İn C Language pls help #include #include #include
İn C Language pls help
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct ArrayList_s
{
void **list;
int size;
int capacity;
int delta_capacity;
} ArrayList_t, *ArrayList;
ArrayList arraylist_create(int capacity, int delta_capacity)
{
ArrayList l;
l = (ArrayList)malloc(sizeof(ArrayList_t));
if (l != NULL)
{
l->list = (void **)malloc(capacity * sizeof(void *));
if (l->list != NULL)
{
l->size = 0;
l->capacity = capacity;
l->delta_capacity = delta_capacity;
}
else
{
free(l);
l = NULL;
}
}
return l;
}
void arraylist_destroy(ArrayList l)
{
free(l->list);
free(l);
}
int arraylist_isempty(ArrayList l)
{
return l->size == 0;
}
int arraylist_isfull(ArrayList l)
{
return l->size == l->capacity;
}
int arraylist_set(ArrayList l, void *e, int index, void **replaced)
{
if (!arraylist_isfull(l))
{
if (index < 0 && index > l->size)
{
index = l->size;
}
else
{
if (replaced != NULL)
{
*replaced = l->list[index];
}
}
if (index == l->size)
{
l->size = l->size + 1;
}
l->list[index] = e;
}
else
{
index = -index;
}
return index;
}
int arraylist_insert(ArrayList l, void *e, int index)
{
void **nlist;
int ncapacity;
if (index >= 0 && index <= l->size)
{
if (arraylist_isfull(l))
{
ncapacity = l->capacity + l->delta_capacity;
nlist = realloc(l->list, ncapacity * sizeof(void *));
if (nlist != NULL)
{
//shift elements between index and l->size-1 to right by 1
memmove(&nlist[index] + 1, &nlist[index], (l->size - index) * sizeof(void *));
l->list = nlist;
l->capacity = ncapacity;
l->size++;
l->list[index] = e;
}
else
{
nlist = (void **)malloc(ncapacity * sizeof(void *));
if (nlist != NULL)
{
//copy elements while shifting elements between index and l->size-1 to right by 1
memcpy(nlist, l->list, index * sizeof(void *));
memcpy(&nlist[index] + 1, &l->list[index], (l->size - index) * sizeof(void *));
free(l->list);
l->list = nlist;
l->capacity = ncapacity;
l->size++;
l->list[index] = e;
}
else
{
index = -index;
}
}
}
else
{
//shift elements between index and l->size-1 to right by 1
memmove(&l->list[index] + 1, &l->list[index], (l->size - index) * sizeof(void *));
l->size++;
l->list[index] = e;
}
}
else
{
index = -index;
}
return index;
}
void *arraylist_delete(ArrayList l, int index)
{
/*
define *e, ncapacity, **nlist
e <- null
if l is not empty and index >= 0 and index < size of l then
e <- l->list[index]
decrease l->size once
shift elements between index + 1 to left by 1
ncapacity <- capacity of l / 2
if size of l <= ncapacity and ncapacity > 0 then
nlist <- realloc(list of l, ncapacity * size of (void *))
if nlist not null then
list of l <- nlist
capacity of l <- ncapacity
else
nlist <- (void **)malloc(ncapacity * size of (void *))
if nlist not null then
memcpy(nlist, list of l, size of l * size of (void *))
free list of l
list of l <- nlist
capacity of l <- ncapacity
endif
endif
endif
return e
*/
}
int main()
{
return 0;
}

Step by step
Solved in 2 steps

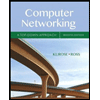
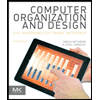
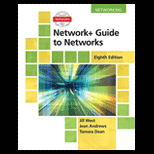
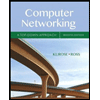
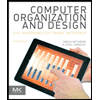
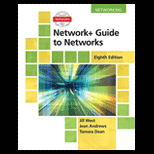
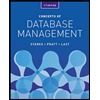
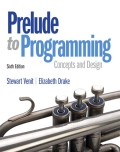
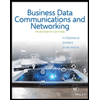