hey, copy this code put it in a compiler, debug its a code was java but converted to c++, i want this to be in and for c++ homework, please help me it wont take time from you. theres just 10 errors idont know how to fix. #include #include #include class Student{ std::wstring studentName; int studentNum = 0; std::wstring SSN; double GPA = 0; double totalCredit = 0; Student(const std::wstring &studentName, int studentNum, const std::wstring &SSN, double GPA, double totalCredit); bool isequal(Student *student2); void addGpa(double amount); }; } { class Course { std::wstring courseName; int courseNum = 0; double creditHour = 0; std::vector array; int counter = 0; Course(const std::wstring &courseName, int courseNum, double creditHour, int registeringStudents); void addStudent(Student *student); bool validate(Student *student); Student *getStudentByStno(int studentNum); std::vector getStudentByGPA(double gpa); double findMaxGpa(); }; ; } { class main { public: static void main(std::vector &args); }; } int main(int argc, char **argv) { std::vector args(argv + 1, argv + argc); javaapplication4::main::main(args); } { Student::Student(const std::wstring &studentName, int studentNum, const std::wstring &SSN, double GPA, double totalCredit) { this->studentName = studentName; this->studentNum = studentNum; this->SSN = SSN; this->GPA = GPA; this->totalCredit = totalCredit; } bool Student::isequal(Student *student2) { if (this->GPA == student2->GPA && this->totalCredit == student2->totalCredit) { return true; } else { return false; } } void Student::addGpa(double amount) { if (amount + this->GPA < 4.0 && amount + this->GPA > 0.35) { this->GPA += amount; } else { std::wcout << L"Result is more then 4.0 or less then 0.35... please try a different amount" << std::endl; } } } { using ArrayList = java::util::ArrayList; Course::Course(const std::wstring &courseName, int courseNum, double creditHour, int registeringStudents) { this->courseName = courseName; this->courseNum = courseNum; this->creditHour = creditHour; this->array = std::vector(registeringStudents); } void Course::addStudent(Student *student) { if (this->array.size() == counter) { std::wcout << L"Failed... course is full" << std::endl; } else if (validate(student)) { this->array[counter] = student; counter++; std::wcout << L"Success in adding student" << std::endl; } else { std::wcout << L"student number already registered" << std::endl; } } bool Course::validate(Student *student) { for (int i = 0; i < this->array.size(); i++) { if (array[i] == nullptr) { continue; } if (student->studentNum == this->array[i]->studentNum) { return false; } } return true; } Student *Course::getStudentByStno(int studentNum) { for (int i = 0; i < array.size(); i++) { if (studentNum == array[i]->studentNum) { std::wcout << L"found and returned" << std::endl; return array[i]; } } std::wcout << L"not found.. returned null" << std::endl; return nullptr; } std::vector Course::getStudentByGPA(double gpa) { std::vector temp(counter); int tempo = 0; for (int i = 0; i < array.size(); i++) { if (array[i] == nullptr) { continue; } if (gpa == array[i]->GPA) { temp[tempo] = array[i]; tempo++; } } return temp; } double Course::findMaxGpa() { double max = 0; for (int i = 0; i < array.size(); i++) { if (array[i] == nullptr) { continue; } if (array[i]->GPA > max) { max = array[i]->GPA; } } return max; } } { void main::main(std::vector &args) { Student *student1 = new Student(L"Saif", 01, L"02323123", 2.0, 3.0); Student *student2 = new Student(L"abdulla", 02, L"1231232", 1.0, 3.0); std::wcout << student1->isequal(student2) << std::endl; Student *student3 = new Student(L"hazem", 01, L"02323123", 3.0, 3.0); Student *student4 = new Student(L"khalid", 04, L"34534535", 3.0, 3.0); std::wcout << student3->isequal(student4) << std::endl; Course *science = new Course(L"science", 01, 3.0, 10); science->addStudent(student1); science->addStudent(student2); science->addStudent(student3); science->addStudent(student4); std::wcout << L"student 1 gpa is: " << student1->GPA << std::endl; student1->addGpa(5.0); std::wcout << L"student 1 gpa is: " << student1->GPA << std::endl; student1->addGpa(0.5); std::wcout << L"student 1 gpa is: " << student1->GPA << std::endl; std::wcout << L"The pointer for student 1 is : " << student1 << std::endl; std::wcout << science->getStudentByStno(01) << std::endl; std::vector array2 = science->getStudentByGPA(3.0); std::wcout << array2[0]->studentName << std::endl; std::wcout << science->findMaxGpa() << std::endl; Student *student5 = new Student(L"smartest", 05, L"24234234", 4.0, 3.0); science->addStudent(student5); std::wcout << science->findMaxGpa() << std::endl; delete science; }
hey, copy this code put it in a compiler, debug its a code was java but converted to c++, i want this to be in and for c++ homework, please help me it wont take time from you. theres just 10 errors idont know how to fix.
#include <string>
#include <
#include <iostream>
class Student{
std::wstring studentName;
int studentNum = 0;
std::wstring SSN;
double GPA = 0;
double totalCredit = 0;
Student(const std::wstring &studentName, int studentNum, const std::wstring &SSN, double GPA, double totalCredit);
bool isequal(Student *student2);
void addGpa(double amount);
};
}
{
class Course
{
std::wstring courseName;
int courseNum = 0;
double creditHour = 0;
std::vector<Student*> array;
int counter = 0;
Course(const std::wstring &courseName, int courseNum, double creditHour, int registeringStudents);
void addStudent(Student *student);
bool validate(Student *student);
Student *getStudentByStno(int studentNum);
std::vector<Student*> getStudentByGPA(double gpa);
double findMaxGpa();
};
;
}
{
class main
{
public:
static void main(std::vector<std::wstring> &args);
};
}
int main(int argc, char **argv)
{
std::vector<std::wstring> args(argv + 1, argv + argc);
javaapplication4::main::main(args);
}
{
Student::Student(const std::wstring &studentName, int studentNum, const std::wstring &SSN, double GPA, double totalCredit)
{
this->studentName = studentName;
this->studentNum = studentNum;
this->SSN = SSN;
this->GPA = GPA;
this->totalCredit = totalCredit;
}
bool Student::isequal(Student *student2)
{
if (this->GPA == student2->GPA && this->totalCredit == student2->totalCredit)
{
return true;
}
else
{
return false;
}
}
void Student::addGpa(double amount)
{
if (amount + this->GPA < 4.0 && amount + this->GPA > 0.35)
{
this->GPA += amount;
}
else
{
std::wcout << L"Result is more then 4.0 or less then 0.35... please try a different amount" << std::endl;
}
}
}
{
using ArrayList = java::util::ArrayList;
Course::Course(const std::wstring &courseName, int courseNum, double creditHour, int registeringStudents)
{
this->courseName = courseName;
this->courseNum = courseNum;
this->creditHour = creditHour;
this->array = std::vector<Student*>(registeringStudents);
}
void Course::addStudent(Student *student)
{
if (this->array.size() == counter)
{
std::wcout << L"Failed... course is full" << std::endl;
}
else if (validate(student))
{
this->array[counter] = student;
counter++;
std::wcout << L"Success in adding student" << std::endl;
}
else
{
std::wcout << L"student number already registered" << std::endl;
}
}
bool Course::validate(Student *student)
{
for (int i = 0; i < this->array.size(); i++)
{
if (array[i] == nullptr)
{
continue;
}
if (student->studentNum == this->array[i]->studentNum)
{
return false;
}
}
return true;
}
Student *Course::getStudentByStno(int studentNum)
{
for (int i = 0; i < array.size(); i++)
{
if (studentNum == array[i]->studentNum)
{
std::wcout << L"found and returned" << std::endl;
return array[i];
}
}
std::wcout << L"not found.. returned null" << std::endl;
return nullptr;
}
std::vector<Student*> Course::getStudentByGPA(double gpa)
{
std::vector<Student*> temp(counter);
int tempo = 0;
for (int i = 0; i < array.size(); i++)
{
if (array[i] == nullptr)
{
continue;
}
if (gpa == array[i]->GPA)
{
temp[tempo] = array[i];
tempo++;
}
}
return temp;
}
double Course::findMaxGpa()
{
double max = 0;
for (int i = 0; i < array.size(); i++)
{
if (array[i] == nullptr)
{
continue;
}
if (array[i]->GPA > max)
{
max = array[i]->GPA;
}
}
return max;
}
}
{
void main::main(std::vector<std::wstring> &args)
{
Student *student1 = new Student(L"Saif", 01, L"02323123", 2.0, 3.0);
Student *student2 = new Student(L"abdulla", 02, L"1231232", 1.0, 3.0);
std::wcout << student1->isequal(student2) << std::endl;
Student *student3 = new Student(L"hazem", 01, L"02323123", 3.0, 3.0);
Student *student4 = new Student(L"khalid", 04, L"34534535", 3.0, 3.0);
std::wcout << student3->isequal(student4) << std::endl;
Course *science = new Course(L"science", 01, 3.0, 10);
science->addStudent(student1);
science->addStudent(student2);
science->addStudent(student3);
science->addStudent(student4);
std::wcout << L"student 1 gpa is: " << student1->GPA << std::endl;
student1->addGpa(5.0);
std::wcout << L"student 1 gpa is: " << student1->GPA << std::endl;
student1->addGpa(0.5);
std::wcout << L"student 1 gpa is: " << student1->GPA << std::endl;
std::wcout << L"The pointer for student 1 is : " << student1 << std::endl;
std::wcout << science->getStudentByStno(01) << std::endl;
std::vector<Student*> array2 = science->getStudentByGPA(3.0);
std::wcout << array2[0]->studentName << std::endl;
std::wcout << science->findMaxGpa() << std::endl;
Student *student5 = new Student(L"smartest", 05, L"24234234", 4.0, 3.0);
science->addStudent(student5);
std::wcout << science->findMaxGpa() << std::endl;
delete science;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

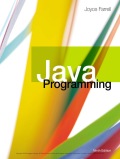
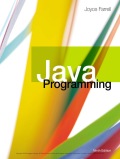