lists
dates.append(date)
values.append(value)
# Return the output lists as a tuple
return (dates, values)
v BIM & Av Tiv Hv
Text Markdown
Write a test function in the cell below. This function should open the file, read the header lines, then call your function with some sample dates and retrieve the
column for gas electricity production (column 1), Label the return values appropriately (you'll probably want to use tuple unpacking) to get two lists: one of
datetime objects and one of values from the column. Print them out one pair per line of date and value. Compare these with the values in your input data to
make sure it is working.
You might want to use the following idiom to print out values from two lists of the same size on the same line to make the output easier to check against your
input file.
# create list a
a = [1,2,3,4]
#create list b
b= ['a', 'b', 'c','d']
#join the lists into a list of tuples using zip and then tuple unpacking
#to grab the values from each list and label with c an di
for c,d in zip(a,b):
print(c,d)
In [4]: # write your code here (testing the function)
def test readDataByDate():
#Opening the file
with open('df_fuel_ckan.csv') as f:
a Reading the header lines
In [4]: write your code here (testing the function)
def
test_readDataByDate():
#Opening the file
with open('df_fuel_ckan.csv') as f:
# Reading the header lines
Out [4]:
easier to check against your input file.
#create list a
a [1,2,3,4]
=
#create list b
b = ['a', 'b', 'c','d']
#join the lists into a list of tuples using zip and then tuple unpacking
#to grab the values from each list and label with c an d
for c,d in zip(a,b):
print(c,d)
In [5]:
header1 f.readline().strip()
header2 f.readline().strip()
#calling function with example dates
start_date datetime.datetime (2009, 1, 1)
end date datetime.datetime (2010, 1, 1)
dates, values readDataByDate(f, start_date, end_date, 1)
#Printing the results one pair per line of date and value.
for date, value in zip(dates, values):
print (f"(date): {value)")
test_readDataByDate()
AttributeError
Cell In[4], line 17
Traceback (most recent call last)
for date, value in zip(dates, values):
print (f"(date): (value)")
15
16
---> 17 test_readDataByDate()
Cell In[4], line 9, in test_readDataByDate()
7 header2
f.readline().strip()
8# calling function with example dates
----> 9 start date datetime.datetime (2009, 1, 1)
10 end date
datetime.datetime (2010, 1, 1)
11 dates, values
readDataByDate(f, start_date, end_date, 1)
AttributeError: type object 'datetime.datetime' has no attribute 'datetime'
nem mewwwwww.
[1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5b1635d1-e3d3-48fc-8dcf-0165292a3c5a%2F26150b9c-6c7b-464f-ba05-b5765fc2625c%2Fno5kald_processed.png&w=3840&q=75)
Transcribed Image Text:In [3]: # write your code here (creating the function)
from datetime import datetime
def readDataByDate(f, startDate, endDate, col):
#Initialize empty lists for the output
dates
[]
values [1
#Loop through the file
for line in f:
# Split the line into columns
columns
line.strip().split(','))
# Extract the date from the first column
date
te datetime.strptime(columns [0], '%Y-%m-%d %H:M:S+00')
# Check if the date is within the specified range
if date
startDate:
continue
elif date > endDate:
break
# Extract the value from the specified column
value float(columns [col])
# Append the date and value to the output lists
dates.append(date)
values.append(value)
# Return the output lists as a tuple
return (dates, values)
v BIM & Av Tiv Hv
Text Markdown
Write a test function in the cell below. This function should open the file, read the header lines, then call your function with some sample dates and retrieve the
column for gas electricity production (column 1), Label the return values appropriately (you'll probably want to use tuple unpacking) to get two lists: one of
datetime objects and one of values from the column. Print them out one pair per line of date and value. Compare these with the values in your input data to
make sure it is working.
You might want to use the following idiom to print out values from two lists of the same size on the same line to make the output easier to check against your
input file.
# create list a
a = [1,2,3,4]
#create list b
b= ['a', 'b', 'c','d']
#join the lists into a list of tuples using zip and then tuple unpacking
#to grab the values from each list and label with c an di
for c,d in zip(a,b):
print(c,d)
In [4]: # write your code here (testing the function)
def test readDataByDate():
#Opening the file
with open('df_fuel_ckan.csv') as f:
a Reading the header lines
In [4]: write your code here (testing the function)
def
test_readDataByDate():
#Opening the file
with open('df_fuel_ckan.csv') as f:
# Reading the header lines
Out [4]:
easier to check against your input file.
#create list a
a [1,2,3,4]
=
#create list b
b = ['a', 'b', 'c','d']
#join the lists into a list of tuples using zip and then tuple unpacking
#to grab the values from each list and label with c an d
for c,d in zip(a,b):
print(c,d)
In [5]:
header1 f.readline().strip()
header2 f.readline().strip()
#calling function with example dates
start_date datetime.datetime (2009, 1, 1)
end date datetime.datetime (2010, 1, 1)
dates, values readDataByDate(f, start_date, end_date, 1)
#Printing the results one pair per line of date and value.
for date, value in zip(dates, values):
print (f"(date): {value)")
test_readDataByDate()
AttributeError
Cell In[4], line 17
Traceback (most recent call last)
for date, value in zip(dates, values):
print (f"(date): (value)")
15
16
---> 17 test_readDataByDate()
Cell In[4], line 9, in test_readDataByDate()
7 header2
f.readline().strip()
8# calling function with example dates
----> 9 start date datetime.datetime (2009, 1, 1)
10 end date
datetime.datetime (2010, 1, 1)
11 dates, values
readDataByDate(f, start_date, end_date, 1)
AttributeError: type object 'datetime.datetime' has no attribute 'datetime'
nem mewwwwww.
[1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
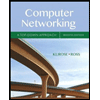
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
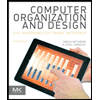
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
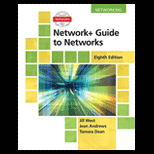
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
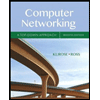
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
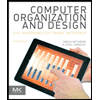
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
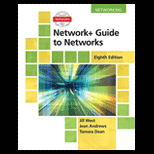
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
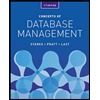
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
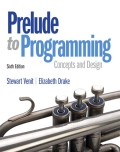
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
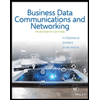
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY