in C# Write a program named InputMethodDemo2 that eliminates the repetitive code in the InputMethod() in the InputMethodDemo program in Figure 8-5. Rewrite the program so the InputMethod() contains only two statements: one = DataEntry("first"); two = DataEntry("second"); I am getting the error Method DataEntry is defined to eliminate repetitive code 0 out of 1 checks passed. unit Test Incomplete Method DataEntry prompts the user to enter an integer and returns the integer Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTest37b77fb0.cs(21,47): error CS0234: The type or namespace name `DataEntry' does not exist in the namespace `InputMethodDemo2'. Are you missing an assembly reference? Test Contents [TestFixture] public class DataEntryMethodTest { [Test ] public void DataEntryTest() { string consoleInput = "97"; int returnedValue; string expectedString = "Enter third integer"; using (var inputs = new StringReader(consoleInput)) { Console.SetIn(inputs); using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); returnedValue = InputMethodDemo2.DataEntry("third"); Assert.AreEqual(expectedString, sw.ToString().Trim()); Assert.AreEqual(97, returnedValue); } } } } the pic is the starter code and this is my code using System; namespace InputMethodDemo2 { class Program { static void Main(string[] args) { int one, two; one = DataEntry("first"); two = DataEntry("second"); Console.WriteLine("You entered " + one + " and " + two); } static int DataEntry(string message) { Console.Write("Enter " + message + " integer: "); return int.Parse(Console.ReadLine()); } } }
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
in C#
Write a
Rewrite the program so the InputMethod() contains only two statements:
one = DataEntry("first");
two = DataEntry("second");
I am getting the error
Method DataEntry is defined to eliminate repetitive code
0 out of 1 checks passed.
Method DataEntry prompts the user to enter an integer and returns the integer


Below is the complete solution with explanation in detail about the error and the solution to fix the error for the given question in C# Programming Language.
Step by step
Solved in 2 steps

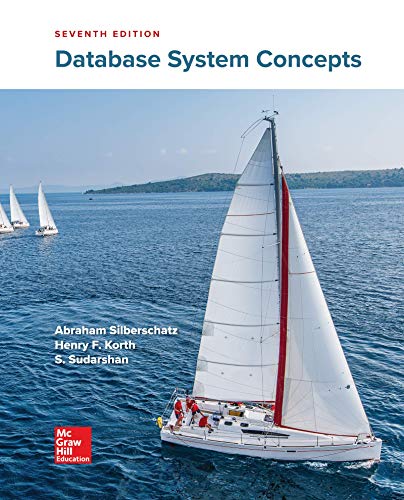
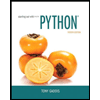
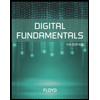
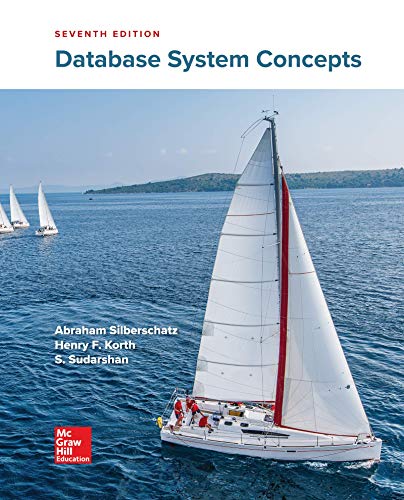
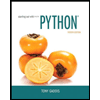
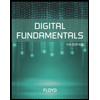
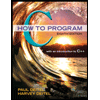
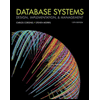
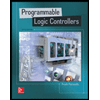