In this project, a rational number is defined as a fraction in which the numerator and denominator are integers with the denominator being non-zero. Requirements To ensure consistency among solutions each implementation must implement the following requirements. • Your implementation should reflect the definition of a simplified rational number at all times. • A long (integer) is used to represent both the numerator and denominator. • The Rational class must be able to handle both positive and negative numbers. • The rational number must be in simplest form after every operation; that is, 8 6 shall be immediately reduced to 4 3 . In order to simplify a rational number properly, use the the Euclidean Algorithm to determine the greatest common divisor of the two numbers. • All methods that have an object as parameter must be able to handle an input of null. • By definition, the denominator of a fraction cannot be zero since it leads to an undefined value. In Java, this division by zero results in an ArithmeticException; the Rational class must throw an ArithmeticException when division by zero is attempted. • The denominator should be initialized to a sensible and consistent value. The numerator shall be initialized to zero. • The denominator should always be positive leaving the numerator to indicate the sign of the number (positive or negative). • The Rational class shall reside in the default package. Methods There are many methods that one would expect to be supported in a Rational class; unless specified, you will have to implement all of the described methods. Constructors • Rational() o Description: constructs a Rational initializing the value to 0. • Rational(long a) o Parameters: a – the default value for the Rational number. o Description: constructs a Rational initializing the value to 1a . • Rational(long a, long b) throws ArithmeticException o Parameters: a – an integer specifying the initial value of the numerator. b – an integer specifying the initial value of the denominator. o Description: constructs a Rational number initializing the object to a b . Accessors • long getNumerator() o Description: returns the current value of the numerator. o Returns: as an accessor method, it only returns the current value of the numerator. • long getDenominator() o Description: returns the current value of the denominator. o Returns: as an accessor method, it only returns the current value of the denominator. Mathematical Operations With each of the following methods, if the input object r is null, you may treat the input as the value zero (0). • Rational add(Rational r) o Parameters: r – the rational number to be added to this rational. o Description: adds r and this, returning a new object with the reduced sum. A common denominator is required to complete this operation. o Returns: returns a new object with the reduced sum. • Rational subtract(Rational r) o Parameters: r – the rational number to be subtracted from this rational. o Description: subtracts r from this, returning a new object with the reduced difference. A common denominator is required to complete this operation. o Returns: returns a new object with the reduced difference. • Rational multiply(Rational r) o Parameters: r – the rational number to be multiplied with thisrational o Returns: returns a new object with the reduced product. • Rational divide(Rational r) throws ArithmeticException o Parameters: r – the rational number that is to divide this rational. o Description: divides this by r, returning a new object with the reduced quotient. o Returns: returns a new object with the reduced quotient. The Greatest Common Divisor Algorithm • private long gcd(long p, long q) o Parameters: p – an integer. q – an integer. o Description: determines the greatest common divisor of the two input integers according to the Euclidean Algorithm; a quick implementation is easily available online. o Returns: the (positive) GCD of the two input integers. o Notes: It is easier to implement this method if both integers are positive values. Supplied Methods Besides the interface specification described above, there are other methods that have been provided; feel free to use them as required in your implementation and testing. Do not modify these methods. o Returns: a String representation of this rational value. • int compareTo(Object obj) o Parameters: obj – an object to compare against this rational value. o Description: determines whether the content of obj is smaller (or larger) than this rational value; the result returned is compliant with the Comparable interface that the Rational class implements. o Returns: -1, 0, or 1 when this object is less than, equal, or greater than obj. • boolean equals(Object obj) o Parameters: obj – an object to compare against this rational value. o Description: determines whether the content of obj equals this rational value. • public static void main(String args[]) o Parameters: args – a rational of values that specifies.
In this project, a rational number is defined as a fraction in which the numerator and denominator are integers with the denominator being non-zero. Requirements To ensure consistency among solutions each implementation must implement the following requirements. • Your implementation should reflect the definition of a simplified rational number at all times. • A long (integer) is used to represent both the numerator and denominator. • The Rational class must be able to handle both positive and negative numbers. • The rational number must be in simplest form after every operation; that is, 8 6 shall be immediately reduced to 4 3 . In order to simplify a rational number properly, use the the Euclidean Algorithm to determine the greatest common divisor of the two numbers. • All methods that have an object as parameter must be able to handle an input of null. • By definition, the denominator of a fraction cannot be zero since it leads to an undefined value. In Java, this division by zero results in an ArithmeticException; the Rational class must throw an ArithmeticException when division by zero is attempted. • The denominator should be initialized to a sensible and consistent value. The numerator shall be initialized to zero. • The denominator should always be positive leaving the numerator to indicate the sign of the number (positive or negative). • The Rational class shall reside in the default package. Methods There are many methods that one would expect to be supported in a Rational class; unless specified, you will have to implement all of the described methods. Constructors • Rational() o Description: constructs a Rational initializing the value to 0. • Rational(long a) o Parameters: a – the default value for the Rational number. o Description: constructs a Rational initializing the value to 1a . • Rational(long a, long b) throws ArithmeticException o Parameters: a – an integer specifying the initial value of the numerator. b – an integer specifying the initial value of the denominator. o Description: constructs a Rational number initializing the object to a b . Accessors • long getNumerator() o Description: returns the current value of the numerator. o Returns: as an accessor method, it only returns the current value of the numerator. • long getDenominator() o Description: returns the current value of the denominator. o Returns: as an accessor method, it only returns the current value of the denominator. Mathematical Operations With each of the following methods, if the input object r is null, you may treat the input as the value zero (0). • Rational add(Rational r) o Parameters: r – the rational number to be added to this rational. o Description: adds r and this, returning a new object with the reduced sum. A common denominator is required to complete this operation. o Returns: returns a new object with the reduced sum. • Rational subtract(Rational r) o Parameters: r – the rational number to be subtracted from this rational. o Description: subtracts r from this, returning a new object with the reduced difference. A common denominator is required to complete this operation. o Returns: returns a new object with the reduced difference. • Rational multiply(Rational r) o Parameters: r – the rational number to be multiplied with thisrational o Returns: returns a new object with the reduced product. • Rational divide(Rational r) throws ArithmeticException o Parameters: r – the rational number that is to divide this rational. o Description: divides this by r, returning a new object with the reduced quotient. o Returns: returns a new object with the reduced quotient. The Greatest Common Divisor Algorithm • private long gcd(long p, long q) o Parameters: p – an integer. q – an integer. o Description: determines the greatest common divisor of the two input integers according to the Euclidean Algorithm; a quick implementation is easily available online. o Returns: the (positive) GCD of the two input integers. o Notes: It is easier to implement this method if both integers are positive values. Supplied Methods Besides the interface specification described above, there are other methods that have been provided; feel free to use them as required in your implementation and testing. Do not modify these methods. o Returns: a String representation of this rational value. • int compareTo(Object obj) o Parameters: obj – an object to compare against this rational value. o Description: determines whether the content of obj is smaller (or larger) than this rational value; the result returned is compliant with the Comparable interface that the Rational class implements. o Returns: -1, 0, or 1 when this object is less than, equal, or greater than obj. • boolean equals(Object obj) o Parameters: obj – an object to compare against this rational value. o Description: determines whether the content of obj equals this rational value. • public static void main(String args[]) o Parameters: args – a rational of values that specifies.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In this project, a rational number is defined as a fraction in which the numerator and denominator are
integers with the denominator being non-zero.
Requirements
To ensure consistency among solutions each implementation must implement the following requirements.
• Your implementation should reflect the definition of a simplified rational number at all times.
• A long (integer) is used to represent both the numerator and denominator.
• The Rational class must be able to handle both positive and negative numbers.
• The rational number must be in simplest form after every operation; that is, 8 6 shall be
immediately reduced to 4 3 . In order to simplify a rational number properly, use the the Euclidean
Algorithm to determine the greatest common divisor of the two numbers.
• All methods that have an object as parameter must be able to handle an input of null.
• By definition, the denominator of a fraction cannot be zero since it leads to an undefined value. In
Java, this division by zero results in an ArithmeticException; the Rational class
must throw an ArithmeticException when division by zero is attempted.
• The denominator should be initialized to a sensible and consistent value. The numerator shall be
initialized to zero.
• The denominator should always be positive leaving the numerator to indicate the sign of the
number (positive or negative).
• The Rational class shall reside in the default package.
Methods
There are many methods that one would expect to be supported in a Rational class; unless specified,
you will have to implement all of the described methods.
Constructors
• Rational()
o Description: constructs a Rational initializing the value to 0.
• Rational(long a)
o Parameters: a – the default value for the Rational number.
o Description: constructs a Rational initializing the value to 1a .
• Rational(long a, long b) throws ArithmeticException
o Parameters:
a – an integer specifying the initial value of the numerator.
b – an integer specifying the initial value of the denominator.
integers with the denominator being non-zero.
Requirements
To ensure consistency among solutions each implementation must implement the following requirements.
• Your implementation should reflect the definition of a simplified rational number at all times.
• A long (integer) is used to represent both the numerator and denominator.
• The Rational class must be able to handle both positive and negative numbers.
• The rational number must be in simplest form after every operation; that is, 8 6 shall be
immediately reduced to 4 3 . In order to simplify a rational number properly, use the the Euclidean
• All methods that have an object as parameter must be able to handle an input of null.
• By definition, the denominator of a fraction cannot be zero since it leads to an undefined value. In
Java, this division by zero results in an ArithmeticException; the Rational class
must throw an ArithmeticException when division by zero is attempted.
• The denominator should be initialized to a sensible and consistent value. The numerator shall be
initialized to zero.
• The denominator should always be positive leaving the numerator to indicate the sign of the
number (positive or negative).
• The Rational class shall reside in the default package.
Methods
There are many methods that one would expect to be supported in a Rational class; unless specified,
you will have to implement all of the described methods.
Constructors
• Rational()
o Description: constructs a Rational initializing the value to 0.
• Rational(long a)
o Parameters: a – the default value for the Rational number.
o Description: constructs a Rational initializing the value to 1a .
• Rational(long a, long b) throws ArithmeticException
o Parameters:
a – an integer specifying the initial value of the numerator.
b – an integer specifying the initial value of the denominator.
o Description: constructs a Rational number initializing the object to a b .
Accessors
• long getNumerator()
o Description: returns the current value of the numerator.
o Returns: as an accessor method, it only returns the current value of the numerator.
• long getDenominator()
o Description: returns the current value of the denominator.
o Returns: as an accessor method, it only returns the current value of the denominator.
Mathematical Operations
With each of the following methods, if the input object r is null, you may treat the input as the value
zero (0).
• Rational add(Rational r)
o Parameters: r – the rational number to be added to this rational.
o Description: adds r and this, returning a new object with the reduced sum. A common
denominator is required to complete this operation.
o Returns: returns a new object with the reduced sum.
• Rational subtract(Rational r)
o Parameters: r – the rational number to be subtracted from this rational.
o Description: subtracts r from this, returning a new object with the reduced difference.
A common denominator is required to complete this operation.
o Returns: returns a new object with the reduced difference.
• Rational multiply(Rational r)
o Parameters: r – the rational number to be multiplied with thisrational
o Returns: returns a new object with the reduced product.
• Rational divide(Rational r) throws ArithmeticException
o Parameters: r – the rational number that is to divide this rational.
o Description: divides this by r, returning a new object with the reduced quotient.
o Returns: returns a new object with the reduced quotient.
Accessors
• long getNumerator()
o Description: returns the current value of the numerator.
o Returns: as an accessor method, it only returns the current value of the numerator.
• long getDenominator()
o Description: returns the current value of the denominator.
o Returns: as an accessor method, it only returns the current value of the denominator.
Mathematical Operations
With each of the following methods, if the input object r is null, you may treat the input as the value
zero (0).
• Rational add(Rational r)
o Parameters: r – the rational number to be added to this rational.
o Description: adds r and this, returning a new object with the reduced sum. A common
denominator is required to complete this operation.
o Returns: returns a new object with the reduced sum.
• Rational subtract(Rational r)
o Parameters: r – the rational number to be subtracted from this rational.
o Description: subtracts r from this, returning a new object with the reduced difference.
A common denominator is required to complete this operation.
o Returns: returns a new object with the reduced difference.
• Rational multiply(Rational r)
o Parameters: r – the rational number to be multiplied with thisrational
o Returns: returns a new object with the reduced product.
• Rational divide(Rational r) throws ArithmeticException
o Parameters: r – the rational number that is to divide this rational.
o Description: divides this by r, returning a new object with the reduced quotient.
o Returns: returns a new object with the reduced quotient.
The Greatest Common Divisor Algorithm
• private long gcd(long p, long q)
o Parameters:
p – an integer.
q – an integer.
o Description: determines the greatest common divisor of the two input integers according
to the Euclidean Algorithm; a quick implementation is easily available online.
o Returns: the (positive) GCD of the two input integers.
o Notes: It is easier to implement this method if both integers are positive values.
Supplied Methods
Besides the interface specification described above, there are other methods that have been provided; feel
free to use them as required in your implementation and testing. Do not modify these methods.
o Returns: a String representation of this rational value.
• int compareTo(Object obj)
o Parameters: obj – an object to compare against this rational value.
o Description: determines whether the content of obj is smaller (or larger) than this
rational value; the result returned is compliant with the Comparable interface that the
Rational class implements.
o Returns: -1, 0, or 1 when this object is less than, equal, or greater than obj.
• boolean equals(Object obj)
o Parameters: obj – an object to compare against this rational value.
o Description: determines whether the content of obj equals this rational value.
• public static void main(String args[])
o Parameters: args – a rational of values that specifies.
• private long gcd(long p, long q)
o Parameters:
p – an integer.
q – an integer.
o Description: determines the greatest common divisor of the two input integers according
to the Euclidean Algorithm; a quick implementation is easily available online.
o Returns: the (positive) GCD of the two input integers.
o Notes: It is easier to implement this method if both integers are positive values.
Supplied Methods
Besides the interface specification described above, there are other methods that have been provided; feel
free to use them as required in your implementation and testing. Do not modify these methods.
o Returns: a String representation of this rational value.
• int compareTo(Object obj)
o Parameters: obj – an object to compare against this rational value.
o Description: determines whether the content of obj is smaller (or larger) than this
rational value; the result returned is compliant with the Comparable interface that the
Rational class implements.
o Returns: -1, 0, or 1 when this object is less than, equal, or greater than obj.
• boolean equals(Object obj)
o Parameters: obj – an object to compare against this rational value.
o Description: determines whether the content of obj equals this rational value.
• public static void main(String args[])
o Parameters: args – a rational of values that specifies.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
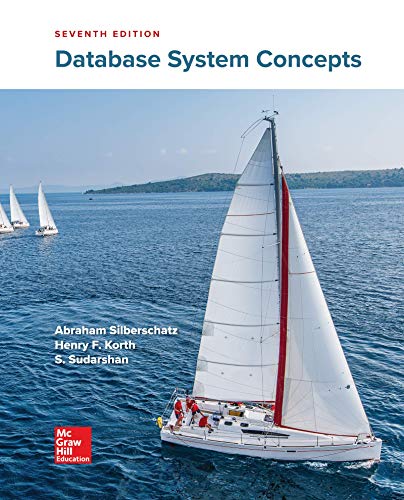
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
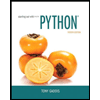
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
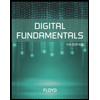
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
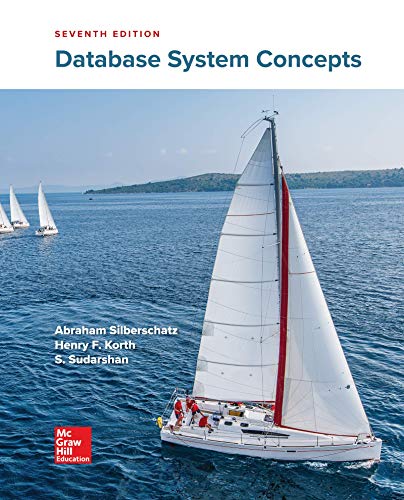
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
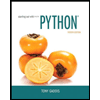
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
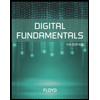
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
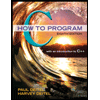
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
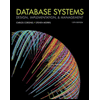
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
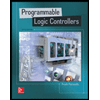
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education