list, L, the function front_two creates a new list of
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Code in python
Given a list, L, the function front_two creates a new list of length 2 out of the first two items in L as follows:
- In the new list, the second item becomes the first item, and the first item becomes the second.
- If L contains only one item, the first item in the new list should be the value None.
- If L is empty, both items in the new list should be value None.
- The function returns the new list.
For example:
Test | Result |
---|---|
L = ['a', 'b', 'c', 'd'] print(front_two(L)) | ['b', 'a'] |
L = [] print(front_two(L)) | [None, None] |
L = [5, 2] print(front_two(L)) | [2, 5] |
L = [7] print(front_two(L)) | [None, 7] |

Summary
Creating Python Lists
In Python, lists are created by enclosing elements in square brackets [] and separating them with commas.
# list of integers
my_list = [1, 2, 3]
Lists can have any number of elements and can be of different types (integers, floats, strings, etc.). increase.
# empty list
my_list = []
# mixed data type list
my_list = [1, "Hello", 3.4]
A list can have other lists as elements. This is called a nested list.
# nested list
my_list = ["mouse", [8, 4, 6], ['a']]
Accessing list elements
There are several ways to access the elements of a list .
List Index
You can use the index operator [] to access elements in a list. In Python, indexing starts at 0, so a 5-item list has indices 0 through 4.
Any attempt to access any other index will throw an IndexError. Index must be an integer. Float and other types are not allowed. This will raise a TypeError.
Step by step
Solved in 2 steps with 1 images

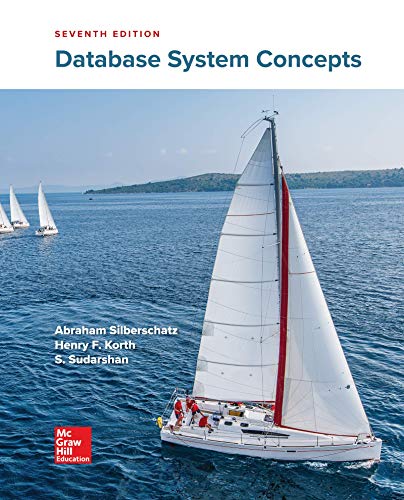
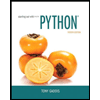
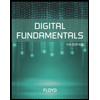
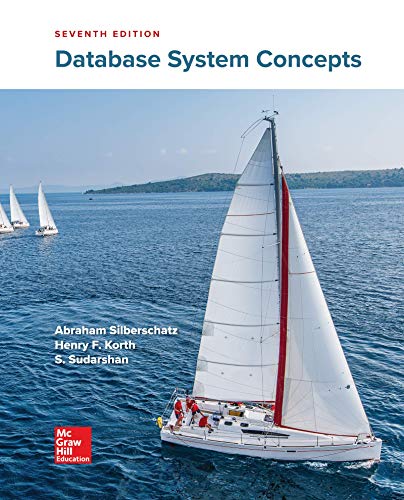
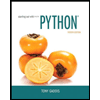
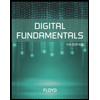
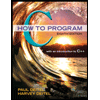
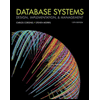
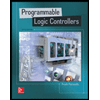