Implement a simple e-mail messaging system. I have already designed a Message class and a Mailbox/MailboxTest class. A message has a recipient, a sender, and a message text. A Mailbox can store and manipulate messages and tell the user how many they have. Supply a number of mailboxes for different users and a user interface(GUI) for the user to login, send messages to other users, read their own messages, and log out. Please explain each thing you do. Here is my Message class: publicclass Message { private String recipient; private String sender; private String messageBody; public Message(Stringsender, String recipient) { this.sender =sender; this.recipient =recipient; this.messageBody =""; } public void append(String text) { this.messageBody +=text +"\n"; } public String toString() { return "From: " + this.sender+ "\n" + "To: " + this.recipient + "\n" + "Message: " + this.messageBody; } } Here is Mailbox class: import java.util.ArrayList; publicclass Mailbox{ private ArrayList messages; public Mailbox() { this.messages = newArrayList(); } public void addMessage(Message m) { this.messages.add(m); } public Message getMessage(int i) { returnthis.messages.get(i); } public void removeMessage(int i) { this.messages.remove(i); } public int mailBoxSize() { returnthis.messages.size(); } } MailBoxTest class: publicclass MailboxTest { public static voidmain(String[] args) { Mailbox mailbox =new Mailbox(); Message message1 =new Message("Bob Frank", "Steve Smith"); message1.append("Hello Bob, how are you? "); mailbox.addMessage(message1); Message message2 =new Message("Steve Smith", "Bob Frank"); message2.append("Hello Steve, I am good. "); mailbox.addMessage(message2); System.out.println(mailbox.getMessage(0).toString()); System.out.println(mailbox.getMessage(1).toString()); System.out.println("Mailbox size = " +mailbox.mailBoxSize()+ " after adding both messages"); mailbox.removeMessage(1); System.out.println("Removed message2"); System.out.println("Mailbox size = " +mailbox.mailBoxSize()+ " after removing message2 \n"); System.out.println(mailbox.getMessage(0).toString()); } }
Implement a simple e-mail messaging system.
I have already designed a Message class and a Mailbox/MailboxTest class. A message has a recipient, a sender, and a message text. A Mailbox can store and manipulate messages and tell the user how many they have.
Supply a number of mailboxes for different users and a user interface(GUI) for the user to login, send messages to other users, read their own messages, and log out.
Please explain each thing you do.
Here is my Message class:
publicclass Message
{
private String recipient;
private String sender;
private String messageBody;
public Message(Stringsender, String recipient) {
this.sender =sender;
this.recipient =recipient;
this.messageBody ="";
}
public void append(String text) {
this.messageBody +=text +"\n";
}
public String toString() {
return "From: " + this.sender+ "\n" + "To: " + this.recipient + "\n" + "Message: " + this.messageBody;
}
}
Here is Mailbox class:
import java.util.ArrayList;
publicclass Mailbox{
private ArrayList<Message> messages;
public Mailbox() {
this.messages = newArrayList<Message>();
}
public void addMessage(Message m) {
this.messages.add(m);
}
public Message getMessage(int i) {
returnthis.messages.get(i);
}
public void removeMessage(int i) {
this.messages.remove(i);
}
public int mailBoxSize()
{
returnthis.messages.size();
}
}
MailBoxTest class:
publicclass MailboxTest
{
public static voidmain(String[] args) {
Mailbox mailbox =new Mailbox();
Message message1 =new Message("Bob Frank", "Steve Smith");
message1.append("Hello Bob, how are you? ");
mailbox.addMessage(message1);
Message message2 =new Message("Steve Smith", "Bob Frank");
message2.append("Hello Steve, I am good. ");
mailbox.addMessage(message2);
System.out.println(mailbox.getMessage(0).toString());
System.out.println(mailbox.getMessage(1).toString());
System.out.println("Mailbox size = " +mailbox.mailBoxSize()+ " after adding both messages");
mailbox.removeMessage(1);
System.out.println("Removed message2");
System.out.println("Mailbox size = " +mailbox.mailBoxSize()+ " after removing message2 \n");
System.out.println(mailbox.getMessage(0).toString());
}
}

Step by step
Solved in 5 steps

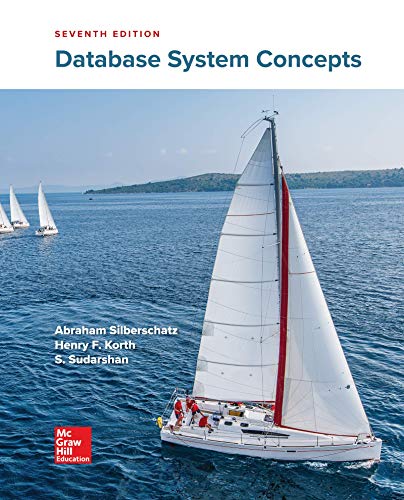
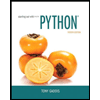
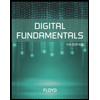
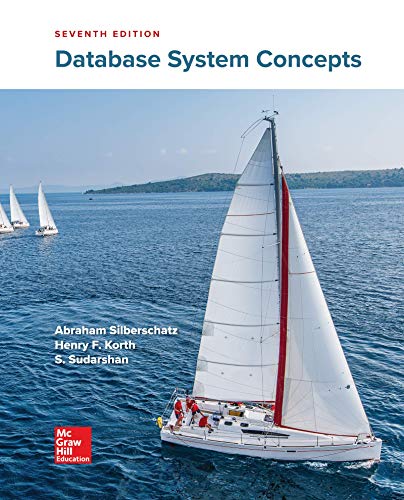
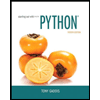
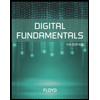
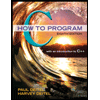
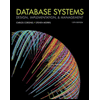
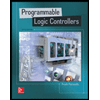