In Checkpoint A, the game will not yet be playable as a two-player game. It will be playable by you in a type of "solitaire mode." Implement these functions from the template following the description (specification) in their docstring: show_top_card() get_top_card() add_card_to_discard() check_racko() find_and_replace() play_hand() The main function to drive the game has been provided. Missing input/output statements are part of play_hand() and left for you to implement, guided by the sample input/output. None of the other functions left to implement use input or print. The case / capitalization of user input should be ignored. On any invalid user input, the program should exit with a non-zero exit code. Hint: Use Python Tutor. as much as possible when testing gameplay. Zybooks requires you specify all your inputs in advance and it is less enjoyable to play that way. Sample input/output 1 When the inputs are: no yes 1 yes 5 yes 7 The expected behavior is (inputs are shown in-line) -------------------------------------------------- Deck: [8, 3, 2] Discard pile: [4] Your current rack is: [5, 1, 7, 6] The top discard card is 4 Do you want it? (yes or no): no The card from the deck is 2 Do you want it? (yes or no): yes Enter the number of the card you want to kick out: 1 Your new rack is: [5, 2, 7, 6] -------------------------------------------------- Deck: [8, 3] Discard pile: [4, 1] Your current rack is: [5, 2, 7, 6] The top discard card is 1 Do you want it? (yes or no): yes Enter the number of the card you want to kick out: 5 Your new rack is: [1, 2, 7, 6] -------------------------------------------------- Deck: [8, 3] Discard pile: [4, 5] Your current rack is: [1, 2, 7, 6] The top discard card is 5 Do you want it? (yes or no): yes Enter the number of the card you want to kick out: 7 Your new rack is: [1, 2, 5, 6] You got Racko-O! Sample input/output 2 When the inputs are: YeS 10 The expected behavior is (inputs are shown in-line) -------------------------------------------------- Deck: [8, 3, 2] Discard pile: [4] Your current rack is: [5, 1, 7, 6] The top discard card is 4 Do you want it? (yes or no): YeS Enter the number of the card you want to kick out: 10 Error: invalid card. Sample input/output 3 When the inputs are: NO Maybe The expected behavior is (inputs are shown in-line) -------------------------------------------------- Deck: [8, 3, 2] Discard pile: [4] Your current rack is: [5, 1, 7, 6] The top discard card is 4 Do you want it? (yes or no): NO The card from the deck is 2 Do you want it? (yes or no): Maybe Error: choice can be only yes or no.
In Checkpoint A, the game will not yet be playable as a two-player game. It will be playable by you in a type of "solitaire mode."
Implement these functions from the template following the description (specification) in their docstring:
- show_top_card()
- get_top_card()
- add_card_to_discard()
- check_racko()
- find_and_replace()
- play_hand()
The main function to drive the game has been provided.
- Missing input/output statements are part of play_hand() and left for you to implement, guided by the sample input/output.
- None of the other functions left to implement use input or print.
- The case / capitalization of user input should be ignored.
- On any invalid user input, the program should exit with a non-zero exit code.
Hint: Use Python Tutor. as much as possible when testing gameplay. Zybooks requires you specify all your inputs in advance and it is less enjoyable to play that way.
Sample input/output 1
When the inputs are:
no
yes
1
yes
5
yes
7
The expected behavior is (inputs are shown in-line)
--------------------------------------------------
Deck: [8, 3, 2]
Discard pile: [4]
Your current rack is: [5, 1, 7, 6]
The top discard card is 4
Do you want it? (yes or no): no
The card from the deck is 2
Do you want it? (yes or no): yes
Enter the number of the card you want to kick out: 1
Your new rack is: [5, 2, 7, 6]
--------------------------------------------------
Deck: [8, 3]
Discard pile: [4, 1]
Your current rack is: [5, 2, 7, 6]
The top discard card is 1
Do you want it? (yes or no): yes
Enter the number of the card you want to kick out: 5
Your new rack is: [1, 2, 7, 6]
--------------------------------------------------
Deck: [8, 3]
Discard pile: [4, 5]
Your current rack is: [1, 2, 7, 6]
The top discard card is 5
Do you want it? (yes or no): yes
Enter the number of the card you want to kick out: 7
Your new rack is: [1, 2, 5, 6]
You got Racko-O!
Sample input/output 2
When the inputs are:
YeS
10
The expected behavior is (inputs are shown in-line)
--------------------------------------------------
Deck: [8, 3, 2]
Discard pile: [4]
Your current rack is: [5, 1, 7, 6]
The top discard card is 4
Do you want it? (yes or no): YeS
Enter the number of the card you want to kick out: 10
Error: invalid card.
Sample input/output 3
When the inputs are:
NO
Maybe
The expected behavior is (inputs are shown in-line)
--------------------------------------------------
Deck: [8, 3, 2]
Discard pile: [4]
Your current rack is: [5, 1, 7, 6]
The top discard card is 4
Do you want it? (yes or no): NO
The card from the deck is 2
Do you want it? (yes or no): Maybe
Error: choice can be only yes or no.
![1 def show_top_card (cards):
2
3
4
5
7
8
9
10
11
12
46
47
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
13 def get_top_card(cards):
14
15
16
17
18
19
20
21
84
85
86
87
88
89
90
91
92
93
94
95
"""Reveals the top card of a stack, without changing the stack.
Parameters:
108
199
110
111
112
113
114
115
116
117
118
119
120
121
122
123
Returns:
124
125
126
127
pass
22
23
24
25 def add_card_to_discard (card, discard):
26
27
28
29
30
31
32
33
34
35
36
37 def check_racko(rack):
38
39
40
41
42
43
44
45
A card (or None if the stack is empty)
"""Removes the top card of a stack and returns it.
cards (list): stack of cards
Parameters:
Returns:
pass
A card (or None if the stack is empty)
cards (list): stack of cards
"""Adds a card to the top of the discard pile.
Parameters:
pass
card (int): a card
discard (list): stack of cards
Returns: None
www
48 def find_and_replace(newCard, cardToBeReplaced, rack, discard):
"""Takes in a new card and finds the card that needs to
49
50
be replaced and replaces it
51
52
53
54
55
56
57
"""Checks if Rack-0 has been achieved.
Parameters:
pass
Returns: True if "Racko-0", otherwise False
rack (list): ordered hand of cards
pass
Parameters:
newCard (int): a card
cardToBeReplaced (int): the card from the hand to be replaced
rack (list): ordered hand of cards
discard (list): stack of cards
Returns: True if successful, otherwise False
def play_hand (rack, deck, discard):
"*"plays a single hand of Racko. See input/output in documentation.
Parameters:
rack (list): the player's hand
deck (list): the deck used to draw new cards
discard (list): the deck used for the discard pile
Returns:
True if Racko is achieved, otherwise False
# show the top card in the discard
#print it for the user
#ask the user if they want it
# if they said 'yes' / I want the discard
96 if __name__ == '__main__
97
98
99
100
101
102
103
104
105
106
107
# get the top card from the discard
# ask the user for the card (number) they want to kick out
#modify the user's rack accordingly
#else, if they said 'no' / I don't want the discard
# get the top card from deck
#print it for the user
#ask the user if they want it
# if they said 'yes' / I want the draw card
#ask the user for the card (number) they want to kick out
#modify the user's rack accordingly
#else, if they said 'no' / I don't want the draw card
# add the draw card to the discard
# otherwise (not 'yes' or 'no') error and exit
# otherwise (not 'yes' or 'no') error and exit
pass
"""Plays a hand of Racko interactively, with a small sample deck"""
# A draw deck, for testing
deck [8, 3, 2, 4]
# A rack for the user, for testing
my_hand= [5, 1, 7, 6]
# An empty discard pile
discard = []
# To start, take thwe top of the deck and out it on the discard
discard_card= get_top_card(deck)
add_card_to_discard (discard_card, discard)
while True:
print('-'*50)
print('Deck:', deck)
print('Discard pile:', discard)
print('Your current rack is:', my_hand)
play_hand (my_hand, deck, discard)
print('Your new rack is:', my_hand)
if len(deck) == 0:
print("\nWOAH! Deck is empty. Exiting")
exit (1)
elif (check_racko (my_hand) == True):
print("\nYou got Racko-0!")
exit(0)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4d8acdf6-9c5e-42f8-ad34-e57535beff59%2Fbd34bf9a-d3ef-4015-b386-fbe044a0b018%2F1ab21fr_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

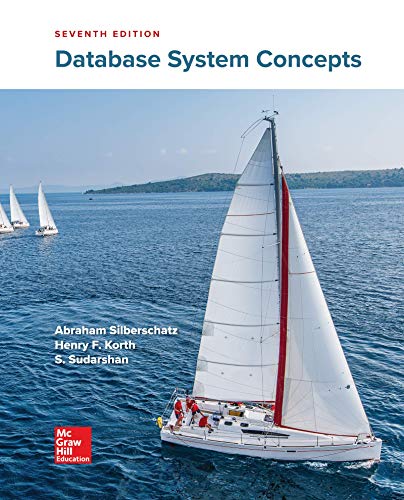
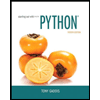
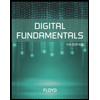
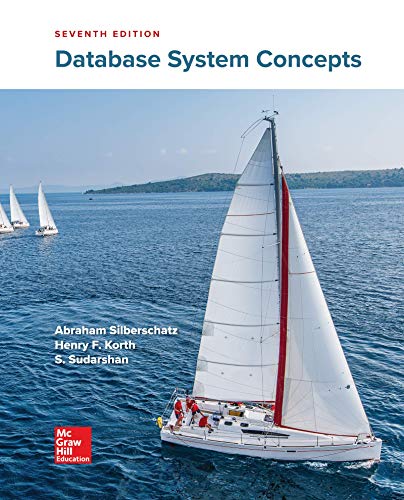
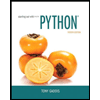
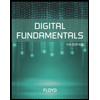
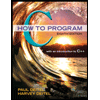
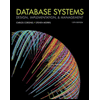
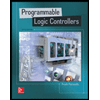