How to fix this code to work... from tkinter import * from tkinter import ttk from tkinter import messagebox class Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.major = m list_of_student = [] window = Tk() window.geometry("800x750") window.title("Simple Student Management System") list_of_students = [] id = 1001 def add_student(): global list_of_students global id if FirstnameEntry.get() =='': messagebox.showerror('erorr', "First name is required") return if LastnameEntry.get() =='': messagebox.showerror('erorr', "Last name is required") return if DateofbirthEntry.get() =='': messagebox.showerror('erorr', "Date of Birth is required") return if MajorEntry.get() =='': instance = Student(id, FirstnameEntry.get(), LastnameEntry.get(), DateofbirthEntry.get()) else: instance = Student(id, FirstnameEntry.get(), LastnameEntry.get(), DateofbirthEntry.get(), MajorEntry.get()) list_of_students.append(instance) messagebox.showinfo("Information", "Student added") id += 1 FirstnameEntry.delete(0, END) LastnameEntry.delete(0, END) DateofbirthEntry.delete(0, END) MajorEntry.delete(0, END) def viewAll(): global list_of_students t.delete("1.0", END) for obj in list_of_students: t.insert(END, Student.print_student_info()) t.insert(END, '\n') if len(list_of_students) ==0: messagebox.showerror("error", "No Student Found") def search_student(): found = False for student in list_of_students: if SearchbyNameEntry.get() == Student.first_name or SearchbyNameEntry.get() == Student.last_name: found = True t.insert(END, Student.print_student_info()) if (found==False): messagebox.showerror("error", "No Student Found") def remove_student(): found = False for student in list_of_students: if RemovebyNameEntry.get() ==str(student.id): found = True list_of_students.remove(student) if (found==False): messagebox.showerror("error", "No Student Found") else: messagebox.showinfo("Information", "Student Removed") Label(text = "First Name").grid(row=1, column = 0, padx=90) Label(text = "Last Name").grid(row=2, column = 0) Label(text = "Date of Birth").grid(row=3, column = 0) Label(text = "Major").grid(row=4, column = 0) FirstnameValue = StringVar() LastnameValue = StringVar() DateofbirthValue = StringVar() MajorValue = StringVar() SearchbyNameValue = StringVar() RemovebyNameValue = StringVar() ViewallValue = StringVar() FirstnameEntry = Entry(window, textvariable=FirstnameValue) LastnameEntry = Entry(window, textvariable=LastnameValue) DateofbirthEntry = Entry(window, textvariable=DateofbirthValue) MajorEntry = Entry(window, textvariable=MajorValue) SearchbyNameEntry = Entry(window, textvariable=SearchbyNameValue) RemovebyNameEntry = Entry(window, textvariable=RemovebyNameValue) ViewallEntry=Entry(window, textvariable=ViewallValue) FirstnameEntry.grid(row=1, column=1, pady=10) LastnameEntry.grid(row=2, column=1, pady=10) DateofbirthEntry.grid(row=3, column=1, pady=10) MajorEntry.grid(row=4, column=1, pady=10) b1= Button(text="Add Student", command= add_student) b1.grid(row=5, column =1, pady=10) ttk.Separator(window, orient=HORIZONTAL).grid(row=7, columnspan=3, ipadx=400, pady=10) b2 = Button(text="Search by name", command=search_student) b2.grid(row=8, column=0, pady=5, padx=0) SearchbyNameEntry.grid(row=8, column=0, columnspan=2, pady=5) b3 = Button(text="View All", command=viewAll) b3.grid(row=8, column=1, pady=5) t = Text(window, width=70, height=30) t.grid(column=0, row=9, columnspan=2, padx=20) ttk.Separator(window, orient=HORIZONTAL).grid(row=50, columnspan=3, ipadx=400, pady=10) b4 = Button(text="Remove ID", command=remove_student) b4.grid(row=52, column=0, pady=5, padx=0) b4.grid(row=52, column=0, columnspan=2, pady=5) window.mainloop()
How to fix this code to work...
from tkinter import *
from tkinter import ttk
from tkinter import messagebox
class Student:
def __init__(self, id, fn, ln, dob, m='undefined'):
self.id = id
self.firstName = fn
self.lastName = ln
self.dateOfBirth = dob
self.major = m
list_of_student = []
window = Tk()
window.geometry("800x750")
window.title("Simple Student Management System")
list_of_students = []
id = 1001
def add_student():
global list_of_students
global id
if FirstnameEntry.get() =='':
messagebox.showerror('erorr', "First name is required")
return
if LastnameEntry.get() =='':
messagebox.showerror('erorr', "Last name is required")
return
if DateofbirthEntry.get() =='':
messagebox.showerror('erorr', "Date of Birth is required")
return
if MajorEntry.get() =='':
instance = Student(id, FirstnameEntry.get(), LastnameEntry.get(), DateofbirthEntry.get())
else:
instance = Student(id, FirstnameEntry.get(), LastnameEntry.get(), DateofbirthEntry.get(), MajorEntry.get())
list_of_students.append(instance)
messagebox.showinfo("Information", "Student added")
id += 1
FirstnameEntry.delete(0, END)
LastnameEntry.delete(0, END)
DateofbirthEntry.delete(0, END)
MajorEntry.delete(0, END)
def viewAll():
global list_of_students
t.delete("1.0", END)
for obj in list_of_students:
t.insert(END, Student.print_student_info())
t.insert(END, '\n')
if len(list_of_students) ==0:
messagebox.showerror("error", "No Student Found")
def search_student():
found = False
for student in list_of_students:
if SearchbyNameEntry.get() == Student.first_name or SearchbyNameEntry.get() == Student.last_name:
found = True
t.insert(END, Student.print_student_info())
if (found==False):
messagebox.showerror("error", "No Student Found")
def remove_student():
found = False
for student in list_of_students:
if RemovebyNameEntry.get() ==str(student.id):
found = True
list_of_students.remove(student)
if (found==False):
messagebox.showerror("error", "No Student Found")
else:
messagebox.showinfo("Information", "Student Removed")
Label(text = "First Name").grid(row=1, column = 0, padx=90)
Label(text = "Last Name").grid(row=2, column = 0)
Label(text = "Date of Birth").grid(row=3, column = 0)
Label(text = "Major").grid(row=4, column = 0)
FirstnameValue = StringVar()
LastnameValue = StringVar()
DateofbirthValue = StringVar()
MajorValue = StringVar()
SearchbyNameValue = StringVar()
RemovebyNameValue = StringVar()
ViewallValue = StringVar()
FirstnameEntry = Entry(window, textvariable=FirstnameValue)
LastnameEntry = Entry(window, textvariable=LastnameValue)
DateofbirthEntry = Entry(window, textvariable=DateofbirthValue)
MajorEntry = Entry(window, textvariable=MajorValue)
SearchbyNameEntry = Entry(window, textvariable=SearchbyNameValue)
RemovebyNameEntry = Entry(window, textvariable=RemovebyNameValue)
ViewallEntry=Entry(window, textvariable=ViewallValue)
FirstnameEntry.grid(row=1, column=1, pady=10)
LastnameEntry.grid(row=2, column=1, pady=10)
DateofbirthEntry.grid(row=3, column=1, pady=10)
MajorEntry.grid(row=4, column=1, pady=10)
b1= Button(text="Add Student", command= add_student)
b1.grid(row=5, column =1, pady=10)
ttk.Separator(window, orient=HORIZONTAL).grid(row=7, columnspan=3, ipadx=400, pady=10)
b2 = Button(text="Search by name", command=search_student)
b2.grid(row=8, column=0, pady=5, padx=0)
SearchbyNameEntry.grid(row=8, column=0, columnspan=2, pady=5)
b3 = Button(text="View All", command=viewAll)
b3.grid(row=8, column=1, pady=5)
t = Text(window, width=70, height=30)
t.grid(column=0, row=9, columnspan=2, padx=20)
ttk.Separator(window, orient=HORIZONTAL).grid(row=50, columnspan=3, ipadx=400, pady=10)
b4 = Button(text="Remove ID", command=remove_student)
b4.grid(row=52, column=0, pady=5, padx=0)
b4.grid(row=52, column=0, columnspan=2, pady=5)
window.mainloop()

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

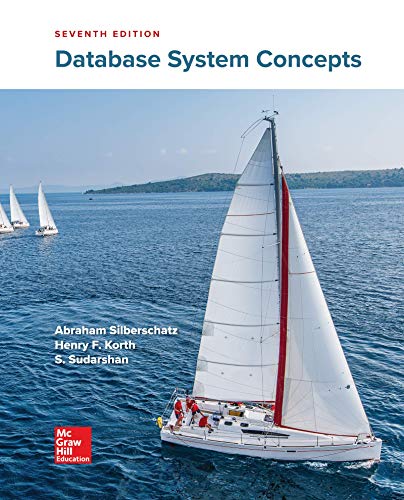
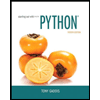
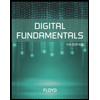
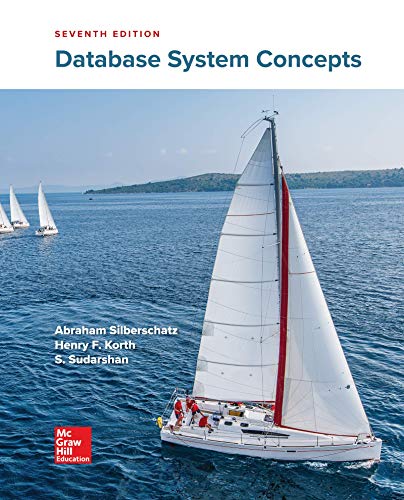
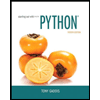
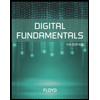
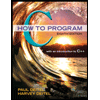
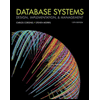
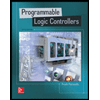