nstructions 4. void Schedule_Vaccinee(schedType *ptr_sched, vaccineeType VACCINEE[], int n) Vaccinees are scheduled based on priority, i.e., those with priority 1 will be vaccinated first before those with priority 2; those with priority 2 are scheduled before 3, and those with priority 3 are scheduled before 4. Tie-breaker: vaccinees with the same priority will be sequenced based on their ID number, i.e., the person with lower ID number will be scheduled first before the one with a higher ID number. Assume that the VACCINEE[] array is already sorted in ascending order by ID number which means -- there’s no need for you to do any sorting. Implement Schedule_Vaccinee() which will update the contents of the sched structure indirectly via the formal parameter ptr_sched. The following sched structure members will change after executing this function: ● n_slots_taken ● VID[] array The n_slots_taken member will indicate how many slots are already taken, i.e., how many people were scheduled. Assume that the n_slots_taken member was initialized to 0 before the call to Schedule_Vaccinee() function. The VID[] array will contain the vaccinees’ ID numbers. The sequence or order of vaccinees is given by the array indices, i.e., VID[0] indicates the ID number of the 1st person to be vaccinated, followed by VID[1], then VID[2], and so forth… The last person in the sequence is the person with an ID stored in VID[n_slots_taken - 1]. TO DO #4: Implement Schedule_Vaccinee() as described above. You must acccess the sched structure members indirectly via the formal structure pointer parameter ptr_sched. @param: ptr_sched contains the memory address the variable of type schedType @param: VACCINEE[] is the array of structures (containing info about people who will be scheduled for vaccination) @param: n is the actual number of elements in VACCINEE[] where n <= MAXSIZE. returns: none (no return value) */ void Schedule_Vaccinee(schedType *ptr_sched, vaccineeType VACCINEE[], int n) { /* Declare your own local variables. Do NOT call printf() or scanf() in this function. */ }
I need help in constructing the function towards the end (bold highlighted).
Following are the given struct that can't be changed
#define MAXSIZE 100
#define MAXSLOTS 100
// DO NOT change the following typedef
typedef char String[11];
// date structure data type
struct dateTag {
int month;
int day;
int year;
};
typedef struct dateTag dateType;
// schedule structure data type
struct schedTag {
dateType date;
int n_slots_taken; // number of slots already taken
int VID[MAXSLOTS]; // VID means Vaccinee ID. VID[i] stores the ID number of the person to be vaccinated
// ex. VID[0] = 102
// means that the 1st person to be vaccinated during
// schedule is the person with ID number 102.
};
typedef struct schedTag schedType;
// name structure data type
struct nameTag {
String last;
String first;
};
typedef struct nameTag nameType;
// vaccinee structure data type
struct vaccineeTag {
int ID; // ID number of the vaccinee, note that this number is system-generated (i.e., not a user input)
nameType name; // name of vacinee
int age; // age of vacinee
int bool_frontliner; // 0 means not frontliner, 1 means frontliner
int bool_comorbidity; // 0 means no comorbidity, 1 means with comorbiditity
int priority; // 0 means priority not yet set, 1 to 4 means priority is set
// where 1 is the highest priority and 4 is the lowest priority
};
typedef struct vaccineeTag vaccineeType;
Instructions
4. void Schedule_Vaccinee(schedType *ptr_sched, vaccineeType VACCINEE[], int n)
Vaccinees are scheduled based on priority, i.e., those with priority 1 will be vaccinated first before those with priority 2; those with priority 2 are scheduled before 3, and those with priority 3 are scheduled before 4.
Tie-breaker: vaccinees with the same priority will be sequenced based on their ID number, i.e., the person with lower ID number will be scheduled first before the one with a higher ID number. Assume that the VACCINEE[] array is already sorted in ascending order by ID number which means -- there’s no need for you to do any sorting.
Implement Schedule_Vaccinee() which will update the contents of the sched structure indirectly via the formal parameter
ptr_sched. The following sched structure members will change after executing this function:
● n_slots_taken
● VID[] array
The n_slots_taken member will indicate how many slots are already taken, i.e., how many people were scheduled. Assume that the n_slots_taken member was initialized to 0 before the call to Schedule_Vaccinee() function. The VID[] array will contain the vaccinees’ ID numbers. The sequence or order of vaccinees is given by the array indices, i.e., VID[0] indicates the ID number of the 1st person to be vaccinated, followed by VID[1], then VID[2], and so forth… The last person in the sequence is the person with an ID stored in VID[n_slots_taken - 1].
TO DO #4: Implement Schedule_Vaccinee() as described above.
You must acccess the sched structure members indirectly via the formal
structure pointer parameter ptr_sched.
@param: ptr_sched contains the memory address the variable of type schedType
@param: VACCINEE[] is the array of structures (containing info about people who will be scheduled for vaccination)
@param: n is the actual number of elements in VACCINEE[] where n <= MAXSIZE.
returns: none (no return value)
*/
void Schedule_Vaccinee(schedType *ptr_sched, vaccineeType VACCINEE[], int n)
{
/*
Declare your own local variables.
Do NOT call printf() or scanf() in this function.
*/
}

Step by step
Solved in 3 steps with 1 images

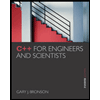
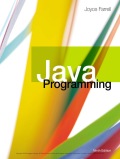
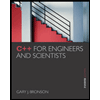
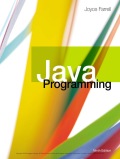