lass diagram for this coding. Show relationships using appropriate arrows. Include cardinality or multiplicity. #Create the class personType from matplotlib.pyplot import phase_spectrum class personType: #create the class constructor def __init__(self,fName,lName): #Initialize the data members self.fName = fName self.lName = lName #Method to access def getFName(self):
lass diagram for this coding. Show relationships using appropriate arrows. Include cardinality or multiplicity. #Create the class personType from matplotlib.pyplot import phase_spectrum class personType: #create the class constructor def __init__(self,fName,lName): #Initialize the data members self.fName = fName self.lName = lName #Method to access def getFName(self):
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Draw a class diagram for this coding.
Show relationships using appropriate arrows. Include cardinality or multiplicity.
#Create the class personType
from matplotlib.pyplot import phase_spectrum
class personType:
#create the class constructor
def __init__(self,fName,lName):
#Initialize the data members
self.fName = fName
self.lName = lName
#Method to access
def getFName(self):
return self.fName
def getLName(self):
return self.lName
#Method to manipulate the data members
def setFName(self,fName):
self.fName = fName
def setLName(self,lName):
self.lName = lName
#Create the class Doctor Type inherit from personType
class doctorType(personType):
#Create the constructor for the doctorType class
def __init__(self, fName, lName,speciality="unknown"):
super().__init__(fName, lName)
self.speciality = speciality
#Methods to access
def getSpeciality(self):
return self.speciality
#Methods to manipulate
def setSpeciality(self,spc):
self.speciality = spc
#create the class billType
class billType():
#Create the constructor for the billType class
def __init__(self,pId, pCharges):
#Initialize the data members
self.pId = pId
self.pCharges = pCharges
#Methods to access
def getpId(self):
return self.pId
def getpChares(self):
return self.pCharges
#Methods to manipulate
def setpId(self,id):
self.pId = id
def setpCharges(self,charges):
self.pCharges = charges
#Create the class Datetype
class dateType():
#create the constructor by initializing the parameters
def __init__(self,pDob,dAdmitted,dDischarged):
self.pDob = pDob
self.dAdmitted = dAdmitted
self.dDischaged = dDischarged
#Create the class patientType inherited from personType
class patientType(personType):
#Create the constructor for the patientType class
def __init__(self, fName, lName,pId,pAge,pDob,phyfName,phylName,dAdmitted,dDischarged):
super().__init__(fName, lName)
self.pId = pId
self.pAge = pAge
#Store the date Info in the dateType class
self.dateInfo = dateType(pDob,dAdmitted,dDischarged)
#use the doctorType class to store the physician Name
self.doctorInfo = doctorType(phyfName,phylName)
#Methods for the manipulation and access
def getpId(self):
return self.pId
def getpAge(self):
return self.pAge
def getPhyfName(self):
return self.doctorInfo.getFName()
def getPhylName(self):
return self.doctorInfo.getLName()
def getdAdmitted(self):
return self.dateInfo.dAdmitted
def getdDischarged(self):
return self.dateInfo.dDischaged
def getdDob(self):
return self.dateInfo.pDob
def setpId(self,id):
self.pId = id
def setpAge(self,age):
self.pAge = age
def setpDob(self,Dob):
self.dateInfo.pDob = Dob
def setphyFName(self,fName):
self.doctorInfo.setFName(fName)
def setphyLName(self,lName):
self.doctorInfo.setLName(lName)
def setdAttended(self,date):
self.dateInfo.dAdmitted = date
def setdDischarged(self,date):
self.dateInfo.dDischaged = date
#main method to test the classes
#Create the person class object
person1 = personType("Daniel","Guzman")
#Create the doctor class Object
doctor1 = doctorType("Elisa","Lazgano","neurosurgeon")
#use the method to get the values
print(doctor1.getFName())
#Change the name of the doctor
doctor1.setFName("Rudolfo")
#print again
print(doctor1.getFName())
#Create the patient type class object
patient1 = patientType("Daniel","Lazcano","1","18","15 Dec 2004","Rudolfo","Lazcano","15 Dec 2021","25 Dec 2021")
#Now try to manipulate
patient1.setdDischarged("24 Dec 2021")
#Try to access again
print(patient1.getdDischarged())
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
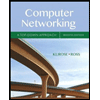
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
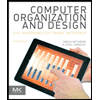
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
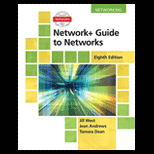
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
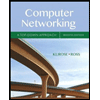
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
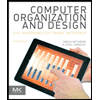
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
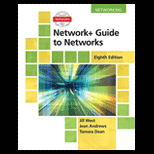
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
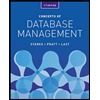
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
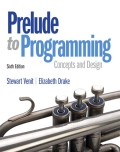
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
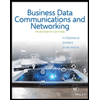
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY