o Calculate state withholding tax ( stateTax ) at 6.5 percent o Calculate federal withholding tax ( federalTax ) at 28.0 percent. o Calculate dependent deductions ( dependentDeduction ) at 2.5 percent of the employee's salary for each dependent. o Calculate total withholding ( totalwithholding ) as stateTax + federalTax + dependentDeduction. o Calculate take-home pay ( takeHomePay ) as salary- totalwithholding.
o Calculate state withholding tax ( stateTax ) at 6.5 percent o Calculate federal withholding tax ( federalTax ) at 28.0 percent. o Calculate dependent deductions ( dependentDeduction ) at 2.5 percent of the employee's salary for each dependent. o Calculate total withholding ( totalwithholding ) as stateTax + federalTax + dependentDeduction. o Calculate take-home pay ( takeHomePay ) as salary- totalwithholding.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 6PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
// This program calculates an employee's take home pay.
#include <iostream>
using namespace std;
int main()
{
double salary = 1250.00;
double stateTax;
double federalTax;
double numDependents = 2;
double dependentDeduction;
double totalWithholding;
double takeHomePay;
// Calculate state tax here
cout << "State Tax: $" << stateTax << endl;
// Calculate federal tax here
cout << "Federal Tax: $" << federalTax << endl;
// Calculate dependent deduction here
cout << "Dependents: $" << dependentDeduction << endl;
// Calculate total withholding here
// Calculate take-home pay here
cout << "Salary: $" << salary << endl;
cout << "Take-Home Pay: $" << takeHomePay << endl;
return 0;
}

Transcribed Image Text:2 https://ng.cengage.com/static/nb/ui/evo/index.html?elSBN=9781337274609&snapshotld%3D2222814&id%3D10754605:
min v
CENGAGE MINDTAP
Understanding Sequential Statements in C++
ome
0 Understanding Sequential
Payroll.cpp
ses
Statements
1 // This program calc
og and Study Tools
2 #include <iostream>
open in the code editor.
3 using namespace std;
4 int main()
her Offers
2. Variables have been declared and initialized for
cal Options
double salary
-12
you as needed, and the input and output
double stateTax;
ege Success Tips
statements have been written. Read the code
double federalTax;
carefully before you proceed to the next step.
double numDependent
eer Success Tips
double dependentDec
3. Write the C++ code needed to perform the
11
double totalwithhol
NDED FOR YOU
following:
12
double takeHomePay;
13
o Calculate state withholding tax ( stateTax ) at
14
/ Calculate state
15
6.5 percent
16
cout << State Tax
v Frontier of College
o Calculate federal withholding tax ( federalTax
17
/ Calculate federal
18
aics
) at 28.0 percent.
cout <<"Federal Tax
o Calculate dependent deductions (
20
/Calculate depender
elp
dependentDeduction ) at 2.5 percent of the
22
cout <<"Dependents:
employee's salary for each dependent.
/ Calculate total wi
Sive Feedback
2B
o Calculate total withholding ( totalwithholding
WCalculate take-home
)as stateTax+ federalTax, +
26
dependentDeduction.
27
cout <Salary: $e
cout <<Take-Home Pay
o Calculate take-home pay ( takeHonePay ) as
return 8
salary
totalwithholding.
4. Compile and execute your program by clicking the
Run button. You should get the following output:
State Tax: $81.25
Federal Tax: $350
Dependents: $62.5
Salary: $1250
Take-Home Pay: $756.25
耳
5)
acer

Transcribed Image Text:https://ng.cengage.com/static/nb/ui/evo/index.html?elSBN=9781337274609&snapshotld%3D2222814&id3D107
CENGAGE MINDTAP
Understanding Sequential Statements in C++
Understanding Sequential
Payroll.cpp
1// This progra
三
Statements
2 #include <iosti
nd Study Tools
3 using namespace
4 int main()
ffers
Summary
5 {
double salar
otions
In this lab, you complete a C++ program with the
double stateT
provided data files. The program calculates the
uccess Tips
81
double federa
amount of tax withheld from an employee's weekly
double numDep
6.
uccess Tips
salary, the tax deduction to which the employee is
10
double depende
entitled for each dependent, and the employee's
11
double totalWi
OFOR YOU
12
double takeHom
take- home pay. The program output includes state
13
tax withheld, federal tax withheld, dependent tax
14
// Calculate s
deductions, salary, and take-home pay.
15
16
cout << "state
Instructions
17
//Calculate fe
ntier of College
18
1. Ensure the source code file named Payroll.cpp is
19
cout << "Federal
20
//Calculate dep
open in the code editor.
21
22
cout << "Depender
2. Variables have been declared and initialized for
23
// Calculate tota
eedback
you as needed, and the input and output
24
statements have been written. Read the code
25
// Calculate take
26
carefully before you proceed to the next step.
27
cout << "Salary: 5
28
cout << "Take-Home
3. Write the C++ code needed to perform the
29
return 0;
following:
30}
31
o Calculate state withholding tax ( stateTax ) at
6.5 percent
o Calculate federal withholding tax ( federalTax
) at 28.0 percent.
o Calculate dependent deductions (
dependentDeduction ) at 2.5 percent of the
employee's salary for each dependent.
o Calculate total withholding ( totalwithholding
as stateTax + federalTax +
cer
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
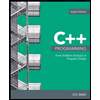
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
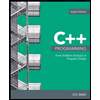
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning