Intro to Java Instuctions: Design and implement a program that reads a series of 10 integers from the user and prints their average For each of the 10 numbers input from the user: one at a time, prompt the user to enter a number. Then read input from the user as a string. Attempt to convert it to an integer using the "Integer.parseInt" method. If the process throws a "NumberFormatExeption", print an appropriate error message and prompt the user for the number again. Continue prompting and reading in number until 10 valid integers have been read Print "The average is" and then print the average of the 10 numbers
Intro to Java Instuctions: Design and implement a program that reads a series of 10 integers from the user and prints their average For each of the 10 numbers input from the user: one at a time, prompt the user to enter a number. Then read input from the user as a string. Attempt to convert it to an integer using the "Integer.parseInt" method. If the process throws a "NumberFormatExeption", print an appropriate error message and prompt the user for the number again. Continue prompting and reading in number until 10 valid integers have been read Print "The average is" and then print the average of the 10 numbers
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 14E
Related questions
Question
![Intro to Java
Instuctions:
Design and implement a program that reads a series of 10 integers from the user and prints their average
For each of the 10 numbers input from the user:
one at a time, prompt the user to enter a number. Then read input from the user as a string.
Attempt to convert it to an integer using the "Integer.parseInt" method.
If the process throws a "NumberFormatExeption", print an appropriate error message and prompt the user for the number again.
Continue prompting and reading in number until 10 valid integers have been read
Print "The average is" and then print the average of the 10 numbers
Code:
import java.util.Scanner;
public class Lab1MainClass {
public static final int VALUES_TO_READ = 10;
public static void main(String[] args) {
// local variables
int[] values = new int [VALUES_TO_READ];
double average;
Scanner scan = new Scanner(System.in);
int values Read = 0; // how many numbers the user has given us, a count of where we are
String userInput;
// read inputs from user
System.out.println("Please enter " + VALUES_TO_READ + "values one at a time.");
while(valuesRead<VALUES_TO_READ) {
System.out.print("Enter value #" + valuesRead + ";");
userInput = scan.nextLine(); // read in the whole line up through the user's enter key
try {
int intValue = Integer.parseInt(userInput);
// parsing was successful
values[valuesRead] = intValue;
valuesRead++;
} catch (NumberFormatException e) {
// parsing was unsuccessful
System.out.println("That's not a number, please try again.");
}
}
// calculate their average
// print out the output average
System.out.println("TODO average and output");
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F00ad0db1-c9d3-4dc7-9356-8892c2066d7c%2Fef5e3cff-df8d-4a91-bb22-6b46a6746a6b%2Fbo9tat_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Intro to Java
Instuctions:
Design and implement a program that reads a series of 10 integers from the user and prints their average
For each of the 10 numbers input from the user:
one at a time, prompt the user to enter a number. Then read input from the user as a string.
Attempt to convert it to an integer using the "Integer.parseInt" method.
If the process throws a "NumberFormatExeption", print an appropriate error message and prompt the user for the number again.
Continue prompting and reading in number until 10 valid integers have been read
Print "The average is" and then print the average of the 10 numbers
Code:
import java.util.Scanner;
public class Lab1MainClass {
public static final int VALUES_TO_READ = 10;
public static void main(String[] args) {
// local variables
int[] values = new int [VALUES_TO_READ];
double average;
Scanner scan = new Scanner(System.in);
int values Read = 0; // how many numbers the user has given us, a count of where we are
String userInput;
// read inputs from user
System.out.println("Please enter " + VALUES_TO_READ + "values one at a time.");
while(valuesRead<VALUES_TO_READ) {
System.out.print("Enter value #" + valuesRead + ";");
userInput = scan.nextLine(); // read in the whole line up through the user's enter key
try {
int intValue = Integer.parseInt(userInput);
// parsing was successful
values[valuesRead] = intValue;
valuesRead++;
} catch (NumberFormatException e) {
// parsing was unsuccessful
System.out.println("That's not a number, please try again.");
}
}
// calculate their average
// print out the output average
System.out.println("TODO average and output");
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
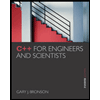
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
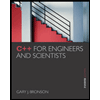
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr