Overview In this project, we are going to build a discrete-time event simulator for a First-Come First-Served (FCFS) CPU scheduling algorithm. The goal of this project is to assess the impact of different workloads on the following (observed) performance metrics: • The average turnaround time of processes • The total throughput (number of processes done per unit time) ⚫ The average CPU utilization The average number of processes in the Ready Queue The simulator needs to generate a sequence of processes. For each process, we need to generate its arrival time and its requested service time. We can assume that processes arrive with an average rate λ and the arrivals follow a Poisson Distribution (hence Exponentially Distributed interarrival times). The service times are generated according to an Exponential Distribution. We will vary a to simulate different loads while keeping the average service time fixed. The simulator should stop after 10,000 processes complete, then it should output the metrics above. Events (e.g., process arrival, process departure) that occur causes the simulator to update its current state (e.g., CPU busy/idle, number of processes in the Ready Queue). Events are to be kept in a priority queue (which can be implemented by a sorted linked list) called Event Queue that describes the future events and is kept sorted by the time of each event. The simulator keeps a clock variable the represents the current time which is initially set as 0. The initialization also creates the first event the arrival of the first process (its time can be obtained by 0 plus a generated interarrival time) and adds it into the Event Queue. As the simulator runs and events are handled, additional future events may be added to the Event Queue. For example, when the simulation clock reaches an arrival event, we can schedule the next arrival event and determine its event time by generating an interarrival time. As another example, at the time when a process just begins its service (execution) on CPU, we can schedule a departure event in the future and determine its event time (i.e., the finish time of this process just begins being serviced) by generating a service time, because FCFS is non-preemptive. Notice that time hops between events, so you would need to update your simulator clock accordingly. Note that the Ready Queue is not to be confused with the Event Queue. For the purpose of this assignment, it might not be necessary to actually implement a Ready Queue; rather, keep number of processes in the Ready Queue as part of the system state should be sufficient. On the other hand, Event Queue would need to be implemented (as a sorted linked list) to progress the simulation. The Runs The simulator should take 2 command-line arguments (or write a "simulator-run" function that takes 2 arguments, if command-line stuffs are too difficult to you): The first is the average arrival rate and the second is the average service time. We will vary the average arrival rate, λ, of processes from 10 processes per second to 30 processes per second with increment step of 1 (i.e., we are doing 21 simulation runs for λ = 10, λ= 11, λ=12, ... λ = 30, respectively). The service time is generated according to an Exponential Distribution with an average service time of 0.04 second. For each run, you would need to output the four itemized metrics above. The Plots The report would include a brief description of the results and show a single plot for each one of the above metrics (with as the x-axis). That is, each plot would have 21 data point, and there are four plots (one for each metrics). A brief description and/or discussion on the interpretation of the plots should also be included.
Overview In this project, we are going to build a discrete-time event simulator for a First-Come First-Served (FCFS) CPU scheduling algorithm. The goal of this project is to assess the impact of different workloads on the following (observed) performance metrics: • The average turnaround time of processes • The total throughput (number of processes done per unit time) ⚫ The average CPU utilization The average number of processes in the Ready Queue The simulator needs to generate a sequence of processes. For each process, we need to generate its arrival time and its requested service time. We can assume that processes arrive with an average rate λ and the arrivals follow a Poisson Distribution (hence Exponentially Distributed interarrival times). The service times are generated according to an Exponential Distribution. We will vary a to simulate different loads while keeping the average service time fixed. The simulator should stop after 10,000 processes complete, then it should output the metrics above. Events (e.g., process arrival, process departure) that occur causes the simulator to update its current state (e.g., CPU busy/idle, number of processes in the Ready Queue). Events are to be kept in a priority queue (which can be implemented by a sorted linked list) called Event Queue that describes the future events and is kept sorted by the time of each event. The simulator keeps a clock variable the represents the current time which is initially set as 0. The initialization also creates the first event the arrival of the first process (its time can be obtained by 0 plus a generated interarrival time) and adds it into the Event Queue. As the simulator runs and events are handled, additional future events may be added to the Event Queue. For example, when the simulation clock reaches an arrival event, we can schedule the next arrival event and determine its event time by generating an interarrival time. As another example, at the time when a process just begins its service (execution) on CPU, we can schedule a departure event in the future and determine its event time (i.e., the finish time of this process just begins being serviced) by generating a service time, because FCFS is non-preemptive. Notice that time hops between events, so you would need to update your simulator clock accordingly. Note that the Ready Queue is not to be confused with the Event Queue. For the purpose of this assignment, it might not be necessary to actually implement a Ready Queue; rather, keep number of processes in the Ready Queue as part of the system state should be sufficient. On the other hand, Event Queue would need to be implemented (as a sorted linked list) to progress the simulation. The Runs The simulator should take 2 command-line arguments (or write a "simulator-run" function that takes 2 arguments, if command-line stuffs are too difficult to you): The first is the average arrival rate and the second is the average service time. We will vary the average arrival rate, λ, of processes from 10 processes per second to 30 processes per second with increment step of 1 (i.e., we are doing 21 simulation runs for λ = 10, λ= 11, λ=12, ... λ = 30, respectively). The service time is generated according to an Exponential Distribution with an average service time of 0.04 second. For each run, you would need to output the four itemized metrics above. The Plots The report would include a brief description of the results and show a single plot for each one of the above metrics (with as the x-axis). That is, each plot would have 21 data point, and there are four plots (one for each metrics). A brief description and/or discussion on the interpretation of the plots should also be included.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
it is NOT allowed to use any library or
package function to directly generate random numbers that follow Poisson Distribution,
Exponential Distribution, or any other advanced distributions. --- Writing such random
number generators is a main purpose of this assignment.
The only exception that you are allowed to directly use is the ones that generate random
numbers that follow a Uniform Distribution. E.g., rand() in C.
package function to directly generate random numbers that follow Poisson Distribution,
Exponential Distribution, or any other advanced distributions. --- Writing such random
number generators is a main purpose of this assignment.
The only exception that you are allowed to directly use is the ones that generate random
numbers that follow a Uniform Distribution. E.g., rand() in C.
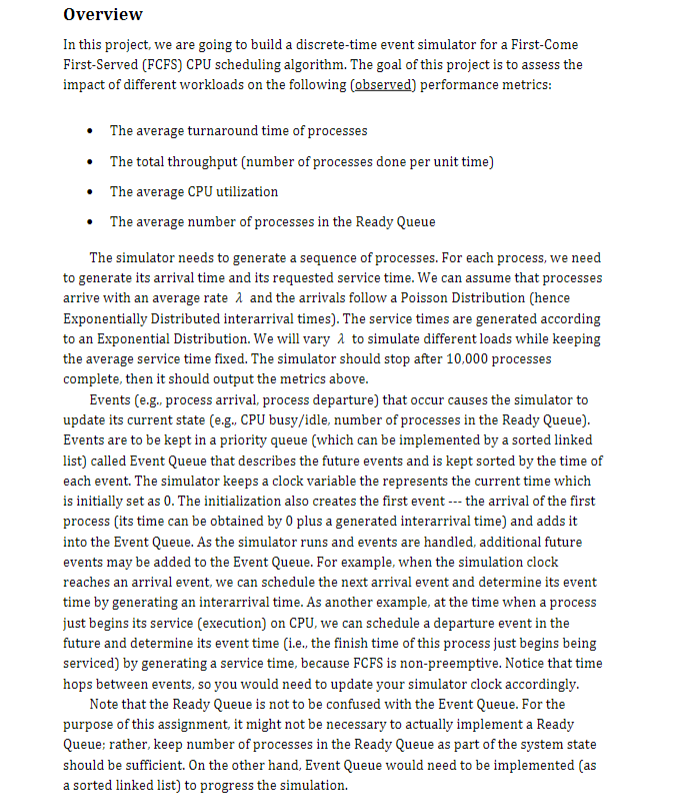
Transcribed Image Text:Overview
In this project, we are going to build a discrete-time event simulator for a First-Come
First-Served (FCFS) CPU scheduling algorithm. The goal of this project is to assess the
impact of different workloads on the following (observed) performance metrics:
• The average turnaround time of processes
•
The total throughput (number of processes done per unit time)
⚫ The average CPU utilization
The average number of processes in the Ready Queue
The simulator needs to generate a sequence of processes. For each process, we need
to generate its arrival time and its requested service time. We can assume that processes
arrive with an average rate λ and the arrivals follow a Poisson Distribution (hence
Exponentially Distributed interarrival times). The service times are generated according
to an Exponential Distribution. We will vary a to simulate different loads while keeping
the average service time fixed. The simulator should stop after 10,000 processes
complete, then it should output the metrics above.
Events (e.g., process arrival, process departure) that occur causes the simulator to
update its current state (e.g., CPU busy/idle, number of processes in the Ready Queue).
Events are to be kept in a priority queue (which can be implemented by a sorted linked
list) called Event Queue that describes the future events and is kept sorted by the time of
each event. The simulator keeps a clock variable the represents the current time which
is initially set as 0. The initialization also creates the first event the arrival of the first
process (its time can be obtained by 0 plus a generated interarrival time) and adds it
into the Event Queue. As the simulator runs and events are handled, additional future
events may be added to the Event Queue. For example, when the simulation clock
reaches an arrival event, we can schedule the next arrival event and determine its event
time by generating an interarrival time. As another example, at the time when a process
just begins its service (execution) on CPU, we can schedule a departure event in the
future and determine its event time (i.e., the finish time of this process just begins being
serviced) by generating a service time, because FCFS is non-preemptive. Notice that time
hops between events, so you would need to update your simulator clock accordingly.
Note that the Ready Queue is not to be confused with the Event Queue. For the
purpose of this assignment, it might not be necessary to actually implement a Ready
Queue; rather, keep number of processes in the Ready Queue as part of the system state
should be sufficient. On the other hand, Event Queue would need to be implemented (as
a sorted linked list) to progress the simulation.
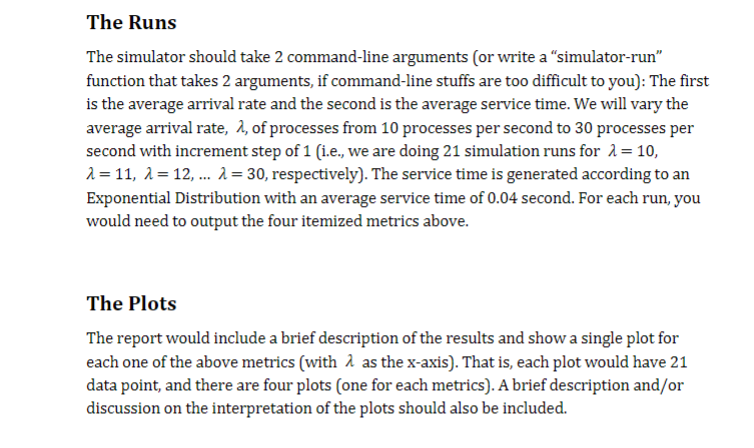
Transcribed Image Text:The Runs
The simulator should take 2 command-line arguments (or write a "simulator-run"
function that takes 2 arguments, if command-line stuffs are too difficult to you): The first
is the average arrival rate and the second is the average service time. We will vary the
average arrival rate, λ, of processes from 10 processes per second to 30 processes per
second with increment step of 1 (i.e., we are doing 21 simulation runs for λ = 10,
λ= 11, λ=12, ... λ = 30, respectively). The service time is generated according to an
Exponential Distribution with an average service time of 0.04 second. For each run, you
would need to output the four itemized metrics above.
The Plots
The report would include a brief description of the results and show a single plot for
each one of the above metrics (with as the x-axis). That is, each plot would have 21
data point, and there are four plots (one for each metrics). A brief description and/or
discussion on the interpretation of the plots should also be included.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 1 steps

Recommended textbooks for you
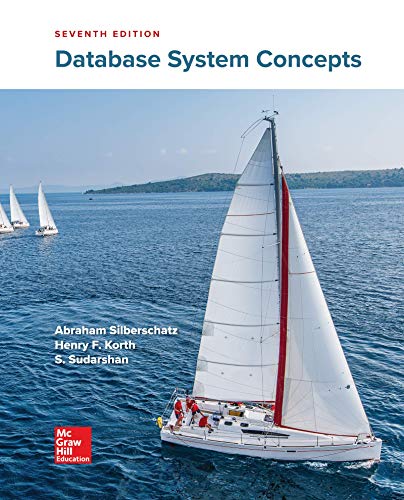
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
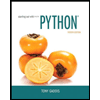
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
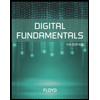
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
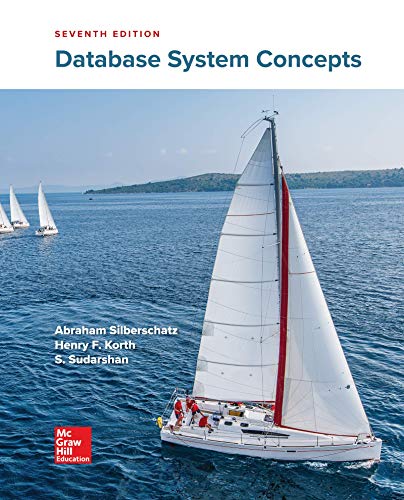
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
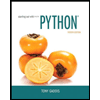
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
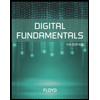
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
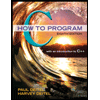
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
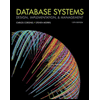
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
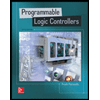
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education