Java programming language I need the two classes ( the testing class and the class with the methods) to be combined into one class. This is the program: import java.util.Scanner; //The Temperature Class Begins Here public class Temperature { private double ftemp; //The constructor public Temperature(double ftemp) { this.ftemp = ftemp; } //Get farenheit Method public double getFtemp() { return ftemp; } //Set farenheit method public void setFtemp(double ftemp) { this.ftemp = ftemp; } // Get Celcius Method public double getCelcius(double ftemp){ //double celcius = (double) (5/9) * (ftemp - 32); double celcius = (ftemp-32)* (double)5/9; return celcius; } // Get Kelvin Method public double getKelving(double ftemp){ double Kelvin; Kelvin = (ftemp-32)*(double)5/9 + 273.15; return Kelvin; } } //The Temperature Class Ends Here //The Temperature Test Class with a main method begins Here class TemperatureTest{ public static void main(String[] args) { Scanner in = new Scanner(System.in); //Prompt User for value in fahrenheit System.out.println("Enter·a·Fahrenheit·temperature:"); double ftem = in.nextDouble(); //Making the instance of the Temperature class Temperature temp = new Temperature(ftem); //Outputing Results System.out.println("The·temperature·in·Fahrenheit·i: "+ftem); System.out.println("The·temperature·in·Celcius·is: "+ temp.getCelcius(ftem)); System.out.println("The·temperature·in·Kelvin·is: " + temp.getKelving(ftem)); } }
Java programming language
I need the two classes ( the testing class and the class with the methods) to be combined into one class.
This is the program:
import java.util.Scanner;
//The Temperature Class Begins Here
public class Temperature {
private double ftemp;
//The constructor
public Temperature(double ftemp) {
this.ftemp = ftemp;
}
//Get farenheit Method
public double getFtemp() {
return ftemp;
}
//Set farenheit method
public void setFtemp(double ftemp) {
this.ftemp = ftemp;
}
// Get Celcius Method
public double getCelcius(double ftemp){
//double celcius = (double) (5/9) * (ftemp - 32);
double celcius = (ftemp-32)* (double)5/9;
return celcius;
}
// Get Kelvin Method
public double getKelving(double ftemp){
double Kelvin;
Kelvin = (ftemp-32)*(double)5/9 + 273.15;
return Kelvin;
}
}
//The Temperature Class Ends Here
//The Temperature Test Class with a main method begins Here
class TemperatureTest{
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
//Prompt User for value in fahrenheit
System.out.println("Enter·a·Fahrenheit·temperature:");
double ftem = in.nextDouble();
//Making the instance of the Temperature class
Temperature temp = new Temperature(ftem);
//Outputing Results
System.out.println("The·temperature·in·Fahrenheit·i: "+ftem);
System.out.println("The·temperature·in·Celcius·is: "+ temp.getCelcius(ftem));
System.out.println("The·temperature·in·Kelvin·is: " + temp.getKelving(ftem));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

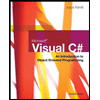
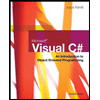