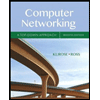
Modify the given code to accept float instead of int as a coefficient in C LANGUAGE:
// Node structure containing power and coefficient of
// variable
struct Node {
int coeff;
int pow;
struct Node* next;
};
// Function to create new node
void create_node(int x, int y, struct Node** temp)
{
struct Node *r, *z;
z = *temp;
if (z == NULL) {
r = (struct Node*)malloc(sizeof(struct Node));
r->coeff = x;
r->pow = y;
*temp = r;
r->next = (struct Node*)malloc(sizeof(struct Node));
r = r->next;
r->next = NULL;
}
else {
r->coeff = x;
r->pow = y;
r->next = (struct Node*)malloc(sizeof(struct Node));
r = r->next;
r->next = NULL;
}
}
// Function Adding two polynomial numbers
void polyadd(struct Node* poly1, struct Node* poly2,
struct Node* poly)
{
while (poly1->next && poly2->next) {
// If power of 1st polynomial is greater then 2nd,
// then store 1st as it is and move its pointer
if (poly1->pow > poly2->pow) {
poly->pow = poly1->pow;
poly->coeff = poly1->coeff;
poly1 = poly1->next;
}
// If power of 2nd polynomial is greater then 1st,
// then store 2nd as it is and move its pointer
else if (poly1->pow < poly2->pow) {
poly->pow = poly2->pow;
poly->coeff = poly2->coeff;
poly2 = poly2->next;
}
// If power of both polynomial numbers is same then
// add their coefficients
else {
poly->pow = poly1->pow;
poly->coeff = poly1->coeff + poly2->coeff;
poly1 = poly1->next;
poly2 = poly2->next;
}
// Dynamically create new node
poly->next
= (struct Node*)malloc(sizeof(struct Node));
poly = poly->next;
poly->next = NULL;
}
while (poly1->next || poly2->next) {
if (poly1->next) {
poly->pow = poly1->pow;
poly->coeff = poly1->coeff;
poly1 = poly1->next;
}
if (poly2->next) {
poly->pow = poly2->pow;
poly->coeff = poly2->coeff;
poly2 = poly2->next;
}
poly->next
= (struct Node*)malloc(sizeof(struct Node));
poly = poly->next;
poly->next = NULL;
}
}
// Display Linked list
void show(struct Node* node)
{
while (node->next != NULL) {
printf("%dx^%d", node->coeff, node->pow);
node = node->next;
if (node->coeff >= 0) {
if (node->next != NULL)
printf("+");
}
}
}
// Driver code
int main()
{
struct Node *poly1 = NULL, *poly2 = NULL, *poly = NULL;
// Create first list of 5x^2 + 4x^1 + 2x^0
create_node(5, 2, &poly1);
create_node(4, 1, &poly1);
create_node(2, 0, &poly1);
// Create second list of -5x^1 - 5x^0
create_node(-5, 1, &poly2);
create_node(-5, 0, &poly2);
printf("1st Number: ");
show(poly1);
printf("\n2nd Number: ");
show(poly2);
poly = (struct Node*)malloc(sizeof(struct Node));
// Function add two polynomial numbers
polyadd(poly1, poly2, poly);
// Display resultant List
printf("\nAdded polynomial: ");
show(poly);
return 0;
}

Step by stepSolved in 2 steps

- //the language is c++ Implement function to tokenize a string. std::vector<std::string> tokenize(std::string& line) { } int main(){ std::string ts = "This is a string with no commas semicolons or fullstops"; std::vector<std::string> tok = tokenize(ts); for (auto x : tok) { std::cout<<x<<std::endl; } return 0; }arrow_forwardC++arrow_forwardGiven the following C code: struct Node int data; struct Node struct Node next; prev; struct Node head; void funx ( ) if (head==NULL) return; struct Node current=head; while (current->next!=NULL) current=current-->next; while (current!=NULL) printf("&d ", current->data; current=current->prev; What is the functionality of this function? a) Print the contents of linked list b) Print the contents of linked list in reverse order c) Nonearrow_forward
- #include <iostream> usingnamespace std; class Queue { int size; int* queue; public: Queue(){ size = 0; queue = new int[100]; } void add(int data){ queue[size]= data; size++; } void remove(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } else{ for(int i =0; i < size -1; i++){ queue[i]= queue[i +1]; } size--; } } void print(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } for(int i =0; i < size; i++){ cout<<queue[i]<<" <- "; } cout << endl; } //your code goes here }; int main(){ Queue q1; q1.add(42); q1.add(2); q1.add(8); q1.add(1); Queue q2; q2.add(3); q2.add(66); q2.add(128); q2.add(5); Queue q3 = q1+q2; q3.print();…arrow_forwardSuppose a node of a doubly linked list is defined as follows: struct Node{ int data; struct Node* next; struct Node* prev; }; Write the function definition of the function deleteElement as presented below. This function deletes a node at position n from a doubly linked list. struct Node* deleteElement(struct Node* head, int n){ //write the function definition }arrow_forwardStack Implementation in C++make code for an application that uses the StackX class to create a stack.includes a brief main() code to test this class.arrow_forward
- C++ PROGRAMMINGTopic: Binary Search Trees Explain the c++ code below.: SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed) int childCount(node* p) { bool Cright = false; bool Cleft = false; if(p->right != NULL){ Cright = true; } if(p->left != NULL){ Cleft = true; } if(Cleft == true && Cright== true){ return 2; } if(Cright){ return 1; } if(Cleft){ return -1; } return 0; } int set(node* p, int e) { int elem = p->element; p->element = e; return elem; } node* addSibling(node* p, int e) { node* P = p->parent; if(P != NULL){ if(sibling(p) == NULL){…arrow_forwardLanguage/Type: C++ binary trees pointers recursion Write a function named hasPath that interacts with a tree of BinaryTreeNode structures representing an unordered binary tree. The function accepts three parameters: a pointer to the root of the tree, and two integers start and end, and returns true if a path can be found in the tree from start down to end. In other words, both start and end must be element data values that are found in the tree, and end must be below start, in one of start's subtrees; otherwise the function returns false. If start and end are the same, you are simply checking whether a single node exists in the tree with that data value. If the tree is empty, your function should return false. For example, suppose a BinaryTreeNode pointer named tree points to the root of a tree storing the following elements. The table below shows the results of several various calls to your function: 67 88 52 1 21 16 99 45 Call Result Reason hasPath(tree, 67, 99) true path exists…arrow_forwardC programming I need help writing a code that uses a struct pointer into a binary tree and using the same pointer into an arrayarrow_forward
- #ifndef NODES_LLOLL_H#define NODES_LLOLL_H #include <iostream> // for ostream namespace CS3358_SP2023_A5P2{ // child node struct CNode { int data; CNode* link; }; // parent node struct PNode { CNode* data; PNode* link; }; // toolkit functions for LLoLL based on above node definitions void Destroy_cList(CNode*& cListHead); void Destroy_pList(PNode*& pListHead); void ShowAll_DF(PNode* pListHead, std::ostream& outs); void ShowAll_BF(PNode* pListHead, std::ostream& outs);} #endif #include "nodes_LLoLL.h"#include "cnPtrQueue.h"#include <iostream>using namespace std; namespace CS3358_SP2023_A5P2{ // do breadth-first (level) traversal and print data void ShowAll_BF(PNode* pListHead, ostream& outs) { cnPtrQueue queue; CNode* currentNode; queue.push(lloLLPtr->getHead()); while (!queue.empty()) { currentNode = queue.front(); queue.pop(); if…arrow_forwardTree Traversal Coding: How do I code the following in C program? // ====== BEGIN INSERT FUNCTION DEFS TO WALK TREE ========= // define 3 functions - preorder, inorder, postorder to walk tree, printing out data (char) // associated with each node visited: void preorder (node* np) {} void inorder (node* np) {} void postorder (node* np) {} walk.c file with the rest of the code given. #include <stdio.h> #include <stdlib.h> #include <stdbool.h> #include <string.h> #define MAX_STRING 200 // ========================== NODE/TREE DEFINITIONS ========================== // define node structure typedef struct nd { int data; struct nd* left; struct nd* right; } node; // "new" function to create a node, set data value to d and children to NULL node* newNode(int d) { node* np; np = (node*)malloc(sizeof(node)); if (np != NULL) { np->data = d; np->left = NULL; np->right = NULL; } return(np); } // declare root of our binary tree…arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
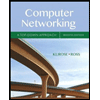
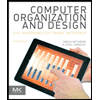
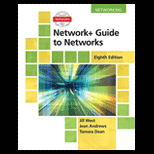
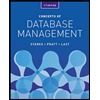
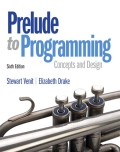
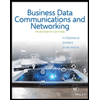