my code is working but i want to remove all the processes from main and put them into a different function. #include #include using namespace std; void holdScreen(); void developerInfo(); int askmultiplyQuestion(); double isGivenSolutionCorrect(int givenAnswer, int expectedAnswer); int numberOfCorrectResponses = 0; int numberOfResponses = 0; double percentageOfCorrectAnswers(); //*************************************************************** // // Function: main // // Description: The main function of the program // // Parameters: None // // Returns: Zero (0) // //************************************************************** int main() { // Do not delete this statement developerInfo(); while (numberOfResponses < 10) { int expectedAnswer = askmultiplyQuestion(); do { int givenAnswer; cin>> givenAnswer; numberOfResponses += 1; if (isGivenSolutionCorrect(givenAnswer, expectedAnswer)) { numberOfCorrectResponses += 1; break; } } while (numberOfResponses < 10); } // Terminate Program holdScreen(); return 0; } int askmultiplyQuestion() { int number1 = rand() % 10; int number2 = rand() % 10; cout << "How much is " << number1 << " times " << number2 << "? "; return number1 * number2; } double isGivenSolutionCorrect(int givenAnswer, int expectedAnswer){ if (givenAnswer == expectedAnswer) { char const *responses_values[] = { "Very good!", "Excellent!", "Nice work!", "Keep up the good work!" ,"Way to go!"}; int numberOfResponses = (sizeof(responses_values) / sizeof(responses_values[0])); cout << responses_values[rand() % numberOfResponses] << endl; return true; } else { char const *responses_values[] = { "That is incorrect!", "No. Please try again!", "Wrong, Try once more!", "No. Don’t give up!" ,"Incorrect. Keep trying!"}; int numberOfResponses = (sizeof(responses_values) / sizeof(responses_values[0])); cout << responses_values[rand() % numberOfResponses] << endl; return false; } double percentageOfCorrectAnswers = double(numberOfCorrectResponses) / double(numberOfResponses);{ if (percentageOfCorrectAnswers < 0.75) { cout << "Please ask your teacher for extra help." << endl; } else { cout << "Good Job!!" << endl; } return 0; } } //********************************************************************* // // Function: holdScreen // // Description: The hold screen function // // Parameters: None // // Returns: N/A // //********************************************************************* void holdScreen() { char ch; cout << "\nPress any key to exit... "; ch = getchar(); } //*************************************************************** // // Function: developerInfo // // Description: The developer's information // // Parameters: None // // Returns: N/A // //************************************************************** void developerInfo() { cout << "Name: Hussain Aldulaimi" << endl; cout << "Course: COSC 1337 Programming Fundamentals II" << endl; cout << "Program: Eight" << endl << endl; } // End of developerInfo
my code is working but i want to remove all the processes from main and put them into a different function.
#include <iostream>
#include <iomanip>
using namespace std;
void holdScreen();
void developerInfo();
int askmultiplyQuestion();
double isGivenSolutionCorrect(int givenAnswer, int expectedAnswer);
int numberOfCorrectResponses = 0;
int numberOfResponses = 0;
double percentageOfCorrectAnswers();
//***************************************************************
//
// Function: main
//
// Description: The main function of the program
//
// Parameters: None
//
// Returns: Zero (0)
//
//**************************************************************
int main()
{
// Do not delete this statement
developerInfo();
while (numberOfResponses < 10) {
int expectedAnswer = askmultiplyQuestion();
do {
int givenAnswer;
cin>> givenAnswer;
numberOfResponses += 1;
if (isGivenSolutionCorrect(givenAnswer, expectedAnswer)) {
numberOfCorrectResponses += 1;
break;
}
} while (numberOfResponses < 10);
}
// Terminate Program
holdScreen();
return 0;
}
int askmultiplyQuestion() {
int number1 = rand() % 10;
int number2 = rand() % 10;
cout << "How much is " << number1 << " times " << number2 << "? ";
return number1 * number2;
}
double isGivenSolutionCorrect(int givenAnswer, int expectedAnswer){
if (givenAnswer == expectedAnswer) {
char const *responses_values[] = { "Very good!", "Excellent!", "Nice work!", "Keep up the good work!" ,"Way to go!"};
int numberOfResponses = (sizeof(responses_values) / sizeof(responses_values[0]));
cout << responses_values[rand() % numberOfResponses] << endl;
return true;
}
else {
char const *responses_values[] = { "That is incorrect!", "No. Please try again!", "Wrong, Try once more!", "No. Don’t give up!" ,"Incorrect. Keep trying!"};
int numberOfResponses = (sizeof(responses_values) / sizeof(responses_values[0]));
cout << responses_values[rand() % numberOfResponses] << endl;
return false;
}
double percentageOfCorrectAnswers = double(numberOfCorrectResponses) / double(numberOfResponses);{
if (percentageOfCorrectAnswers < 0.75)
{ cout << "Please ask your teacher for extra help." << endl; }
else { cout << "Good Job!!" << endl; }
return 0;
}
}
//*********************************************************************
//
// Function: holdScreen
//
// Description: The hold screen function
//
// Parameters: None
//
// Returns: N/A
//
//*********************************************************************
void holdScreen()
{
char ch;
cout << "\nPress any key to exit... ";
ch = getchar();
}
//***************************************************************
//
// Function: developerInfo
//
// Description: The developer's information
//
// Parameters: None
//
// Returns: N/A
//
//**************************************************************
void developerInfo()
{
cout << "Name: Hussain Aldulaimi" << endl;
cout << "Course: COSC 1337
cout << "Program: Eight"
<< endl
<< endl;
} // End of developerInfo

Step by step
Solved in 3 steps with 1 images

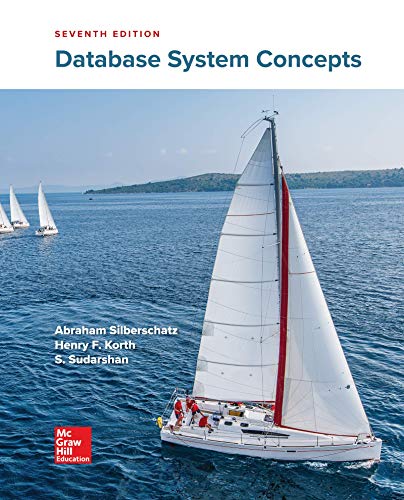
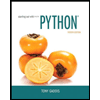
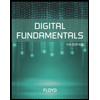
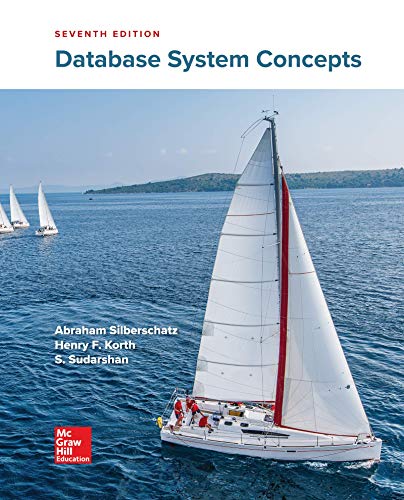
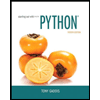
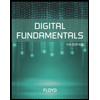
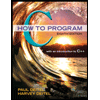
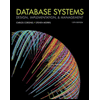
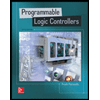