Part 3. MySorter Class 1. Create a new class, MySorter. 2. Copy the quicksort, merge sort and insertion algorithms from the Sorter class to the MySorter class. 3. Change the sorting algorithms to work with arrays of Library objects instead of ints. Use the compareTo() method from the Library class to sort the Library objects by branch name. 4. Create a main() method to test the sorting algorithms on short lists of Library objects. (You can just adapt the main() method given in the Sorter class.) Use asserts. Part 5. Driver Class 1. The Driver class pulls all the pieces together. It contains a working program that will read the Library objects from a file, call the quicksort method to sort an array of Library objects and report the amount of time it takes to do the sort. 2. Run the Driver program and make sure it correctly reads data from a file, calls your quicksort method from the MySorter class and sorts the data. 3. Enable asserts which will check to be sure your sort is working correctly. 4. Study the Driver so you understand what it is doing. 5. Alter the code and repeat the test to make sure merge sort and insertion sort also correctly sort a list of Library objects. Part 6. The Experiment You may wish to change the Driver class to run multiple experiments in one go. HOWEVER, if you choose to do that, be sure to re-populate the array before every sort. That is, do not sort data that is already sorted. Read from the file and put fresh Library objects into the array before every sort. other Make sure each line in the graph is a different color and/or texture. Add a legend to the chart so the viewer can tell what each line means. Write a brief report on the experiment. Address these points: What is the expected (theoretical) performance of each sort? (For example, O(N), O(N2), O(lg N), O(N lg N).) What is the actual performance of each sort as revealed by your data? Hint: Look at the curves in your graph. According to your data, which sort is best? Which is worst? What did you learn from doing this project?
Part 3. MySorter Class
1. Create a new class, MySorter.
2. Copy the quicksort, merge sort and insertion
3. Change the sorting algorithms to work with arrays of Library objects instead of ints. Use the compareTo() method from the Library class to sort the Library objects by branch name.
4. Create a main() method to test the sorting algorithms on short lists of Library objects. (You can just adapt the main() method given in the Sorter class.) Use asserts.
Part 5. Driver Class
1. The Driver class pulls all the pieces together. It contains a working program that will read the Library objects from a file, call the quicksort method to sort an array of Library objects and report the amount of time it takes to do the sort.
2. Run the Driver program and make sure it correctly reads data from a file, calls your quicksort method from the MySorter class and sorts the data.
3. Enable asserts which will check to be sure your sort is working correctly.
4. Study the Driver so you understand what it is doing.
5. Alter the code and repeat the test to make sure merge sort and insertion sort also correctly sort a list of Library objects.
Part 6. The Experiment
You may wish to change the Driver class to run multiple experiments in one go. HOWEVER, if you choose to do that, be sure to re-populate the array before every sort. That is, do not sort data that is already sorted. Read from the file and put fresh Library objects into the array before every sort.
other
- Make sure each line in the graph is a different color and/or texture.
- Add a legend to the chart so the viewer can tell what each line means.
Write a brief report on the experiment. Address these points:
- What is the expected (theoretical) performance of each sort? (For example, O(N), O(N2), O(lg N), O(N lg N).)
- What is the actual performance of each sort as revealed by your data? Hint: Look at the curves in your graph.
- According to your data, which sort is best? Which is worst?
- What did you learn from doing this project?



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

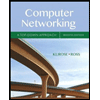
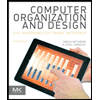
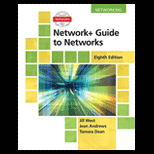
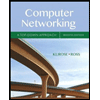
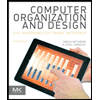
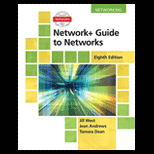
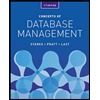
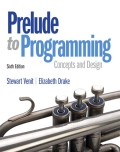
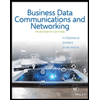