n this lab, you will create a programmer-defined class and then use it in a Java program. The program should create two Rectangle objects and find their area and perimeter. Instructions Make sure the class file named Rectangle.java is open. In the Rectangle class, create two private attributes named length and width. Both length and width should be data type double. Write public set methods to set the values for length and width. Write public get methods to retrieve the values for length and width. Write a public calculateArea()method and a public calculatePerimeter() method to calculate and return the area of the rectangle and the perimeter of the rectangle. Open the file named MyRectangleClassProgram.java. In the MyRectangleClassProgramclass, create two Rectangle objects named rectangle1 and rectangle2. Set the length of rectangle1 to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.0. Print the value of rectangle1’s perimeter and area, and then print the value of rectangle2’s perimeter and area. Execute the program.
n this lab, you will create a programmer-defined class and then use it in a Java program. The program should create two Rectangle objects and find their area and perimeter. Instructions Make sure the class file named Rectangle.java is open. In the Rectangle class, create two private attributes named length and width. Both length and width should be data type double. Write public set methods to set the values for length and width. Write public get methods to retrieve the values for length and width. Write a public calculateArea()method and a public calculatePerimeter() method to calculate and return the area of the rectangle and the perimeter of the rectangle. Open the file named MyRectangleClassProgram.java. In the MyRectangleClassProgramclass, create two Rectangle objects named rectangle1 and rectangle2. Set the length of rectangle1 to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.0. Print the value of rectangle1’s perimeter and area, and then print the value of rectangle2’s perimeter and area. Execute the program.
Chapter16: Graphics
Section: Chapter Questions
Problem 16RQ
Related questions
Question
In this lab, you will create a programmer-defined class and then use it in a Java program. The program should create two Rectangle objects and find their area and perimeter.
Instructions
- Make sure the class file named Rectangle.java is open.
- In the Rectangle class, create two private attributes named length and width. Both length and width should be data type double.
- Write public set methods to set the values for length and width.
- Write public get methods to retrieve the values for length and width.
- Write a public calculateArea()method and a public calculatePerimeter() method to calculate and return the area of the rectangle and the perimeter of the rectangle.
- Open the file named MyRectangleClassProgram.java.
- In the MyRectangleClassProgramclass, create two Rectangle objects named rectangle1 and rectangle2.
- Set the length of rectangle1 to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.0.
- Print the value of rectangle1’s perimeter and area, and then print the value of rectangle2’s perimeter and area.
- Execute the program.
// This program uses the programmer-defined Rectangle class.
public class MyRectangleClassProgram
{
publicstaticvoidmain(Stringargs[])
{
// Create rectangle1 and rectangle2 objects here.
Rectangle rectangle1=newRectangle();
Rectangle rectangle2=newRectangle();
// Set the length of rectangle1 to 10.0 here.
rectangle1.setLength(10.0);
// Set the width of rectangle1 to 5.0 here.
rectangle1.setWidth(5.0);
// Print the area and perimeter of rectangle1 here.
System.out.println("Area of rectangle1: "+rectangle1.calculateArea());
System.out.println("Perimeter of rectangle1: "+rectangle1.calculatePerimeter());
// Set the length of rectangle2 to 7.0 here.
rectangle2.setLength(7.0);
// Set the width of rectangle2 to 3.0 here.
rectangle2.setWidth(3.0);
// Print the area and perimeter of rectangle2 here.
System.out.println("Area of rectangle2: "+rectangle2.calculateArea());
System.out.println("Perimeter of rectangle2: "+rectangle2.calculatePerimeter());
System.exit(0);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
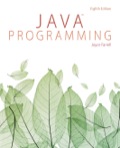
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
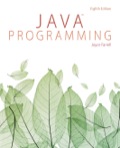
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT