I attached the instructions below, but I have no idea what this question is asking. I don't know what exactly is being tested for equality, less than, or greater than.
I attached the instructions below, but I have no idea what this question is asking. I don't know what exactly is being tested for equality, less than, or greater than.
"""
Project 9.1
Resources to manage a student's name and test scores.
Includes methods for comparisons and testing for equality.
"""
class Student(object):
"""Represents a student."""
def __init__(self, name, number):
"""All scores are initially 0."""
self.name = name
self.scores = []
for count in range(number):
self.scores.append(0)
def getName(self):
"""Returns the student's name."""
return self.name
def setScore(self, i, score):
"""Resets the ith score, counting from 1."""
self.scores[i - 1] = score
def getScore(self, i):
"""Returns the ith score, counting from 1."""
return self.scores[i - 1]
def getAverage(self):
"""Returns the average score."""
return sum(self.scores) / len(self._scores)
def getHighScore(self):
"""Returns the highest score."""
return max(self.scores)
def __str__(self):
"""Returns the string representation of the student."""
return "Name: " + self.name + "\nScores: " + \
" ".join(map(str, self.scores))
# Write method definitions here
def __eq__(self):
pass
def __lt__(self):
pass
def __ge__(self):
pass
def main():
"""A simple test."""
student = Student("Ken", 5)
print(student)
for i in range(1, 6):
student.setScore(i, 100)
print(student)
if __name__ == "__main__":
main()


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

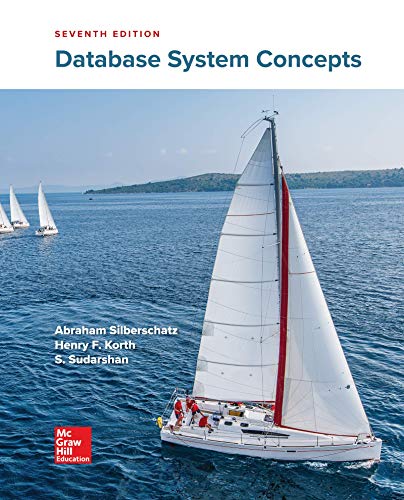
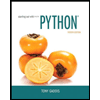
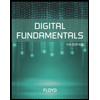
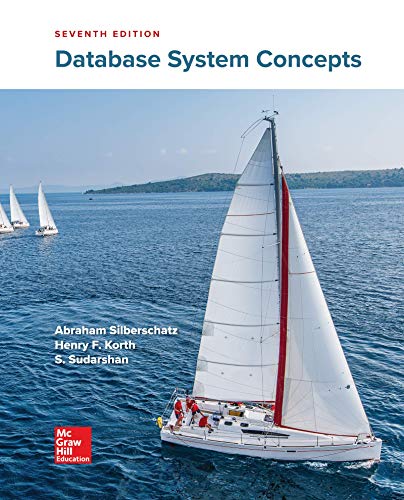
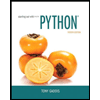
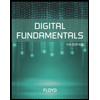
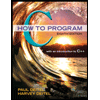
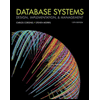
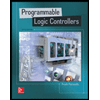