In this lab you will add code to the given java file to produce a Javax.swing GUI very much like the image below in image Use the ‘starter’ file, TestGUICounter.java For this lab, read my pseudocode in the given java files FIRST!! ANALYZE my code carefully. Then start coding the solution. I have given the solution to each lab instructor to help you during the time for your specific lab. PLEASE FINISH THE JAVA CODE AS GUIDED BY THE Slashed LINES import java.awt.*; import java.awt.event.*; import javax.swing.*; public class TestGUICounter { static JFrame frame_1; static JButton start_val, add_1, minus_1, reset_1; static JLabel label_1, label_num; static JTextField text_1; static JPanel panel_center, panel_south; static int iCounter; public static void main(String[] args) { frame_1 = new JFrame(); frame_1.setLayout(new BorderLayout()); panel_center = new JPanel(); panel_south = new JPanel(); panel_center.setLayout(new FlowLayout()); panel_south.setLayout(new FlowLayout()); start_val = new JButton("Start Counter"); add_1 = new JButton("Add 1"); minus_1 = new JButton("Delete 1"); reset_1 = new JButton("Reset"); label_1 = new JLabel("Value is: "); // setup all buttons to use the "ButtonListener()" class for ActionEvents // Put the JtextField, and the two JLabel in the 'panel_center' // and the buttons in the 'panel_south' panels. // add the two panels to the 'frame_1' in the proper // area (CENTER, SOUTH, NORTH, EAST, WEST) frame_1.setSize(450, 200); frame_1.setVisible(true); frame_1.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } private static class ButtonListener implements ActionListener { public void actionPerformed(ActionEvent e) { // write code here to test which of the three buttons // have been clicked using 'e.getSource().equals...' // very similar to the demonstration I did in class lecture // First, when 'start_val' clicked, then get the value from the 'text_1' // text box (user 'getText()' method) and set the 'label_num' and the // iCounter to be that value. Next, in the if 'add_1' clicked, then // add 1 to the 'iCounter' and update label: 'label_num' and if // the 'minus_1' is clicked, delete 1 from iCounter and update // the 'label_num' Then, if 'reset_1' is clicked, set things blank and back to zero. // HINT: when you get the value from a JTextField, it is a string, so // you have to convert it to an integer to give the value to 'iCounter' // and to convert an integer to a string, use 'Integer.parseInt(string variable)' // } } }
In this lab you will add code to the given java file to produce a Javax.swing GUI very much like the image
below in image
Use the ‘starter’ file, TestGUICounter.java
For this lab, read my pseudocode in the given java files FIRST!!
ANALYZE my code carefully.
Then start coding the solution.
I have given the solution to each lab instructor to help you during the time for your specific lab.
PLEASE FINISH THE JAVA CODE AS GUIDED BY THE Slashed LINES
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class TestGUICounter {
static JFrame frame_1;
static JButton start_val, add_1, minus_1, reset_1;
static JLabel label_1, label_num;
static JTextField text_1;
static JPanel panel_center, panel_south;
static int iCounter;
public static void main(String[] args) {
frame_1 = new JFrame();
frame_1.setLayout(new BorderLayout());
panel_center = new JPanel();
panel_south = new JPanel();
panel_center.setLayout(new FlowLayout());
panel_south.setLayout(new FlowLayout());
start_val = new JButton("Start Counter");
add_1 = new JButton("Add 1");
minus_1 = new JButton("Delete 1");
reset_1 = new JButton("Reset");
label_1 = new JLabel("Value is: ");
// setup all buttons to use the "ButtonListener()" class for ActionEvents
// Put the JtextField, and the two JLabel in the 'panel_center'
// and the buttons in the 'panel_south' panels.
// add the two panels to the 'frame_1' in the proper
// area (CENTER, SOUTH, NORTH, EAST, WEST)
frame_1.setSize(450, 200);
frame_1.setVisible(true);
frame_1.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private static class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
// write code here to test which of the three buttons
// have been clicked using 'e.getSource().equals...'
// very similar to the demonstration I did in class lecture
// First, when 'start_val' clicked, then get the value from the 'text_1'
// text box (user 'getText()' method) and set the 'label_num' and the
// iCounter to be that value. Next, in the if 'add_1' clicked, then
// add 1 to the 'iCounter' and update label: 'label_num' and if
// the 'minus_1' is clicked, delete 1 from iCounter and update
// the 'label_num' Then, if 'reset_1' is clicked, set things blank and back to zero.
// HINT: when you get the value from a JTextField, it is a string, so
// you have to convert it to an integer to give the value to 'iCounter'
// and to convert an integer to a string, use 'Integer.parseInt(string variable)'
//
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

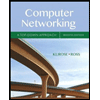
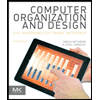
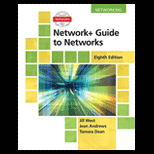
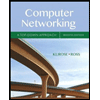
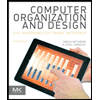
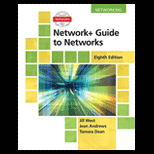
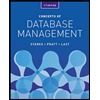
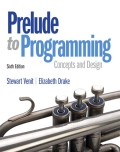
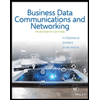