Introduction Some number of teams are participating in a race. You are not told how many teams are participating but you do know that: • Each team has a name, which is one of the uppercase letters A-Z. • No two teams have the same name, so there are a maximum number of 26 teams. • Each team has the same number of members. • No two runners cross the finish line at the same time - i.e. there are no ties. At the end of the race, we can write the results as a string of characters indicating the order in which runners crossed the finish line. Input Your program will ask the user to input a string of uppercase characters indicating the outcome of a race. Output Your program will output: The team's score is the sum of the members score divided by the number of people on the team. So, team A's score is (3+5+6)/3 = 14/3=4.66 and team Z's score is (1+2+4)/3=7/3=2.33 • The number of teams. • The number of runners on a team. • The names of the teams - in alphabetical order - together with the team score. • The name and score of the winning team. So, for the example above the program will print: There are 2 teams. For example: ZZAZAA We can see there were two teams: A and Z. Team A's runners finished in Each team has 3 runners. 3rd, 5th and 6th place. Team Z's runners finished in 1st, 2nd and 4th place. Scoring the race Each runner is assigned a score equal to their finishing place. In the example above team Z's runners achieved scores of 1, 2 and 4. Team A's The winning team is team Z with a score of 2.33. runners scores were 3, 5, and 6 respectively. Team Score 4.66 A Z 2.33 Your program should loop asking for input processing the data until the user enters "done". Your program should detect the error condition where teams do not have the same number of runners. In that case it should print an error message_ and resume by requesting the next input.
Introduction Some number of teams are participating in a race. You are not told how many teams are participating but you do know that: • Each team has a name, which is one of the uppercase letters A-Z. • No two teams have the same name, so there are a maximum number of 26 teams. • Each team has the same number of members. • No two runners cross the finish line at the same time - i.e. there are no ties. At the end of the race, we can write the results as a string of characters indicating the order in which runners crossed the finish line. Input Your program will ask the user to input a string of uppercase characters indicating the outcome of a race. Output Your program will output: The team's score is the sum of the members score divided by the number of people on the team. So, team A's score is (3+5+6)/3 = 14/3=4.66 and team Z's score is (1+2+4)/3=7/3=2.33 • The number of teams. • The number of runners on a team. • The names of the teams - in alphabetical order - together with the team score. • The name and score of the winning team. So, for the example above the program will print: There are 2 teams. For example: ZZAZAA We can see there were two teams: A and Z. Team A's runners finished in Each team has 3 runners. 3rd, 5th and 6th place. Team Z's runners finished in 1st, 2nd and 4th place. Scoring the race Each runner is assigned a score equal to their finishing place. In the example above team Z's runners achieved scores of 1, 2 and 4. Team A's The winning team is team Z with a score of 2.33. runners scores were 3, 5, and 6 respectively. Team Score 4.66 A Z 2.33 Your program should loop asking for input processing the data until the user enters "done". Your program should detect the error condition where teams do not have the same number of runners. In that case it should print an error message_ and resume by requesting the next input.
Operations Research : Applications and Algorithms
4th Edition
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Wayne L. Winston
Chapter19: Probabilistic Dynamic Programming
Section19.4: Further Examples Of Probabilistic Dynamic Programming Formulations
Problem 7P
Related questions
Question
C++
Hi! I need help answering Question 1 properly in C++, I messed up on the calculation. Down below I provided the Introduction to the question and what the output should look like. Thank you.

Transcribed Image Text:Introduction
Some number of teams are participating in a race. You are not told how
many teams are participating but you do know that:
• Each team has a name, which is one of the uppercase letters A-Z.
• No two teams have the same name, so there are a maximum
number of 26 teams.
• Each team has the same number of members.
• No two runners cross the finish line at the same time - i.e. there are
no ties.
At the end of the race, we can write the results as a string of characters
indicating the order in which runners crossed the finish line.
Input
Your program will ask the user to input a string of uppercase characters
indicating the outcome of a race.
Output
Your program will output:
The team's score is the sum of the members score divided by the number
of people on the team. So, team A's score is (3+5+6)/3 = 14/3=4.66 and
team Z's score is (1+2+4)/3=7/3=2.33
The number of teams.
• The number of runners on a team.
• The names of the teams - in alphabetical order together with the
team score.
• The name and score of the winning team.
So, for the example above the program will print:
There are 2 teams.
For example: ZZAZAA
We can see there were two teams: A and Z. Team A's runners finished in Each team has 3 runners.
3rd, 5th and 6th place. Team Z's runners finished in 1st, 2nd and 4th place.
Scoring the race
Each runner is assigned a score equal to their finishing place. In the
example above team Z's runners achieved scores of 1, 2 and 4. Team A's The winning team is team Z with a score of 2.33.
runners scores were 3, 5, and 6 respectively.
Team Score
4.66
2.33
A
Z
Your program should loop asking for input processing the data until the
user enters “done”.
Your program should detect the error condition where teams do not have
the same number of runners. In that case it should print an error message,
and resume by requesting the next input.

Transcribed Image Text:Example 1 output for score the race
(input in bold) :
Please enter the team score
1. Each team has a name
which is one of the uppercase letters A-Z.
2. No two teams have the same name.
There are a maximum number of 26 teams.
3. Each team has the same number of members.
No two runners cross the finish line at the
same time.
i.e., there are no ties.
Enter the Team and player: ACBBCAACBZZZRERERE
There are 6 teams.
Each team has 3 runners.
Team Score
A 4.66667
C 5
B 5.33333
Z 11
R
15
E
16
Example 1 output for score the race
(input in bold) :
Please enter the team score
1. Each team has a name
which is one of the uppercase letters A-Z.
2. No two teams have the same name.
There are a maximum number of 26 teams.
3. Each team has the same number of members.
No two runners cross the finish line at the
same time.
i.e., there are no ties.
Enter the Team and player: asdad
It's invalid input.
Please re-enter the team score: asdadw
It's invalid input.
Please re-enter the team score: aasda
It's invalid input.
Please re-enter the team score: azz
It's invalid input.
Please re-enter the team score: azzaaz
There are 2 teams.
Each team has 3 runners.
Team Score
a 3.33333
z 3.66667
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
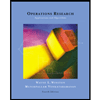
Operations Research : Applications and Algorithms
Computer Science
ISBN:
9780534380588
Author:
Wayne L. Winston
Publisher:
Brooks Cole
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
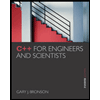
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
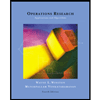
Operations Research : Applications and Algorithms
Computer Science
ISBN:
9780534380588
Author:
Wayne L. Winston
Publisher:
Brooks Cole
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
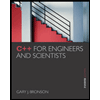
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr