My output is showing duplicating "The Surface Area(SA) of Square:". How can I display only one but get the same results? Below is my code. There is also an attached picture for question reference. Square.java public class Square { public double height; public double width; public double surfaceArea; //getter setter surfaceArea public void computeSurfaceArea(){ surfaceArea = height * width; System.out.println("The Surface Area(SA) of Square: " + surfaceArea); } public double getSurfaceArea(){ return surfaceArea; } //setter for height and width public void setHeight(double height){ this.height = height; } public void setWidth(double width){ this.width = width; } } Cube.java public class Cube extends Square { private double depth; //computation for surface area override public void computeSurfaceArea() { super.computeSurfaceArea(); System.out.println("The Surface Area(SA) of Cube: " + ((2 * super.getSurfaceArea()) + (2 * width * depth) + (2 * height * depth))); } //setter for depth public void setDepth(double depth) { this.depth = depth; } } DemoSquare.java public class DemoSquare { public static void main(String[] args) { Square s = new Square(); Cube c = new Cube(); //set values for square s.setHeight(2); s.setWidth(3); s.computeSurfaceArea(); //set values for cube c.setHeight(4); c.setWidth(5); c.setDepth(66); c.computeSurfaceArea(); } }
My output is showing duplicating "The Surface Area(SA) of Square:". How can I display only one but get the same results? Below is my code. There is also an attached picture for question reference.
Square.java
public class Square {
public double height;
public double width;
public double surfaceArea;
//getter setter surfaceArea
public void computeSurfaceArea(){
surfaceArea = height * width;
System.out.println("The Surface Area(SA) of Square: " + surfaceArea);
}
public double getSurfaceArea(){
return surfaceArea;
}
//setter for height and width
public void setHeight(double height){
this.height = height;
}
public void setWidth(double width){
this.width = width;
}
}
Cube.java
public class Cube extends Square {
private double depth;
//computation for surface area override
public void computeSurfaceArea() {
super.computeSurfaceArea();
System.out.println("The Surface Area(SA) of Cube: " + ((2 * super.getSurfaceArea()) + (2 * width * depth) + (2 * height * depth)));
}
//setter for depth
public void setDepth(double depth) {
this.depth = depth;
}
}
DemoSquare.java
public class DemoSquare {
public static void main(String[] args) {
Square s = new Square();
Cube c = new Cube();
//set values for square
s.setHeight(2);
s.setWidth(3);
s.computeSurfaceArea();
//set values for cube
c.setHeight(4);
c.setWidth(5);
c.setDepth(66);
c.computeSurfaceArea();
}
}



Step by step
Solved in 2 steps with 1 images

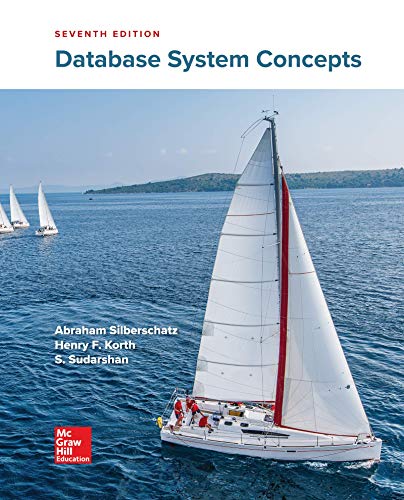
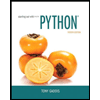
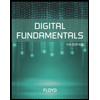
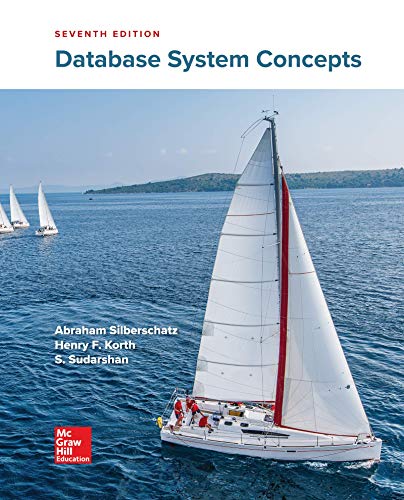
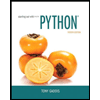
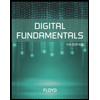
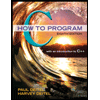
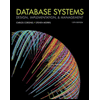
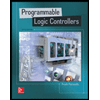